优化其中的for line in lines: rho, theta = line[0] a = np.cos(theta) b = np.sin(theta) x0 = a * rho y0 = b * rho x1 = int(x0 + 1000 * (-b)) y1 = int(y0 + 1000 * (a)) x2 = int(x0 - 1000 * (-b)) y2 = int(y0 - 1000 * (a))
时间: 2024-02-26 14:59:24 浏览: 17
这段代码是在进行霍夫直线变换后,将检测到的每条直线的参数 rho 和 theta 通过余弦、正弦函数计算出直线的斜率,然后计算直线的两个端点坐标,最终在图像上绘制出这条直线。
如果想要优化这段代码,可以考虑使用 numpy 库提供的向量化运算来代替 for 循环,从而提高代码的运行效率。具体来说,可以将 lines 数组转换为 numpy 数组,然后使用 numpy 库提供的一些函数来实现直线端点坐标的计算。以下是一种可能的优化方法:
```python
# 将 lines 数组转换为 numpy 数组
lines = np.array(lines)
# 计算直线的斜率
rho = lines[:, 0, 0]
theta = lines[:, 0, 1]
a = np.cos(theta)
b = np.sin(theta)
# 计算直线的端点坐标
x0 = a * rho
y0 = b * rho
x1 = np.round(x0 + 1000 * (-b)).astype(int)
y1 = np.round(y0 + 1000 * (a)).astype(int)
x2 = np.round(x0 - 1000 * (-b)).astype(int)
y2 = np.round(y0 - 1000 * (a)).astype(int)
# 组合直线的端点坐标
points = np.stack([np.stack([x1, y1], axis=1),
np.stack([x2, y2], axis=1)], axis=1)
# 在图像上绘制直线
for point in points:
cv2.line(img, tuple(point[0]), tuple(point[1]), (0, 0, 255), 2)
```
这样做的好处是使用了 numpy 库提供的向量化运算,避免了 for 循环的开销,从而提高了代码的运行效率。
相关推荐
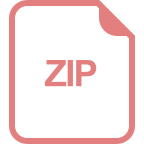
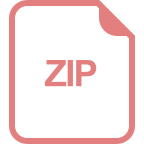
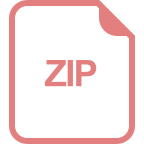














