modbus rtu代码示例
时间: 2023-08-25 12:07:35 浏览: 33
对于modbus RTU通信协议的代码示例,我们可以以C语言为例,使用串口通信库来实现。
以下是一个简单的modbus RTU通信示例,其中包括了从机和主机的代码:
从机代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <fcntl.h>
#include <termios.h>
#define BAUDRATE B9600
#define DEVICE "/dev/ttyUSB0"
#define SLAVEADDR 1
int main(int argc, char **argv) {
int fd, n;
struct termios oldtio, newtio;
unsigned char buf[256];
unsigned char response[256];
fd = open(DEVICE, O_RDWR | O_NOCTTY);
if (fd < 0) {
perror(DEVICE);
exit(-1);
}
tcgetattr(fd, &oldtio);
bzero(&newtio, sizeof(newtio));
newtio.c_cflag = BAUDRATE | CS8 | CLOCAL | CREAD;
newtio.c_iflag = IGNPAR | ICRNL;
newtio.c_oflag = 0;
newtio.c_lflag = ICANON;
tcflush(fd, TCIFLUSH);
tcsetattr(fd,TCSANOW,&newtio);
while (1) {
bzero(buf, sizeof(buf));
n = read(fd, buf, sizeof(buf));
if (n > 0 && buf[0] == SLAVEADDR && buf[1] == 0x03) {
int addr = (buf[2] << 8) | buf[3];
int count = (buf[4] << 8) | buf[5];
bzero(response, sizeof(response));
response[0] = SLAVEADDR;
response[1] = 0x03;
response[2] = count * 2;
for (int i = 0; i < count; i++) {
int value = i + addr;
response[3 + i * 2] = (value >> 8) & 0xff;
response[4 + i * 2] = value & 0xff;
}
write(fd, response, 3 + count * 2);
}
}
tcsetattr(fd,TCSANOW,&oldtio);
close(fd);
return 0;
}
```
主机代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <fcntl.h>
#include <termios.h>
#define BAUDRATE B9600
#define DEVICE "/dev/ttyUSB0"
#define SLAVEADDR 1
int main(int argc, char **argv) {
int fd, n;
struct termios oldtio, newtio;
unsigned char buf[256];
unsigned char request[256];
fd = open(DEVICE, O_RDWR | O_NOCTTY);
if (fd < 0) {
perror(DEVICE);
exit(-1);
}
tcgetattr(fd, &oldtio);
bzero(&newtio, sizeof(newtio));
newtio.c_cflag = BAUDRATE | CS8 | CLOCAL | CREAD;
newtio.c_iflag = IGNPAR | ICRNL;
newtio.c_oflag = 0;
newtio.c_lflag = ICANON;
tcflush(fd, TCIFLUSH);
tcsetattr(fd,TCSANOW,&newtio);
int startAddr = 0;
int count = 10;
bzero(request, sizeof(request));
request[0] = SLAVEADDR;
request[1] = 0x03;
request[2] = (startAddr >> 8) & 0xff;
request[3] = startAddr & 0xff;
request[4] = (count >> 8) & 0xff;
request[5] = count & 0xff;
int crc = 0xFFFF;
for (int i = 0; i < 6; i++) {
crc ^= request[i];
for (int j = 0; j < 8; j++) {
if (crc & 0x0001) {
crc >>= 1;
crc ^= 0xA001;
} else {
crc >>= 1;
}
}
}
request[6] = crc & 0xff;
request[7] = (crc >> 8) & 0xff;
write(fd, request, 8);
bzero(buf, sizeof(buf));
n = read(fd, buf, sizeof(buf));
if (n > 0 && buf[0] == SLAVEADDR && buf[1] == 0x03) {
int count = buf[2] / 2;
for (int i = 0; i < count; i++) {
int value = (buf[3 + i * 2] << 8) | buf[4 + i * 2];
printf("Address: %d, Value: %d\n", startAddr + i, value);
}
}
tcsetattr(fd,TCSANOW,&oldtio);
close(fd);
return 0;
}
```
在这个示例中,从机接收主机的读请求,并返回对应地址的数据。主机发送读请求,并解析从机返回的数据。示例中使用了一个简单的CRC16校验,不过实际使用中还需要根据协议的要求进行校验。
相关推荐
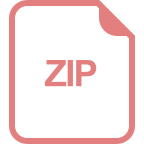














