error C2352: CTestView::SetLight : illegal call of non-static member function
时间: 2023-12-21 09:06:39 浏览: 33
这个错误通常发生在尝试在非静态成员函数中访问非静态成员变量时。如果您想在非静态成员函数中访问非静态成员变量,必须先创建该类的对象并使用该对象来调用该函数。
例如:
```cpp
class CTestView {
public:
void SetLight(int nLight) { m_nLight = nLight; }
private:
int m_nLight;
};
int main() {
CTestView view;
view.SetLight(1);
return 0;
}
```
如果您需要在静态成员函数中访问非静态成员变量,则必须先创建该类的对象并使用该对象来访问该变量。
例如:
```cpp
class CTestView {
public:
static void SetLight(int nLight) {
CTestView view;
view.m_nLight = nLight;
}
private:
int m_nLight;
};
int main() {
CTestView::SetLight(1);
return 0;
}
```
请注意,这里我们创建了一个新的CTestView对象来访问m_nLight变量。
相关问题
void CTestView::DrawPolygon(CDC *pDC,CLine *line)//绘制立方体线框模型 { for(int nFace=0;nFace<6;nFace++) { CP2 t; for(int nVertex=0;nVertex<F[nFace].vN;nVertex++)//顶点循环 { ObliqueProject(P[F[nFace].vI[nVertex]]);//斜等测投影 if(0==nVertex) { line->MoveTo(pDC,ScreenP.x,ScreenP.y); t=ScreenP; } else line->LineTo(pDC,ScreenP.x,ScreenP.y); } line->LineTo(pDC,t.x,t.y);//闭合多边形 } }改为三棱锥
void CTestView::DrawPyramid(CDC *pDC, CLine *line)//绘制三棱锥线框模型
{
for(int nFace = 0; nFace < 4; nFace++)
{
CP2 t;
for(int nVertex = 0; nVertex < P[nFace].vN; nVertex++)
{
ObliqueProject(P[nFace].vI[nVertex]);//斜等测投影
if(0 == nVertex)
{
line->MoveTo(pDC, ScreenP.x, ScreenP.y);
t = ScreenP;
}
else
{
line->LineTo(pDC, ScreenP.x, ScreenP.y);
}
}
line->LineTo(pDC, t.x, t.y);//闭合多边形
}
for(int nVertex = 0; nVertex < P[4].vN; nVertex++)//底面的顶点循环
{
ObliqueProject(P[4].vI[nVertex]);//斜等测投影
if(0 == nVertex)
{
line->MoveTo(pDC, ScreenP.x, ScreenP.y);
}
else
{
line->LineTo(pDC, ScreenP.x, ScreenP.y);
}
}
}
void CTestView::ReadPoint()//点表 { double a=100;//立方体边长为a //顶点的三维坐标(x,y,z) P[0].x=0;P[0].y=0;P[0].z=0; P[1].x=a;P[1].y=0;P[1].z=0; P[2].x=a;P[2].y=a;P[2].z=0; P[3].x=0;P[3].y=a;P[3].z=0; P[4].x=0;P[4].y=0;P[4].z=a;改为三棱锥
好的,那么你想要将这个立方体改为三棱锥,我可以帮你修改代码。三棱锥有五个顶点,其中一个为顶点,其他四个为底面的四个点。假设三棱锥的高为h,那么可以将代码修改如下:
```
void CTestView::ReadPoint()//点表
{
double a=100;//底面边长为a,也可以自己定义
double h=150;//三棱锥的高
//顶点的三维坐标(x,y,z)
P[0].x=a/2;P[0].y=a/2;P[0].z=h; //顶点
P[1].x=0;P[1].y=0;P[1].z=0; //底面第一个点
P[2].x=a;P[2].y=0;P[2].z=0; //底面第二个点
P[3].x=a;P[3].y=a;P[3].z=0; //底面第三个点
P[4].x=0;P[4].y=a;P[4].z=0; //底面第四个点
}
```
这样,立方体的代码就被修改成了三棱锥的代码。注意,这里假设三棱锥的底面为正三角形。如果底面是其它形状,需要根据实际情况进行修改。
相关推荐
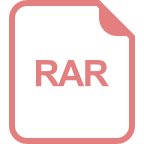
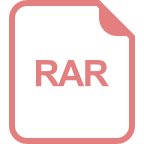
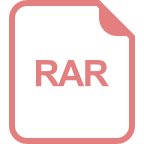








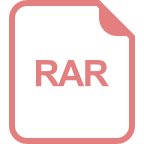
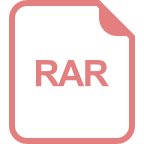
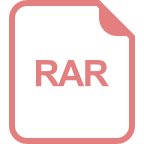
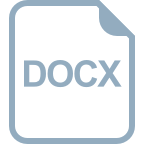