KLD粒子滤波方法代码实现
时间: 2023-11-02 10:08:39 浏览: 123
KLD粒子滤波 (KLD Particle Filter) 是一种高效的粒子滤波算法,它通过自适应地增加或删除粒子来提高粒子的重要性采样效率。下面是KLD粒子滤波的代码实现:
```python
import numpy as np
class KLDParticleFilter:
def __init__(self, num_particles, motion_model, measurement_model, resampling_threshold=0.5):
self.num_particles = num_particles
self.motion_model = motion_model
self.measurement_model = measurement_model
self.resampling_threshold = resampling_threshold
self.particles = None
self.weights = None
def initialize_particles(self, initial_state):
self.particles = np.random.normal(loc=initial_state, scale=0.1, size=(self.num_particles, len(initial_state)))
self.weights = np.ones(self.num_particles) / self.num_particles
def predict(self, control_input):
self.particles = self.motion_model(self.particles, control_input)
def update(self, observation):
likelihoods = self.measurement_model(observation, self.particles)
self.weights *= likelihoods
self.weights /= np.sum(self.weights)
n_eff = 1 / np.sum(np.square(self.weights))
if n_eff < self.resampling_threshold * self.num_particles:
self.resample()
def resample(self):
indices = np.random.choice(self.num_particles, size=self.num_particles, replace=True, p=self.weights)
self.particles = self.particles[indices]
self.weights = np.ones(self.num_particles) / self.num_particles
def estimate(self):
return np.average(self.particles, axis=0, weights=self.weights)
```
在这个实现中,我们首先定义了一个 `KLDParticleFilter` 类,它包含了粒子滤波的所有步骤。在初始化时,我们需要指定粒子的数量、运动模型、测量模型和重采样阈值。在 `initialize_particles` 方法中,我们根据初始状态生成一些随机粒子,并将它们的权重初始化为相等值。在 `predict` 方法中,我们使用运动模型对粒子进行预测。在 `update` 方法中,我们使用测量模型对粒子的权重进行更新,并检查有效粒子数是否小于重采样阈值。如果是,我们就进行重采样。最后,在 `estimate` 方法中,我们使用加权平均估计当前状态。
需要注意的是,这个实现只是一个简单的示例,实际使用中需要根据具体情况进行调整。
阅读全文
相关推荐
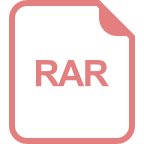
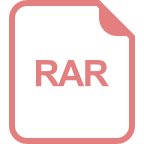
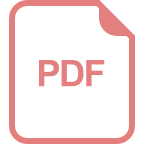














