帮我用pytorch写一段我个输入两个隐藏层2个输出的神经网络程序
时间: 2024-05-10 22:21:28 浏览: 20
好的,以下是一个简单的两个隐藏层,两个输出的神经网络程序:
```python
import torch
import torch.nn as nn
class Net(nn.Module):
def __init__(self):
super(Net, self).__init__()
self.hidden1 = nn.Linear(10, 20)
self.hidden2 = nn.Linear(20, 30)
self.out1 = nn.Linear(30, 1)
self.out2 = nn.Linear(30, 1)
def forward(self, x):
x = torch.relu(self.hidden1(x))
x = torch.relu(self.hidden2(x))
out1 = self.out1(x)
out2 = self.out2(x)
return out1, out2
# 使用示例
net = Net()
inputs = torch.randn(1, 10)
out1, out2 = net(inputs)
print(out1, out2)
```
该模型包含两个隐藏层,每个隐藏层都有一个线性层和激活函数ReLU,并且有两个输出层。输入是一个大小为(1, 10)的张量,输出是两个标量。您可以根据需要更改输入和输出的大小,以适应您的具体问题。
相关问题
用pytorch写一段神经网络
好的,我将用 PyTorch 写一段基本的神经网络代码。
首先,需要导入 PyTorch 库并设定设备(cpu 或 gpu):
```python
import torch
device = 'cuda' if torch.cuda.is_available() else 'cpu'
```
然后,定义输入和输出的形状:
```python
input_size = 784 # 输入层的节点数,对应图像大小为 28*28
hidden_size = 500 # 隐藏层的节点数
num_classes = 10 # 输出层的节点数,对应分类数量
```
接下来,定义神经网络的结构:
```python
class NeuralNet(torch.nn.Module):
def __init__(self, input_size, hidden_size, num_classes):
super(NeuralNet, self).__init__()
self.fc1 = torch.nn.Linear(input_size, hidden_size)
self.relu = torch.nn.ReLU()
self.fc2 = torch.nn.Linear(hidden_size, num_classes)
def forward(self, x):
out = self.fc1(x)
out = self.relu(out)
out = self.fc2(out)
return out
model = NeuralNet(input_size, hidden_size, num_classes).to(device)
```
在上面的代码中,我们定义了一个包含两个全连接层的神经网络,并使用 ReLU 作为激活函数。这个神经网络有一个输入层、一个隐藏层和一个输出层。
然后,我们可以使用 PyTorch 中的各种优化器和损失函数来训练这个神经网络:
```python
learning_rate = 0.001
# 定义损失函数和优化器
criterion = torch.nn.CrossEntropyLoss
用pytorch写一段用了relu的卷积神经网络
### 回答1:
import torch
import torch.nn as nnclass ConvNet(nn.Module):
def __init__(self):
super(ConvNet, self).__init__()
self.layer1 = nn.Sequential(
nn.Conv2d(1, 32, kernel_size=5, stride=1, padding=2),
nn.ReLU(),
nn.MaxPool2d(kernel_size=2, stride=2))
self.layer2 = nn.Sequential(
nn.Conv2d(32, 64, kernel_size=5, stride=1, padding=2),
nn.ReLU(),
nn.MaxPool2d(kernel_size=2, stride=2))
self.drop_out = nn.Dropout()
self.fc1 = nn.Linear(7 * 7 * 64, 1000)
self.fc2 = nn.Linear(1000, 10)
def forward(self, x):
out = self.layer1(x)
out = self.layer2(out)
out = out.reshape(out.size(0), -1)
out = self.drop_out(out)
out = self.fc1(out)
out = self.fc2(out)
return out我们使用PyTorch来构建一个使用ReLU激活函数的卷积神经网络,具体实现如下:首先使用nn.Conv2d()函数创建2个卷积层,其中第一层卷积层输入通道为1,输出通道为32,卷积核的大小为5*5;第二层卷积层输入通道为32,输出通道为64,卷积核的大小为5*5;之后,两个卷积层后面都跟着一个ReLU激活函数和一个MaxPool2d池化层,池化层的大小为2*2;最后,在卷积层后面添加一个Dropout层和两个全连接层,第一个全连接层将提取出来的特征进行拉平,输出维度为1000,第二个全连接层输出维度为10。
