修改代码,使startGame方法运行到执行getHinder()后回到for(;success;)
时间: 2024-03-03 08:51:04 浏览: 48
很抱歉,由于我并不知道你的具体代码,我无法提供修改建议。但是,一般来说,如果你想要回到 `for(;success;)` 中执行,你可以将 `getHinder()` 的返回值作为 `success` 的判断条件之一,例如:
```java
for (boolean success = true; success; ) {
// ...
int hinder = getHinder();
if (hinder == 0) {
success = false;
} else {
// do something with hinder
}
// ...
}
```
这样,当 `getHinder()` 返回 0 时,`success` 被置为 false,循环停止,否则循环继续执行。
相关问题
修改代码,使startGame方法运行到执行getHinder()后回到for(;success;) public void startGame() { Scanner scanner = new Scanner(System.in); while (true) { System.out.println("请选择游戏模式(1-娱乐模式,2-困难模式,0-退出):"); int mode = scanner.nextInt(); if (mode == 0) { break; } List<ChengYu> chengYuList; if (mode == 1) { chengYuList = commonChengYuList; } else { chengYuList = fullChengYuList; } Collections.shuffle(chengYuList); // 打乱顺序 int score = 0; boolean success = true; //随机抽取一个成语 Random random = new Random(); int index = random.nextInt(chengYuList.size()); ChengYu cy = chengYuList.get(index); String currentIdiom = cy.getChengYu(); for(;success;) { System.out.println(hintCount); for (ChengYu chengYu : commonChengYuList) if(chengYu.getChengYu().startsWith((currentIdiom.substring(currentIdiom.length() - 1)))) break; System.out.println("请回答以下成语的下一个成语:" + currentIdiom + "(难度:" + cy.getDifficultyLevel().getName() + ")"); String answer = scanner.next(); if (!cy.isCorrectAnswer(answer,commonChengYuList)) { System.out.println("回答错误!"); success = false; getHint(currentIdiom); } else { score++; } if (success) { System.out.println("恭喜您回答正确,得分:" + score); for (ChengYu nextChengYu : fullChengYuList) if(nextChengYu.getChengYu().startsWith((answer.substring(answer.length() - 1)))) currentIdiom=nextChengYu.getChengYu(); } } } scanner.close(); } //给予用户最多3次提示的机会 private int maxHintCount=3; // 最大提示次数 private int hintCount=0; // 当前提示次数 public String getHint(String currentIdiom) { if (hintCount >= maxHintCount) { System.out.println("提示次数已全部用完,请重新开始游戏!"); hintCount=0; return null; // 已经用完所有提示次数,返回null } for (ChengYu idiom : fullChengYuList) { if(hintCount<maxHintCount) { String reminder=idiom.getChengYu(); if (reminder.startsWith(currentIdiom.substring(currentIdiom.length() - 1))) { System.out.println("提示:"+reminder); hintCount++; break; } } } return null; }
在 `getHint()` 方法中,当提示次数已经用完时,需要将 `hintCount` 重置为 0,否则在下一轮游戏时,提示次数将被累加上一轮游戏中剩余的提示次数,从而导致提示次数异常。修改后的代码如下:
```java
public String getHint(String currentIdiom) {
if (hintCount >= maxHintCount) {
System.out.println("提示次数已全部用完,请重新开始游戏!");
int hintCount = 0; // 重置 hintCount 为 0
return null; // 已经用完所有提示次数,返回null
}
for (ChengYu idiom : fullChengYuList) {
if(hintCount<maxHintCount) {
String reminder=idiom.getChengYu();
if (reminder.startsWith(currentIdiom.substring(currentIdiom.length() - 1))) {
System.out.println("提示:"+reminder);
hintCount++;
break;
}
}
}
return null;
}
```
另外,在 `startGame()` 方法中,如果用户回答错误时,需要在调用 `getHint()` 方法后重新进入循环判断。修改后的代码如下:
```java
if (!cy.isCorrectAnswer(answer,commonChengYuList)) {
System.out.println("回答错误!");
success = false;
getHint(currentIdiom);
continue; // 重新进入循环判断
} else {
score++;
}
```
这样,当用户回答错误时,程序会调用 `getHint()` 方法给予提示,并重新进入循环判断,直到用户回答正确或者提示次数用完为止。
public class BaseBallPlayer { private String pname;//球员名称 public BaseBallPlayer (String name) { pname=name; } public void strikeBall() {//击球 System.out.println(pname+" strikes the ball!"); } public void catchBall() {//接球 System.out.println(pname+" catches the ball!"); } public void runBase() {//跑垒 System.out.println(pname+" runs to the base!"); } }基于这段代码,设计BaseBallTeam类,除构造方法和继承的属性或方法外,仅对外提供addPlayer和startGame方法。BaseBallTeam可以存放多个类型为Striker或Catcher的球员。BaseBallTeam中的play方法继承于Striker类和Catcher类 (1)addPlayer方法的功能为:若当前 BaseBallTeam 未满员,则添加一个球员 对象到 BaseBallTeam 中,否则打印“球队满员,请另寻他处。” 2) startGame 方法的功能为:若球员人数大于 0,则让当前 BaseBallTeam 中 的全部球员对象逐一进行游戏,否则打印“没有球员可以开始游戏。”
以下是基于给出代码的BaseBallTeam类设计:
```
public class BaseBallTeam extends BaseBallPlayer {
private ArrayList<BaseBallPlayer> players;
private final int maxPlayers = 10; // 球队最大人数
public BaseBallTeam() {
super("");
players = new ArrayList<BaseBallPlayer>();
}
public void addPlayer(BaseBallPlayer player) {
if (players.size() < maxPlayers) {
players.add(player);
System.out.println(player.pname + " has joined the team.");
} else {
System.out.println("The team is full. Please find another one.");
}
}
public void startGame() {
if (players.size() > 0) {
System.out.println("The game begins!");
for (BaseBallPlayer player : players) {
player.strikeBall();
player.catchBall();
player.runBase();
}
} else {
System.out.println("There are no players to start the game.");
}
}
}
```
在这个类中,我们使用了一个ArrayList来存储球队中的球员对象,addPlayer方法可以将一个球员对象添加到这个ArrayList中,如果球队已经满员,则输出提示信息。startGame方法可以让球队中的所有球员逐一进行游戏,如果球员人数为0,则输出提示信息。此外,这个类还继承了BaseBallPlayer类中的方法,包括strikeBall、catchBall和runBase方法。因此,这个类中的play方法也就继承了Striker类和Catcher类中的play方法。
阅读全文
相关推荐















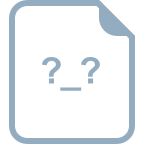
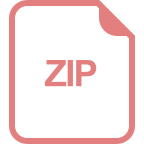