KLD粒子滤波方法代码实现
时间: 2023-11-02 13:19:08 浏览: 179
KLD粒子滤波(KLD Particle Filter)是一种基于粒子滤波(Particle Filter)的自适应重要性采样算法,其目的是在不知道系统噪声分布的情况下,自适应调整粒子数,以达到比较好的估计效果。以下是KLD粒子滤波方法的代码实现:
```python
import numpy as np
import math
class KLDParticleFilter:
def __init__(self, initial_state, num_particles, motion_model, measurement_model, resampling_threshold=0.5, kld_err=0.01, kld_z=0.99):
self.num_particles = num_particles
self.motion_model = motion_model
self.measurement_model = measurement_model
self.resampling_threshold = resampling_threshold
self.kld_err = kld_err
self.kld_z = kld_z
self.particles = np.tile(initial_state, (num_particles, 1)) + np.random.randn(num_particles, len(initial_state)) * 0.1
self.weights = np.ones(num_particles) / num_particles
self.resample_count = 0
def predict(self, control_input):
self.particles = self.motion_model(self.particles, control_input) + np.random.randn(self.num_particles, len(control_input)) * 0.1
def update(self, measurement):
likelihood = self.measurement_model(measurement, self.particles)
self.weights *= likelihood
self.weights /= np.sum(self.weights)
# check if the effective sample size is below the resampling threshold
ess = 1 / np.sum(np.square(self.weights))
if ess < self.resampling_threshold * self.num_particles:
self.resample()
def resample(self):
# calculate the Kullback-Leibler divergence between the current and the target distributions
kl_div = self.kld(self.weights)
# compute the new number of particles
n = int(math.ceil(self.kld_z / self.kld_err ** 2 * kl_div))
# resample particles
indices = self.stratified_resample(self.weights)
self.particles = self.particles[indices]
self.weights = np.ones(self.num_particles) / self.num_particles
self.resample_count += 1
def kld(self, weights):
# compute the Kullback-Leibler divergence between the current and the uniform distribution
return np.sum(weights * np.log(weights * self.num_particles))
def stratified_resample(self, weights):
indices = np.zeros(self.num_particles, dtype=np.int32)
cum_weights = np.cumsum(weights)
step = 1 / self.num_particles
u = np.random.uniform(0, step)
idx = 0
for i in range(self.num_particles):
while u > cum_weights[idx]:
idx += 1
indices[i] = idx
u += step
return indices
```
其中,`initial_state`是粒子的初始状态,`num_particles`是粒子的数量,`motion_model`是运动模型,`measurement_model`是测量模型,`resampling_threshold`是重采样阈值,`kld_err`和`kld_z`是KLD粒子滤波算法中的参数。
`predict`方法根据控制量,对粒子进行运动模型的预测;`update`方法利用测量值更新粒子的权重,并判断是否需要进行重采样;`resample`方法实现了KLD粒子滤波算法中的自适应重采样;`kld`方法计算粒子权重分布与均匀分布的Kullback-Leibler散度;`stratified_resample`方法实现了分层重采样。
阅读全文
相关推荐
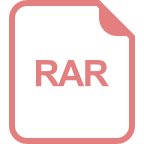
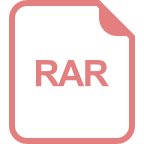
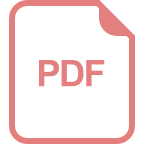















