using C++ to Write a program that takes a string input from the user and counts the number of unique characters in the string. Note:The program should only count each unique character once, even if it appears multiple times in the input string. The program should also ignore any whitespace characters or special characters that are not letters or numbers. Example: : Input: hello world Output: 7 (unique characters are (h, e, l, o, w, r, d)).同时将单个字符输出
时间: 2024-02-14 21:09:19 浏览: 76
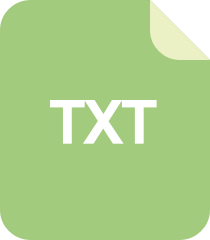
一个C++语言编写的程序
好的,以下是使用C++编写的程序,可以实现从用户输入的字符串中计算唯一字符的数量,并输出每个唯一字符:
```
#include <iostream>
#include <string>
#include <unordered_set>
int main() {
std::string input_str;
std::getline(std::cin, input_str); // 从用户输入读入字符串
std::unordered_set<char> unique_chars;
for (char c : input_str) {
if (std::isalnum(c)) { // 判断字符是否为字母或数字
unique_chars.insert(c); // 将唯一字符插入到无序集合中
}
}
std::cout << unique_chars.size() << " (unique characters are: ";
for (char c : unique_chars) {
std::cout << c << " ";
}
std::cout << ")" << std::endl; // 输出唯一字符的数量和每个唯一字符
return 0;
}
```
程序首先从用户输入中读取字符串,然后使用`std::unordered_set`来存储唯一的字符。在遍历输入字符串的每个字符时,程序判断字符是否为字母或数字,如果是,则将其插入到无序集合中。由于无序集合只能存储唯一元素,因此重复的字符只会被计数一次。最后,程序输出唯一字符的数量和每个唯一字符。
注意,程序需要包含头文件`<iostream>`、`<string>`和`<unordered_set>`,并且需要使用命名空间`std`。此外,程序还需要使用`std::getline()`函数来读取包含空格的输入字符串。
阅读全文
相关推荐















