优先队列式分支限界旅行商
时间: 2024-12-27 12:18:41 浏览: 5
### 使用优先队列式分支限界法解决旅行商问题
#### 算法概述
为了高效求解旅行商问题,可以利用优先队列式的分支限界法。这种方法通过构建一棵排列树并以广度优先或最小耗费优先的方式搜索这棵树来寻找最优路径[^1]。
#### 排列树结构
对于给定的城市集合,算法会创建一颗排列树,在此树上每一个节点代表部分访问过的城市序列。叶子节点则对应完整的巡回路线。当遍历至某一节点时,计算其对应的子树成本下限(`lcost`),以此作为该节点在优先队列中的优先级[^2]。
#### 成本估算函数
设当前已经产生的费用为 `cc` ,而未被考虑在内的其余城市的最低出行开销总和记作 `rcost` 。那么任意中间状态下的估计花费就等于两者之和:\[ lcost = cc + rcost \]
#### 伪代码描述
以下是基于上述原理设计的一个简化版Python实现:
```python
import heapq
def tsp_branch_and_bound(dist_matrix, start_city):
n = len(dist_matrix)
# 初始化变量
best_cost = float('inf')
pq = [(0, [start_city], set([start_city]))]
while pq:
cost_so_far, path, visited_cities = heapq.heappop(pq)
last_visited = path[-1]
if len(path) == n and dist_matrix[last_visited][start_city]:
current_total_cost = cost_so_far + dist_matrix[last_visited][start_city]
if current_total_cost < best_cost:
best_path = path[:] + [start_city]
best_cost = current_total_cost
else:
for next_city in range(n):
if next_city not in visited_cities and dist_matrix[last_visited][next_city]:
new_cost = cost_so_far + dist_matrix[last_visited][next_city]
estimated_remaining_cost = sum(min(row) for row in (dist_matrix[i] for i in range(n) if i not in visited_cities))
lower_bound = new_cost + estimated_remaining_cost
if lower_bound < best_cost:
new_visited = visited_cities | {next_city}
new_path = path[:]
new_path.append(next_city)
heapq.heappush(pq, (new_cost, new_path, new_visited))
return best_path, best_cost
```
这段程序接收一个距离矩阵以及起始城市索引作为输入参数,并返回最佳游览顺序及其关联的成本。它使用了堆数据结构(`heapq`)模拟了一个最小化优先队列用于管理待处理的状态列表。
阅读全文
相关推荐
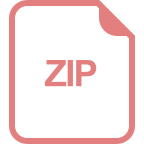
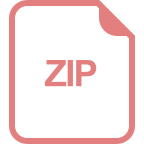
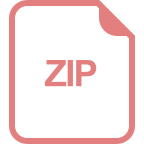
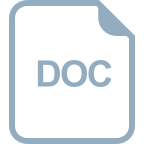














