用C语言实现这行C++代码 pathStack.push(new int[2]{cury, curx});
时间: 2024-02-17 09:05:21 浏览: 8
在C语言中,我们可以使用结构体代替动态内存分配来完成这个操作。下面是一个示例代码:
```c
struct int_arr {
int arr[2];
};
struct int_arr* create_int_arr(int a, int b) {
struct int_arr* new_int_arr = malloc(sizeof(struct int_arr));
new_int_arr->arr[0] = a;
new_int_arr->arr[1] = b;
return new_int_arr;
}
struct int_arr* arr = create_int_arr(cury, curx);
pathStack.push(arr);
```
首先,我们定义了一个名为 `int_arr` 的结构体,它包含一个大小为 2 的整型数组。然后,我们实现了一个名为 `create_int_arr` 的函数,该函数接受两个整数作为参数,并返回指向新分配的 `int_arr` 结构体的指针。该函数通过 `malloc()` 函数为结构体分配内存,并将输入参数存储在数组中。最后,我们创建了一个指向新分配的 `int_arr` 结构体的指针,并将其作为参数传递给 `pathStack.push()` 函数。
相关问题
java swing实现俄罗斯方块代码
下面是一个简单的 Java Swing 实现俄罗斯方块的代码示例:
```java
import java.awt.Color;
import java.awt.Dimension;
import java.awt.Graphics;
import java.awt.Point;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.KeyEvent;
import java.awt.event.KeyListener;
import java.util.ArrayList;
import java.util.Random;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.Timer;
public class Tetris extends JPanel implements ActionListener, KeyListener {
private static final long serialVersionUID = 1L;
private final int WIDTH = 10;
private final int HEIGHT = 22;
private Timer timer;
private boolean isFallingFinished = false;
private boolean isStarted = false;
private boolean isPaused = false;
private int numLinesRemoved = 0;
private int curX = 0;
private int curY = 0;
private JLabel statusbar;
private Shape curPiece;
private Shape.Tetrominoes[] board;
public Tetris(JFrame parent) {
initTetris(parent);
}
private void initTetris(JFrame parent) {
setFocusable(true);
curPiece = new Shape();
timer = new Timer(400, this);
timer.start();
statusbar = new JLabel(" 0");
parent.add(statusbar, BorderLayout.SOUTH);
board = new Shape.Tetrominoes[WIDTH * HEIGHT];
addKeyListener(this);
clearBoard();
}
public void actionPerformed(ActionEvent e) {
if (isFallingFinished) {
isFallingFinished = false;
newPiece();
} else {
oneLineDown();
}
}
private int squareWidth() {
return (int) getSize().getWidth() / WIDTH;
}
private int squareHeight() {
return (int) getSize().getHeight() / HEIGHT;
}
private Shape.Tetrominoes shapeAt(int x, int y) {
return board[(y * WIDTH) + x];
}
public void start() {
if (isPaused)
return;
isStarted = true;
isFallingFinished = false;
numLinesRemoved = 0;
clearBoard();
newPiece();
timer.start();
}
private void pause() {
if (!isStarted)
return;
isPaused = !isPaused;
if (isPaused) {
timer.stop();
statusbar.setText("paused");
} else {
timer.start();
statusbar.setText(String.valueOf(numLinesRemoved));
}
repaint();
}
public void paint(Graphics g) {
super.paint(g);
Dimension size = getSize();
int boardTop = (int) size.getHeight() - HEIGHT * squareHeight();
for (int i = 0; i < HEIGHT; ++i) {
for (int j = 0; j < WIDTH; ++j) {
Shape.Tetrominoes shape = shapeAt(j, HEIGHT - i - 1);
if (shape != Shape.Tetrominoes.NoShape)
drawSquare(g, j * squareWidth(), boardTop + i
* squareHeight(), shape);
}
}
if (curPiece.getShape() != Shape.Tetrominoes.NoShape) {
for (int i = 0; i < 4; ++i) {
int x = curX + curPiece.getX(i);
int y = curY - curPiece.getY(i);
drawSquare(g, x * squareWidth(), boardTop + (HEIGHT - y - 1)
* squareHeight(), curPiece.getShape());
}
}
}
private void dropDown() {
int newY = curY;
while (newY > 0) {
if (!tryMove(curPiece, curX, newY - 1))
break;
--newY;
}
pieceDropped();
}
