改写“def truncated_svd(matrix,percent):#percent表示选择的特征值个数占所有特征值个数的比例 #进行奇异值分解 U,sigma,V_T=np.linalg.svd(matrix) m=U.shape[0] n=V_T.shape[0] w=np.zeros((m,n)) for k in range(len(sigma)): #以得到该通道的近似数据矩阵 #逐个累加 w=w+sigma[k]*np.dot(U[:,k].reshape(m,1),V_T[k,:].reshape(1,n)) #如果已经超过设定的比例阈值 if(float(k)/len(sigma)>percent): #将数据值规范到0-255范围内 w[w<0]=0 w[w>255]=255 break #为返回的近似数据矩阵的元素数据类型规范 #为uint8 return np.rint(w).astype("uint8")”
时间: 2023-06-20 14:06:02 浏览: 124
可以改写为:
```python
def truncated_svd(matrix, percent):
# 使用奇异值分解进行矩阵分解
U, sigma, V_T = np.linalg.svd(matrix)
# 计算特征值个数
num_features = len(sigma)
# 根据所选特征值占比计算要保留的特征值个数
num_keep = int(num_features * percent)
# 计算近似矩阵
w = np.dot(U[:, :num_keep], np.dot(np.diag(sigma[:num_keep]), V_T[:num_keep, :]))
# 将数据值规范到0-255范围内
w[w < 0] = 0
w[w > 255] = 255
# 将数据类型转换为uint8
return w.astype("uint8")
```
这个改写版本的思路是先计算所需要保留的特征值个数,再根据这个特征值个数计算近似矩阵,最后将数据值规范到0-255范围内并将数据类型转换为uint8。这样可以避免循环计算,提高运行效率。
相关问题
改写“def RE(U,sigma,V_T,percent):#percent表示选择的特征值个数占所有特征值个数的比例 m=U.shape[0] n=V_T.shape[0] w=np.zeros((m,n)) for k in range(len(sigma)): #以得到该通道的近似数据矩阵 #逐个累加 w=w+sigma[k]*np.dot(U[:,k].reshape(m,1),V_T[k,:].reshape(1”
这段代码的作用是对一个矩阵进行奇异值分解,并根据给定的比例选择特征值,生成一个近似的矩阵。
以下是改写后的代码:
```
def truncated_svd(matrix, percent):
# 进行奇异值分解
U, sigma, V_T = np.linalg.svd(matrix)
# 计算需要保留的特征值个数
num_sv = int(percent * len(sigma))
# 生成近似的数据矩阵
approx_matrix = np.zeros(matrix.shape)
for k in range(num_sv):
approx_matrix += sigma[k] * np.outer(U[:, k], V_T[k, :])
return approx_matrix
```
改写后的代码将原来的函数名 `RE` 改为了 `truncated_svd`,更好地反映了函数的作用。此外,为了代码的可读性,将原来的变量名 `w` 改为了 `approx_matrix`,更好地表达了变量的含义。
obj/single_step.o: in function `single_step_': single_step.f90:(.text+0x13): relocation truncated to fit: R_X86_64_PC32 against `.bss' single_step.f90:(.text+0x56): relocation truncated to fit: R_X86_64_PC32 against `.bss' single_step.f90:(.text+0x62): relocation truncated to fit: R_X86_64_PC32 against `.bss' single_step.f90:(.text+0x7a): relocation truncated to fit: R_X86_64_PC32 against `.bss' single_step.f90:(.text+0x81): relocation truncated to fit: R_X86_64_PC32 against `.bss' single_step.f90:(.text+0x140): relocation truncated to fit: R_X86_64_PC32 against `.bss' single_step.f90:(.text+0x152): relocation truncated to fit: R_X86_64_PC32 against `.bss' single_step.f90:(.text+0x15d): relocation truncated to fit: R_X86_64_PC32 against `.bss' single_step.f90:(.text+0x18e): relocation truncated to fit: R_X86_64_PC32 against `.bss' single_step.f90:(.text+0x1d4): relocation truncated to fit: R_X86_64_PC32 against `.bss' single_step.f90:(.text+0x26a): additional relocation overflows omitted from the output collect2: error: ld returned 1 exit status make: *** [makefile:40: plasma] Error 1
这个错误是由于链接器(ld)在链接时发现某些符号引用无法适应可执行文件的地址空间而导致的。具体来说,这些错误信息提示了一些符号(例如`.bss`)的重定位超出了限制。
要解决这个问题,你可以尝试以下几种方法:
1. 检查你的代码中是否存在一些过大的数组或变量,它们可能会导致符号引用超出限制。如果有的话,你可以尝试减小数组或变量的大小,或者将它们分配到堆上而不是栈上。
2. 检查你的编译选项是否正确设置。你可以尝试添加`-mcmodel=medium`或`-mcmodel=large`选项来扩大地址空间。
3. 如果你正在使用静态库或共享库,确保这些库是针对相同的架构和地址空间编译的。如果不是,你可能需要重新编译这些库以匹配你的目标架构和地址空间。
4. 检查你的链接脚本(如果有的话)是否正确配置了地址空间。
希望以上方法能帮助你解决问题!如果还有其他疑问,请随时提问。
阅读全文
相关推荐
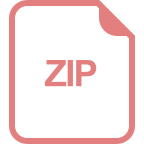
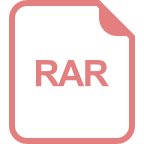














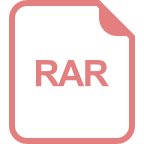