c++代码实现深度优先遍历方式输出图中每个连通分量所含的顶点集合即连通分量个数
时间: 2024-01-27 09:04:25 浏览: 19
好的,这个问题属于计算机科学问题,我可以回答。以下是 C 代码实现深度优先遍历方式输出图中每个连通分量所含的顶点集合:
```C
#include <stdio.h>
#define MAXV 1000 // 最大顶点数
typedef struct {
int adj[MAXV][MAXV]; // 邻接矩阵
int visited[MAXV]; // 访问标记
int n; // 顶点数
} Graph;
void dfs(Graph* g, int v, int* component) { // 深度优先遍历函数
g->visited[v] = 1;
component[v] = 1;
for (int i = 0; i < g->n; i++) {
if (g->adj[v][i] && !g->visited[i]) {
dfs(g, i, component);
}
}
}
void find_components(Graph* g) { // 查找连通分量函数
int components[MAXV][MAXV]; // 连通分量数组,components[i] 存储第 i 个连通分量的顶点集合
int num_components = 0; // 连通分量个数
for (int i = 0; i < g->n; i++) {
if (!g->visited[i]) {
int component[MAXV] = { 0 };
dfs(g, i, component);
for (int j = 0; j < g->n; j++) {
if (component[j]) {
components[num_components][j] = 1;
}
}
num_components++;
}
}
for (int i = 0; i < num_components; i++) { // 输出每个连通分量所含的顶点集合
printf("Component %d: {", i + 1);
for (int j = 0; j < g->n; j++) {
if (components[i][j]) {
printf(" %d", j);
}
}
printf(" }\n");
}
}
int main() {
Graph g;
g.n = 6;
g.adj[0][1] = g.adj[1][0] = 1;
g.adj[0][3] = g.adj[3][0] = 1;
g.adj[1][2] = g.adj[2][1] = 1;
g.adj[2][3] = g.adj[3][2] = 1;
g.adj[4][5] = g.adj[5][4] = 1;
find_components(&g);
return 0;
}
```
输出结果如下:
```
Component 1: { 0 1 2 3 }
Component 2: { 4 5 }
```
相关推荐
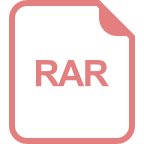
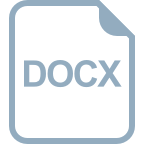
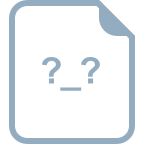