private void oneLineDown() {
if (!tryMove(curPiece, curX, curY - 1))
pieceDropped();
}
private void clearBoard() {
for (int i = 0; i < HEIGHT * WIDTH; ++i)
board[i] = Shape.Tetrominoes.NoShape;
}
private void pieceDropped() {
for (int i = 0; i < 4; ++i) {
int x = curX + curPiece.getX(i);
int y = curY - curPiece.getY(i);
board[(y * WIDTH) + x] = curPiece.getShape();
}
removeFullLines();
if (!isFallingFinished)
newPiece();
}
private void newPiece() {
curPiece.setRandomShape();
curX = WIDTH / 2 + 1;
curY = HEIGHT - 1 + curPiece.minY();
if (!tryMove(curPiece, curX, curY)) {
curPiece.setShape(Shape.Tetrominoes.NoShape);
timer.stop();
isStarted = false;
statusbar.setText("game over");
}
}
private boolean tryMove(Shape newPiece, int newX, int newY) {
for (int i = 0; i < 4; ++i) {
int x = newX + newPiece.getX(i);
int y = newY - newPiece.getY(i);
if (x < 0 || x >= WIDTH || y < 0 || y >= HEIGHT)
return false;
if (shapeAt(x, y) != Shape.Tetrominoes.NoShape)
return false;
}
curPiece = newPiece;
curX = newX;
curY = newY;
repaint();
return true;
}
private void removeFullLines() {
int numFullLines = 0;
for (int i = HEIGHT - 1; i >= 0; --i) {
boolean lineIsFull = true;
for (int j = 0; j < WIDTH; ++j) {
if (shapeAt(j, i) == Shape.Tetrominoes.NoShape) {
lineIsFull = false;
break;
}
}
if (lineIsFull) {
++numFullLines;
for (int k = i; k < HEIGHT - 1; ++k) {
for (int j = 0; j < WIDTH; ++j)
board[(k * WIDTH) + j] = shapeAt(j, k + 1);
}
}
}
if (numFullLines > 0) {
numLinesRemoved += numFullLines;
statusbar.setText(String.valueOf(numLinesRemoved));
isFallingFinished = true;
curPiece.setShape(Shape.Tetrominoes.NoShape);
repaint();
}
}
private void drawSquare(Graphics g, int x, int y,
Shape.Tetrominoes shape) {
Color colors[] = { new Color(0, 0, 0), new Color(204, 102, 102),
new Color(102, 204, 102), new Color(102, 102, 204),
new Color(204, 204, 102), new Color(204, 102, 204),
new Color(102, 204, 204), new Color(218, 170, 0)
};
Color color = colors[shape.ordinal()];
g.setColor(color);
g.fillRect(x + 1, y + 1, squareWidth() - 2, squareHeight() - 2);
g.setColor(color.brighter());
g.drawLine(x, y + squareHeight() - 1, x, y);
g.drawLine(x, y, x + squareWidth() - 1, y);
g.setColor(color.darker());
g.drawLine(x + 1, y + squareHeight() - 1,
x + squareWidth() - 1, y + squareHeight() - 1);
g.drawLine(x + squareWidth() - 1, y + squareHeight() - 1,
x + squareWidth() - 1, y + 1);
}
public void keyPressed(KeyEvent e) {
if (!isStarted || curPiece.getShape() == Shape.Tetrominoes.NoShape) {
return;
}
int keycode = e.getKeyCode();
if (keycode == 'p' || keycode == 'P') {
pause();
return;
}
if (isPaused)
return;
switch (keycode) {
case KeyEvent.VK_LEFT:
tryMove(curPiece, curX - 1, curY);
break;
case KeyEvent.VK_RIGHT:
tryMove(curPiece, curX + 1, curY);
break;
case KeyEvent.VK_DOWN:
tryMove(curPiece.rotateRight(), curX, curY);
break;
case KeyEvent.VK_UP:
tryMove(curPiece.rotateLeft(), curX, curY);
break;
case KeyEvent.VK_SPACE:
dropDown();
break;
case 'd':
case 'D':
oneLineDown();
break;
}
}
public void keyReleased(KeyEvent e) {
}
public void keyTyped(KeyEvent e) {
}
}
class Shape {
enum Tetrominoes {
NoShape, ZShape, SShape, LineShape, TShape, SquareShape, LShape, MirroredLShape
};
private Tetrominoes pieceShape;
private int coords[][];
private int[][][] coordsTable;
public Shape() {
coords = new int[4][2];
setShape(Tetrominoes.NoShape);
}
public void setShape(Tetrominoes shape) {
