public static void shuijishu(){ int numRandomNumbers = 3000; Random random = new Random(); BigInteger[] randomNumbers = new BigInteger[numRandomNumbers]; // Generate random numbers with BigInteger for (int i = 0; i < numRandomNumbers; i++) { randomNumbers[i] = BigInteger.probablePrime(50, random); // Generate a 50-bit prime number with high probability } // Calculate the product of the random numbers BigInteger product = BigInteger.ONE; for (BigInteger number : randomNumbers) { product = product.multiply(number); } // Calculate the geometric mean of the random numbers double geometricMean = Math.pow(product.doubleValue(), 1.0 / numRandomNumbers); System.out.println("The geometric mean of the random numbers is: " + geometricMean); } 这段代码最终计算结果显示为: Infinity 有什么整改建议吗
时间: 2024-02-14 14:23:28 浏览: 18
这段代码的问题在于,在计算几何平均数时,使用了Math.pow(double a, double b)方法,该方法在a为无穷大或负数时,返回的结果为Infinity。而在这段代码中,product.doubleValue()的值可能为无穷大,因此计算结果为Infinity。
为了避免这个问题,可以使用BigDecimal类来进行精确的数值计算,而不是使用double类型。另外,由于这段代码生成的随机数是素数,因此它们的乘积可能会非常大,超出了double类型的范围,因此使用BigDecimal也可以避免这个问题。
以下是使用BigDecimal进行计算的示例代码:
public static void shuijishu() {
int numRandomNumbers = 3000;
Random random = new Random();
BigInteger[] randomNumbers = new BigInteger[numRandomNumbers];
// Generate random numbers with BigInteger
for (int i = 0; i < numRandomNumbers; i++) {
randomNumbers[i] = BigInteger.probablePrime(50, random); // Generate a 50-bit prime number with high probability
}
// Calculate the product of the random numbers
BigDecimal product = BigDecimal.ONE;
for (BigInteger number : randomNumbers) {
product = product.multiply(new BigDecimal(number));
}
// Calculate the geometric mean of the random numbers
BigDecimal geometricMean = BigDecimalMath.pow(product, BigDecimal.valueOf(1.0 / numRandomNumbers));
System.out.println("The geometric mean of the random numbers is: " + geometricMean);
}
在这个示例代码中,我们使用了BigDecimalMath类来计算幂,因为BigDecimal没有提供计算幂的方法。另外,为了将double类型的指数转换为BigDecimal类型,我们使用了BigDecimal.valueOf方法。
相关推荐
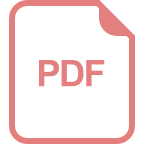
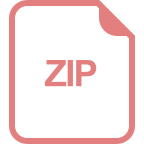














