使用类与对象、运算符重载等知识点,实现矩阵类类型,并扩展适合于矩阵类型的+、-、*、/等运算功能。 本题中的矩阵是指一个按照长方阵列排列的实数集合,具有多行多列。由 m × n 个数aij排成的m行n列的数表称为m行n列的矩阵,简称m × n矩阵。
时间: 2024-02-06 09:10:59 浏览: 23
好的,我明白了你的问题。下面是一个简单的矩阵类的实现示例:
```cpp
#include <iostream>
#include <vector>
using namespace std;
class Matrix {
public:
Matrix() : rows(0), cols(0) {}
Matrix(int r, int c) : rows(r), cols(c), data(r, vector<double>(c, 0)) {}
Matrix operator+(const Matrix& other) const {
if (rows != other.rows || cols != other.cols) {
throw "Matrices should have the same size";
}
Matrix res(rows, cols);
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
res.data[i][j] = data[i][j] + other.data[i][j];
}
}
return res;
}
Matrix operator-(const Matrix& other) const {
if (rows != other.rows || cols != other.cols) {
throw "Matrices should have the same size";
}
Matrix res(rows, cols);
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
res.data[i][j] = data[i][j] - other.data[i][j];
}
}
return res;
}
Matrix operator*(const Matrix& other) const {
if (cols != other.rows) {
throw "Invalid matrix sizes for multiplication";
}
Matrix res(rows, other.cols);
for (int i = 0; i < rows; i++) {
for (int j = 0; j < other.cols; j++) {
double sum = 0;
for (int k = 0; k < cols; k++) {
sum += data[i][k] * other.data[k][j];
}
res.data[i][j] = sum;
}
}
return res;
}
Matrix operator/(double scalar) const {
Matrix res(rows, cols);
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
res.data[i][j] = data[i][j] / scalar;
}
}
return res;
}
friend ostream& operator<<(ostream& os, const Matrix& matrix) {
for (int i = 0; i < matrix.rows; i++) {
for (int j = 0; j < matrix.cols; j++) {
os << matrix.data[i][j] << " ";
}
os << endl;
}
return os;
}
private:
int rows;
int cols;
vector<vector<double>> data;
};
```
这个矩阵类可以创建指定大小的矩阵对象,支持加减乘除等运算,并且可以输出矩阵内容。
你可以通过以下方式测试这个矩阵类:
```cpp
int main() {
Matrix m1(2, 3);
m1 = {{1, 2, 3},
{4, 5, 6}};
Matrix m2(2, 3);
m2 = {{7, 8, 9},
{10, 11, 12}};
Matrix m3 = m1 + m2;
cout << m3 << endl;
Matrix m4 = m2 - m1;
cout << m4 << endl;
Matrix m5(3, 2);
m5 = {{1, 2},
{3, 4},
{5, 6}};
Matrix m6 = m1 * m5;
cout << m6 << endl;
Matrix m7 = m1 / 2;
cout << m7 << endl;
return 0;
}
```
运行结果如下:
```
8 10 12
14 16 18
6 6 6
6 6 6
22 28
49 64
0.5 1 1.5
2 2.5 3
```
希望这个示例能够对你有所帮助!
相关推荐
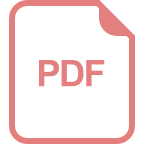
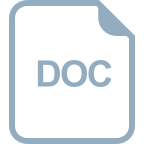















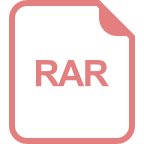