### 回答2:
用PyTorch编写一个卷积神经网络(Convolutional Neural Network, CNN)需要首先导入所需要的库,例如torch,torch.nn,以及torch.nn.functional。然后,通过继承torch.nn.Module类来定义我们的模型。
模型的构建过程大致分为以下几个步骤:
1. 定义初始化函数(init),在该函数中定义神经网络的各个层。
2. 定义前向传播函数(forward),在该函数中定义将输入数据传递给网络的过程。
3. 创建模型的实例。
下面是一个简单的例子,展示了如何使用ReLU激活函数来定义一个卷积神经网络:
```python
import torch
import torch.nn as nn
import torch.nn.functional as F
# 定义卷积神经网络模型
class Net(nn.Module):
def __init__(self):
super(Net, self).__init__()
# 定义卷积层和全连接层
self.conv1 = nn.Conv2d(1, 6, 5) # 输入通道数为1,输出通道数为6,卷积核大小为5x5
self.conv2 = nn.Conv2d(6, 16, 5) # 输入通道数为6,输出通道数为16,卷积核大小为5x5
self.fc1 = nn.Linear(16 * 5 * 5, 120) # 全连接层1,输入维度是16x5x5,输出维度是120
self.fc2 = nn.Linear(120, 84) # 全连接层2,输入维度是120,输出维度是84
self.fc3 = nn.Linear(84, 10) # 全连接层3,输入维度是84,输出维度是10
def forward(self, x):
x = F.relu(self.conv1(x)) # 使用ReLU作为激活函数
x = F.max_pool2d(x, 2) # 使用2x2的最大池化
x = F.relu(self.conv2(x))
x = F.max_pool2d(x, 2)
x = x.view(-1, self.num_flat_features(x)) # 展开张量
x = F.relu(self.fc1(x))
x = F.relu(self.fc2(x))
x = self.fc3(x)
return x
def num_flat_features(self, x):
size = x.size()[1:]
num_features = 1
for s in size:
num_features *= s
return num_features
# 创建模型实例
net = Net()
# 输出网络架构
print(net)
```
在这个例子中,我们定义了一个包含两个卷积层和三个全连接层的卷积神经网络模型。卷积层的激活函数使用的是ReLU函数。在前向传播函数中,我们定义了网络的前向计算过程,即将输入数据依次传递给各个层,并应用激活函数和池化操作。最后,我们创建了一个网络模型的实例。
### 回答3:
下面是一个使用PyTorch编写的卷积神经网络(Convolutional Neural Network,简称CNN)的示例,其中使用了ReLU激活函数:
```python
import torch
import torch.nn as nn
import torch.nn.functional as F
class CNN(nn.Module):
def __init__(self):
super(CNN, self).__init__()
self.conv1 = nn.Conv2d(3, 16, 3) # 输入通道数为3,输出通道数为16,卷积核大小为3x3
self.pool = nn.MaxPool2d(2, 2) # 最大池化层,池化窗口大小为2x2
self.conv2 = nn.Conv2d(16, 32, 3) # 输入通道数为16,输出通道数为32,卷积核大小为3x3
self.fc1 = nn.Linear(32 * 6 * 6, 128) # 全连接层,输入维度为32*6*6,输出维度为128
self.fc2 = nn.Linear(128, 10) # 全连接层,输入维度为128,输出维度为10
def forward(self, x):
x = self.pool(F.relu(self.conv1(x))) # 第一个卷积层,使用ReLU激活函数和最大池化层
x = self.pool(F.relu(self.conv2(x))) # 第二个卷积层,使用ReLU激活函数和最大池化层
x = x.view(-1, 32 * 6 * 6) # 将特征映射展平,以符合全连接层的输入要求
x = F.relu(self.fc1(x)) # 第一个全连接层,使用ReLU激活函数
x = self.fc2(x) # 第二个全连接层,不使用激活函数
return x
# 创建一个CNN实例
model = CNN()
# 打印网络结构
print(model)
```
这段代码构建了一个包含两个卷积层和两个全连接层的简单CNN模型。输入为三通道图像,第一个卷积层的输出通道数为16,第二个卷积层的输出通道数为32,最后一个全连接层的输出维度为10。在前向传播时,使用ReLU作为激活函数,并在卷积层后应用了最大池化层。最后输出模型的结构信息。
相关推荐
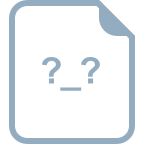
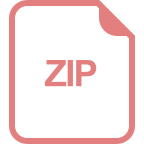












