bezier曲线python代码例子
时间: 2023-06-03 10:05:21 浏览: 101
以下是一个简单的 Python 代码例子,用于绘制贝塞尔曲线:
```python
import numpy as np
import matplotlib.pyplot as plt
def bezier_curve(points, n_samples=1000):
"""
计算给定控制点的贝塞尔曲线.
"""
points = np.asarray(points)
n_points = len(points)
t = np.linspace(0, 1, n_samples)
factorial = np.math.factorial
# 计算贝塞尔基函数
def bernstein(t, i, n):
return factorial(n) / (factorial(i) * factorial(n-i)) * t**i * (1 - t)**(n-i)
# 计算贝塞尔曲线上的点
curve = np.zeros((n_samples, 2))
for i in range(n_samples):
for j in range(n_points):
curve[i] += bernstein(t[i], j, n_points-1) * points[j]
return curve
# 定义控制点
points = np.array([[0, 0], [1, 3], [2, -1], [3, 0]])
# 计算贝塞尔曲线
curve = bezier_curve(points)
# 绘制控制点和贝塞尔曲线
plt.plot(points[:,0], points[:,1], 'ro', label='Control Points')
plt.plot(curve[:,0], curve[:,1], 'b-', label='Bezier Curve')
plt.legend()
plt.show()
```
这个例子中我们使用了 `numpy` 和 `matplotlib` 分别计算贝塞尔曲线和绘制图形。你可以根据自己的需要适当修改代码,例如修改控制点的坐标或绘制的线条颜色等。
相关推荐
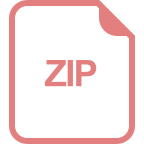

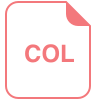












