对偶单纯形算法的代码实现
时间: 2024-06-02 18:05:01 浏览: 22
对偶单纯形算法是线性规划中的一种常见算法,其主要思想是不断通过单纯形操作来改变对偶问题的解以逼近原问题的最优解。对偶单纯形算法的代码实现需要注意以下几点:
1. 初始时需要构造对偶问题,并将对偶问题转化为标准型。
2. 每次迭代时需要选取一个入基变量和一个出基变量。
3. 对偶单纯形算法需要保证对偶问题的可行性,因此要在每次迭代之前检验对偶问题的可行性。
4. 对于无界问题和不可行问题需要进行特殊处理。
下面是对偶单纯形算法的代码实现(Python):
```python
import numpy as np
def dual_simplex(c, A, b):
# 构造对偶问题
m, n = A.shape
c_d = -b
A_d = -A.T
b_d = c
# 将对偶问题转化为标准型
c_d = np.hstack((c_d, np.zeros(m)))
A_d = np.hstack((A_d, np.eye(m)))
basic_idx = range(n, n+m)
while True:
# 计算对偶问题的解
x_d = np.zeros(n+m)
x_d[basic_idx] = np.linalg.solve(A_d[:, basic_idx], b_d)
u = c_d + A_d.T @ x_d
# 检验对偶问题的可行性
if np.all(u >= 0):
x = np.zeros(n)
x[basic_idx[:n]] = x_d[basic_idx[:n]]
return x, -c @ x
# 选取入基变量和出基变量
j0 = np.argmin(u)
if u[j0] >= 0:
return None, -np.inf
d = np.linalg.solve(A_d[:, basic_idx], A_d[:, j0])
if np.all(d <= 0):
return None, np.inf
ratios = x_d[basic_idx] / d
ratios[d <= 0] = np.inf
i0 = basic_idx[np.argmin(ratios)]
# 更新基
basic_idx[basic_idx == i0] = j0
if __name__ == '__main__':
c = np.array([2, 1, 0, 0])
A = np.array([
[1, 2, 1, 0],
[1, 1, 0, 1]
])
b = np.array([4, 3])
x, z = dual_simplex(c, A, b)
print(x, z)
```
相关推荐
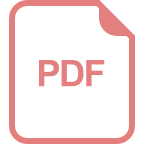
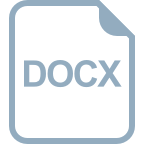
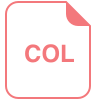













