ros moveit中点云图转环境scene的Python接口
时间: 2024-03-05 14:47:41 浏览: 149
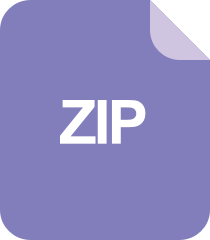
moveit_python:纯Python绑定到ROS MoveIt!

如果你是指点云图(即已经通过某种方式生成了点云图像,比如激光雷达扫描等),同样可以使用Python接口将其转换为MoveIt中的环境场景。具体的代码如下:
```python
import rospy
from sensor_msgs.msg import PointCloud2
from moveit_msgs.msg import CollisionObject
from moveit_msgs.srv import ApplyPlanningScene
from shape_msgs.msg import SolidPrimitive
from geometry_msgs.msg import Pose
import numpy as np
import cv2
def pointcloud_to_collision_object(pointcloud_msg):
# 将点云图像转换为点云数据
point_cloud = np.zeros((pointcloud_msg.height, pointcloud_msg.width, 3))
for i in range(pointcloud_msg.height * pointcloud_msg.width):
point_cloud[i // pointcloud_msg.width][i % pointcloud_msg.width] = [pointcloud_msg.data[i * 3], pointcloud_msg.data[i * 3 + 1], pointcloud_msg.data[i * 3 + 2]]
# 将点云数据转换为moveit_msgs::CollisionObject消息类型
collision_object = CollisionObject()
collision_object.header.frame_id = pointcloud_msg.header.frame_id
collision_object.id = "pointcloud"
primitive = SolidPrimitive()
primitive.type = SolidPrimitive.BOX
primitive.dimensions = [0.1, 0.1, 0.1]
pose = Pose()
pose.orientation.w = 1.0
collision_object.primitives.append(primitive)
collision_object.primitive_poses.append(pose)
return collision_object
def update_planning_scene(collision_object):
# 将CollisionObject消息发布到/planning_scene中,更新当前的环境场景
rospy.wait_for_service("/apply_planning_scene")
try:
apply_planning_scene = rospy.ServiceProxy("/apply_planning_scene", ApplyPlanningScene)
request = ApplyPlanningSceneRequest()
request.scene.is_diff = True
request.scene.world.collision_objects.append(collision_object)
response = apply_planning_scene(request)
except rospy.ServiceException as e:
rospy.logerr("Failed to update planning scene: {}".format(str(e)))
if __name__ == "__main__":
rospy.init_node("pointcloud_to_collision_object")
# 读取点云图像
pointcloud_image = cv2.imread("pointcloud_image.jpg", cv2.IMREAD_GRAYSCALE)
# 将点云图像转换为PointCloud2消息
pointcloud_msg = PointCloud2()
pointcloud_msg.header.frame_id = "map"
pointcloud_msg.height = pointcloud_image.shape[0]
pointcloud_msg.width = pointcloud_image.shape[1]
pointcloud_msg.is_dense = True
pointcloud_msg.is_bigendian = False
pointcloud_msg.fields.append(PointField(name="x", offset=0, datatype=7, count=1))
pointcloud_msg.fields.append(PointField(name="y", offset=4, datatype=7, count=1))
pointcloud_msg.fields.append(PointField(name="z", offset=8, datatype=7, count=1))
pointcloud_msg.point_step = 12
pointcloud_msg.row_step = pointcloud_image.shape[1] * pointcloud_msg.point_step
# 将点云图像转换为点云数据
point_cloud = np.zeros((pointcloud_msg.height, pointcloud_msg.width, 3))
for i in range(pointcloud_msg.height * pointcloud_msg.width):
point_cloud[i // pointcloud_msg.width][i % pointcloud_msg.width] = [i % pointcloud_msg.width, i // pointcloud_msg.width, pointcloud_image[i // pointcloud_msg.width][i % pointcloud_msg.width]]
pointcloud_msg.data = point_cloud.astype(np.float32).tostring()
# 将PointCloud2消息转换为CollisionObject消息
collision_object = pointcloud_to_collision_object(pointcloud_msg)
# 发布CollisionObject消息到/planning_scene中,更新当前的环境场景
update_planning_scene(collision_object)
rospy.spin()
```
在上述代码中,首先读取点云图像,并将其转换为PointCloud2消息。然后将PointCloud2消息转换为点云数据,并将其转换为moveit_msgs::CollisionObject消息类型。最后将CollisionObject消息发布到/planning_scene中,更新当前的环境场景。
阅读全文
相关推荐
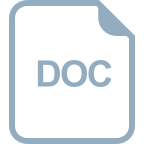
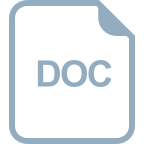

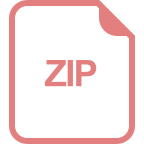



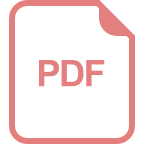
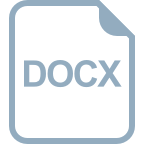
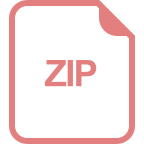
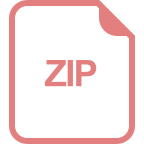
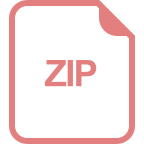
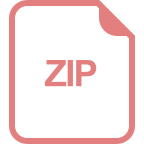
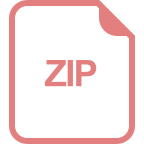
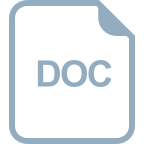


