stm32f407光电小车以坐标行进的代码
时间: 2023-07-09 19:49:30 浏览: 38
首先,需要明确小车的坐标系以及如何进行坐标转换。假设小车当前所在位置为(x,y),朝向角度为theta,前进距离为d,则小车前进后的新位置可以通过以下公式计算:
new_x = x + d * cos(theta)
new_y = y + d * sin(theta)
接下来是具体的代码实现:
```c
#include "stm32f4xx.h"
#include "math.h"
#define PI 3.14159265358979323846
// 小车当前位置和朝向角度
float x = 0.0;
float y = 0.0;
float theta = 0.0;
// 前进距离和速度
float distance = 0.0;
float speed = 0.0;
// 转弯半径和速度
float radius = 0.0;
float turn_speed = 0.0;
// 计算角度对应的弧度值
float deg2rad(float deg) {
return deg / 180.0 * PI;
}
// 前进一定距离
void move_forward(float distance) {
float dx = distance * cos(deg2rad(theta));
float dy = distance * sin(deg2rad(theta));
x += dx;
y += dy;
}
// 左转一定角度
void turn_left(float angle) {
float dtheta = deg2rad(angle);
float dx = radius * (sin(theta + dtheta) - sin(theta));
float dy = radius * (-cos(theta + dtheta) + cos(theta));
x += dx;
y += dy;
theta += angle;
}
// 右转一定角度
void turn_right(float angle) {
turn_left(-angle);
}
// 初始化
void init() {
// TODO: 初始化GPIO和PWM等
}
int main(void) {
init();
while(1) {
// TODO: 读取光电传感器数据,决定前进距离和速度
if(distance > 0.0) {
move_forward(distance);
}
// TODO: 根据光电传感器数据,决定转弯半径和速度
if(radius > 0.0 && turn_speed > 0.0) {
float arc_length = radius * deg2rad(90.0);
float arc_time = arc_length / turn_speed;
// 左转90度
turn_left(90.0);
// 等待转弯完成
HAL_Delay((uint32_t)(arc_time * 1000));
}
}
}
```
需要注意的是,上述代码仅提供了基本的坐标行进功能,具体实现还需要根据硬件和实际需求进行调整。
相关推荐
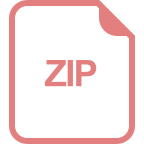
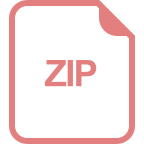