coordsTable = new int[][][] {
{ { 0, 0 }, { 0, 0 }, { 0, 0 }, { 0, 0 } },
{ { 0, -1 }, { 0, 0 }, { -1, 0 }, { -1, 1 } },
{ { 0, -1 }, { 0, 0 }, { 1, 0 }, { 1, 1 } },
{ { 0, -1 }, { 0, 0 }, { 0, 1 }, { 0, 2 } },
{ { -1, 0 }, { 0, 0 }, { 1, 0 }, { 0, 1 } },
{ { 0, 0 }, { 1, 0 }, { 0, 1 }, { 1, 1 } },
{ { -1, -1 }, { 0, -1 }, { 0, 0 }, { 0, 1 } },
{ { 1, -1 }, { 0, -1 }, { 0, 0 }, { 0, 1 } }
};
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 2; ++j) {
coords[i][j] = coordsTable[shape.ordinal()][i][j];
}
}
pieceShape = shape;
}
private void setX(int index, int x) {
coords[index][0] = x;
}
private void setY(int index, int y) {
coords[index][1] = y;
}
public int x(int index) {
return coords[index][0];
}
public int y(int index) {
return coords[index][1];
}
public Tetrominoes getShape() {
return pieceShape;
}
public void setRandomShape() {
Random r = new Random();
int x = Math.abs(r.nextInt()) % 7 + 1;
Tetrominoes[] values = Tetrominoes.values();
setShape(values[x]);
}
public int minX() {
int m = coords[0][0];
for (int i = 0; i < 4; i++) {
m = Math.min(m, coords[i][0]);
}
return m;
}
public int minY() {
int m = coords[0][1];
for (int i = 0; i < 4; i++) {
m = Math.min(m, coords[i][1]);
}
return m;
}
public Shape rotateLeft() {
if (pieceShape == Tetrominoes.SquareShape)
return this;
Shape result = new Shape();
result.pieceShape = pieceShape;
for (int i = 0; i < 4; ++i) {
result.setX(i, y(i));
result.setY(i, -x(i));
}
return result;
}
public Shape rotateRight() {
if (pieceShape == Tetrominoes.SquareShape)
return this;
Shape result = new Shape();
result.pieceShape = pieceShape;
for (int i = 0; i < 4; ++i) {
result.setX(i, -y(i));
result.setY(i, x(i));
}
return result;
}
public int getX(int index) {
return coords[index][0];
}
public int getY(int index) {
return coords[index][1];
}
}
public class TetrisGame extends JFrame {
private static final long serialVersionUID = 1L;
public TetrisGame() {
initUI();
}
private void initUI() {
Tetris board = new Tetris(this);
add(board);
setTitle("Tetris");
setSize(400, 700);
setDefaultCloseOperation(EXIT_ON_CLOSE);
setLocationRelativeTo(null);
}
public static void main(String[] args) {
TetrisGame game = new TetrisGame();
game.setVisible(true);
}
}
```
这个代码示例可以直接运行,实现了一个简单的俄罗斯方块游戏。
c++实现俄罗斯方块小游戏
罗斯方块是一款经典的游戏,它的实现可以使用C++语言。游戏的实现需要用到图形界面库和计时器等功能。游戏的主要逻辑是方块的移动和旋转,以及方块与地图的碰撞检测。下面是一个简单的俄罗斯方块游戏实现的代码框架:
```
#include <iostream>
#include <windows.h>
#include <conio.h>
#include <time.h>
using namespace std;
const int WIDTH = 10; // 地图宽度
const int HEIGHT = 20; // 地图高度
const int BLOCK_SIZE = 4; // 方块大小
int map[HEIGHT][WIDTH]; // 地图数组
int block[BLOCK_SIZE][BLOCK_SIZE]; // 方块数组
int curX, curY; // 当前方块的位置
int curBlock; // 当前方块的形状
int nextBlock; // 下一个方块的形状
int score; // 得分
int level; // 等级
// 初始化游戏
void initGame();
// 绘制游戏界面
void drawGame();
// 生成新的方块
void newBlock();
// 方块下落
void blockDown();
// 方块左移
void blockLeft();
// 方块右移
void blockRight();
// 方块旋转
void blockRotate();
// 消除满行
void clearLine();
// 判断游戏是否结束
bool isGameOver();
// 主函数
int main() {
initGame();
while (true) {
drawGame();
blockDown();
if (isGameOver()) {
break;
}
}
return 0;
}
```
其中,initGame()函数用于初始化游戏数据,drawGame()函数用于绘制游戏界面,newBlock()函数用于生成新的方块,blockDown()函数用于方块下落,blockLeft()和blockRight()函数用于方块左右移动,blockRotate()函数用于方块旋转,clearLine()函数用于消除满行,isGameOver()函数用于判断游戏是否结束。
相关推荐
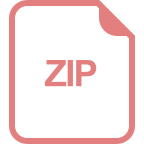












