c语言 五子棋代码 用队列存储每一步棋
时间: 2024-01-18 12:02:21 浏览: 64
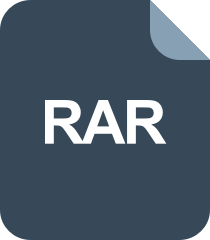
Five.rar(C语言版)
好的,下面是一个简单的 C 语言五子棋代码,使用队列存储每一步棋:
```c
#include <stdio.h>
#include <stdlib.h>
#define BOARD_SIZE 15
// 定义队列节点
typedef struct Node {
int x;
int y;
struct Node* next;
} Node;
// 定义队列
typedef struct Queue {
Node* front;
Node* rear;
} Queue;
// 初始化队列
void initQueue(Queue* q) {
q->front = q->rear = NULL;
}
// 入队
void enQueue(Queue* q, int x, int y) {
Node* node = (Node*)malloc(sizeof(Node));
node->x = x;
node->y = y;
node->next = NULL;
if (q->rear == NULL) {
q->front = q->rear = node;
} else {
q->rear->next = node;
q->rear = node;
}
}
// 出队
void deQueue(Queue* q) {
if (q->front == NULL) return;
Node* node = q->front;
q->front = q->front->next;
if (q->front == NULL) {
q->rear = NULL;
}
free(node);
}
// 判断胜利
int checkWin(int board[][BOARD_SIZE], int x, int y) {
int i, j, k;
int count = 1;
// 判断横向
for (i = y - 1; i >= 0 && board[x][i] == board[x][y]; i--, count++);
for (i = y + 1; i < BOARD_SIZE && board[x][i] == board[x][y]; i++, count++);
if (count >= 5) return 1;
// 判断纵向
count = 1;
for (i = x - 1; i >= 0 && board[i][y] == board[x][y]; i--, count++);
for (i = x + 1; i < BOARD_SIZE && board[i][y] == board[x][y]; i++, count++);
if (count >= 5) return 1;
// 判断右上斜向
count = 1;
for (i = x - 1, j = y + 1; i >= 0 && j < BOARD_SIZE && board[i][j] == board[x][y]; i--, j++, count++);
for (i = x + 1, j = y - 1; i < BOARD_SIZE && j >= 0 && board[i][j] == board[x][y]; i++, j--, count++);
if (count >= 5) return 1;
// 判断右下斜向
count = 1;
for (i = x - 1, j = y - 1; i >= 0 && j >= 0 && board[i][j] == board[x][y]; i--, j--, count++);
for (i = x + 1, j = y + 1; i < BOARD_SIZE && j < BOARD_SIZE && board[i][j] == board[x][y]; i++, j++, count++);
if (count >= 5) return 1;
return 0;
}
// 打印棋盘
void printBoard(int board[][BOARD_SIZE]) {
int i, j;
for (i = 0; i < BOARD_SIZE; i++) {
for (j = 0; j < BOARD_SIZE; j++) {
if (board[i][j] == 1) {
printf("● ");
} else if (board[i][j] == 2) {
printf("○ ");
} else {
printf("+ ");
}
}
printf("\n");
}
}
int main() {
int board[BOARD_SIZE][BOARD_SIZE] = {0};
int player = 1;
Queue steps;
initQueue(&steps);
while (1) {
int x, y;
printf("玩家 %d,请输入您要落子的位置(x y):", player);
scanf("%d%d", &x, &y);
if (x < 0 || x >= BOARD_SIZE || y < 0 || y >= BOARD_SIZE) {
printf("输入位置不合法,请重新输入!\n");
continue;
}
if (board[x][y] != 0) {
printf("该位置已经有棋子,请重新输入!\n");
continue;
}
board[x][y] = player;
enQueue(&steps, x, y);
printBoard(board);
if (checkWin(board, x, y)) {
printf("玩家 %d 赢了!\n", player);
break;
}
player = player == 1 ? 2 : 1;
}
// 打印每一步棋
Node* node = steps.front;
while (node) {
printf("(%d,%d) ", node->x, node->y);
node = node->next;
}
printf("\n");
return 0;
}
```
在这个代码中,我们定义了一个 `Node` 结构体作为队列的节点,其中包含了横纵坐标信息。然后定义了一个 `Queue` 结构体作为队列,其中包含了队列的头和尾指针。接着定义了一些队列操作函数,包括初始化队列、入队、出队。最后定义了一个 `checkWin` 函数用来判断是否胜利,一个 `printBoard` 函数用来打印棋盘。
在 `main` 函数中,我们使用一个 `while` 循环来让玩家交替下棋。每次玩家输入横纵坐标后,我们先判断输入位置是否合法,如果不合法就让玩家重新输入。然后判断该位置是否已经有棋子,如果有就让玩家重新输入。接着把该位置上的棋子放到棋盘上,并把该节点入队到 `steps` 队列中。然后打印棋盘,判断是否胜利。如果胜利就输出胜利信息,结束游戏。
最后,我们可以遍历 `steps` 队列,输出每一步棋的横纵坐标。
阅读全文
相关推荐
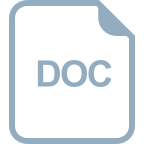
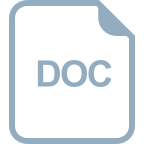


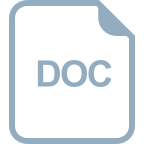
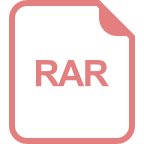
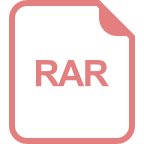
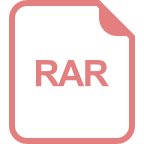
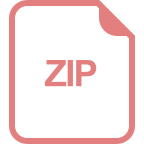
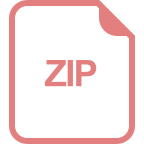
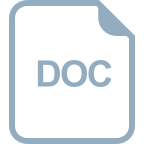
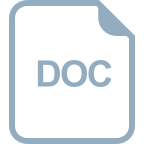
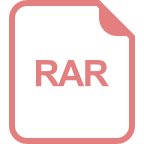