利用最速下降法求解多目标优化算法测试函数python绘制及相关代码
时间: 2023-06-11 14:08:16 浏览: 119
多目标优化算法中,最速下降法是一种常用的优化方法。下面提供一个利用Python绘制多目标优化算法测试函数图像的示例代码,其中包含了最速下降法的实现。
首先,需要导入相关的库:
```python
import numpy as np
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
```
接着,定义多目标测试函数。这里我们以ZDT1函数为例:
```python
def ZDT1(x):
f1 = x[0]
g = 1 + 9 / (len(x) - 1) * np.sum(x[1:])
f2 = g * (1 - np.sqrt(f1 / g))
return np.array([f1, f2])
```
然后,实现最速下降法:
```python
def gradient_descent(f, x0, alpha=0.01, eps=1e-6, max_iter=1000):
x = x0
iter = 0
while iter < max_iter:
fx = f(x)
grad = np.zeros_like(x)
for i in range(len(x)):
delta = np.zeros_like(x)
delta[i] = eps
grad[i] = (f(x + delta) - fx) / eps
x = x - alpha * grad
if np.linalg.norm(grad) < eps:
break
iter += 1
return x
```
最后,利用以上两个函数绘制测试函数的图像:
```python
def plot_ZDT1():
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
X = np.arange(0, 1, 0.01)
Y = np.arange(0, 1, 0.01)
X, Y = np.meshgrid(X, Y)
Z = np.zeros_like(X)
for i in range(X.shape[0]):
for j in range(X.shape[1]):
Z[i][j] = ZDT1([X[i][j], Y[i][j]])[0]
ax.plot_surface(X, Y, Z, cmap='rainbow', alpha=0.5)
ax.set_xlabel('x1')
ax.set_ylabel('x2')
ax.set_zlabel('f1')
ax.view_init(elev=30, azim=220)
x0 = np.array([0.1, 0.1])
alpha = 0.005
eps = 1e-6
max_iter = 1000
xs = gradient_descent(ZDT1, x0, alpha=alpha, eps=eps, max_iter=max_iter)
ax.scatter(xs[0], xs[1], ZDT1(xs)[0], color='r', s=100, marker='o')
plt.show()
```
调用plot_ZDT1函数即可绘制出ZDT1函数的图像,并在图中标记出最速下降法搜索到的最优解。
阅读全文
相关推荐
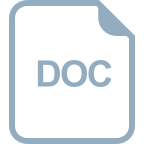
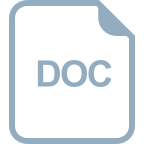
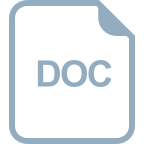

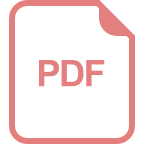
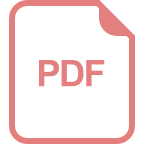
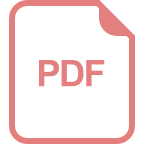









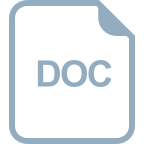
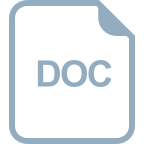
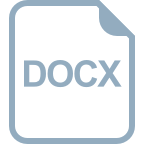