详细解释:// 1. get camera intrinsic and pose base::BaseCameraModelPtr camera_model = QueryCameraModel(camera); if (camera_model == nullptr) { AERROR << "Failed to get camera model for " << camera->GetSensorId(); return distance; } Eigen::Matrix4d world2camera_pose; if (!QueryWorld2CameraPose(camera, &world2camera_pose)) { AERROR << "Failed to query camera pose"; return distance; } Eigen::Matrix4d lidar2world_pose; if (!QueryLidar2WorldPose(lidar, &lidar2world_pose)) { AERROR << "Failed to query lidar pose"; return distance; } Eigen::Matrix4d lidar2camera_pose = static_cast<Eigen::Matrix<double, 4, 4, 0, 4, 4>>(world2camera_pose * lidar2world_pose);
时间: 2024-04-27 09:25:16 浏览: 11
这段代码主要实现了获取相机内参和位姿,以及获取激光雷达到相机的变换矩阵。具体步骤如下:
1. 先通过传入的相机对象获取相机的内参,即相机的焦距、像素中心点等参数,这里调用了QueryCameraModel函数,返回的是一个BaseCameraModelPtr类型的智能指针,指向了相机模型对象。
2. 如果获取相机模型对象失败,则输出错误信息并返回distance(距离)。
3. 接着调用QueryWorld2CameraPose函数获取相机在世界坐标系下的位姿,即相机的位置和朝向。
4. 如果获取相机位姿失败,则输出错误信息并返回distance。
5. 接着调用QueryLidar2WorldPose函数获取激光雷达在世界坐标系下的位姿。
6. 如果获取激光雷达位姿失败,则输出错误信息并返回distance。
7. 计算激光雷达到相机的变换矩阵,即lidar2camera_pose,这里将相机的位姿和激光雷达的位姿进行矩阵相乘得到。
8. 最后返回distance。
相关问题
转换成matlab:def severed_sphere(intrinsic_process, k1=5.5, k2=2): assert intrinsic_process.shape[0] == 2 intrinsic_process_temp = numpy.copy(intrinsic_process) #intrinsic_process_temp = (intrinsic_process_temp.T-numpy.mean(intrinsic_process_temp, axis=1).T).T observed_process = numpy.empty((3, intrinsic_process_temp.shape[1]), dtype=numpy.float64) observed_process[0] = numpy.sin(intrinsic_process_temp[0]*k1)*numpy.cos(intrinsic_process_temp[1]*k2) observed_process[1] = numpy.cos(intrinsic_process_temp[0]*k1)*numpy.cos(intrinsic_process_temp[1]*k2) observed_process[2] = numpy.sin(intrinsic_process_temp[1]*k2) return observed_process
在Matlab中,您可以将以下Python代码转换为对应的Matlab代码:
```matlab
function observed_process = severed_sphere(intrinsic_process, k1, k2)
assert(size(intrinsic_process, 1) == 2);
intrinsic_process_temp = intrinsic_process;
% intrinsic_process_temp = (intrinsic_process_temp.' - mean(intrinsic_process_temp, 2).').';
observed_process = zeros(3, size(intrinsic_process_temp, 2));
observed_process(1,:) = sin(intrinsic_process_temp(1,:) * k1) .* cos(intrinsic_process_temp(2,:) * k2);
observed_process(2,:) = cos(intrinsic_process_temp(1,:) * k1) .* cos(intrinsic_process_temp(2,:) * k2);
observed_process(3,:) = sin(intrinsic_process_temp(2,:) * k2);
end
```
请注意,在Matlab中没有直接相当于`empty`函数的功能,因此我们使用`zeros`函数来初始化`observed_process`数组。另外,对于行向量和列向量的转置,我们使用了`.`运算符。
liushuideng.c(13): error C264: intrinsic '__nop_': declaration/activation error
这个错误提示是因为编译器找不到 `__nop_` 函数的定义,可能是编译器版本不支持 `__nop_` 函数。
你可以尝试使用其他的延时函数来代替 `__nop_` 函数,比如可以使用 `__asm` 嵌入汇编代码来实现延时,或者使用定时器来产生延时。
以下是使用 `__asm` 嵌入汇编代码实现延时的例子:
```c
void Delay10us(void)
{
__asm
NOP
NOP
NOP
NOP
NOP
NOP
NOP
NOP
NOP
NOP
__endasm;
}
```
或者使用定时器来产生延时的例子:
```c
void Delay10us(void)
{
TMOD &= 0xF0;
TMOD |= 0x01;
TH0 = 0xFF;
TL0 = 0xF6;
TR0 = 1;
while (TF0 == 0);
TR0 = 0;
TF0 = 0;
}
```
请注意,以上的代码仅供参考,具体实现方式需要根据实际情况进行调整。
相关推荐
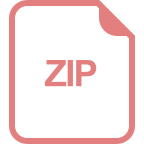
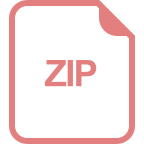












