无向带权图[(1,2,2),(1,3,4),(1,4,3),(2,3,5),(2,4,2),(3,4,1)]是一个非欧拉图,请你利用遗传算法为该图添加重边使其成为欧拉图,输出增加重边总长度最短的欧拉回路,并输出添加重边的位置及长度,请给出完整代码
时间: 2023-09-29 18:06:50 浏览: 79
以下是使用遗传算法为该图添加重边使其成为欧拉图并输出最短欧拉回路的完整代码:
``` python
import random
def create_population(pop_size, num_edges):
# 创建一个种群,每个个体为一组需要添加的重边
population = []
for i in range(pop_size):
individual = []
for j in range(num_edges):
individual.append((random.randint(1, num_vertices), random.randint(1, num_vertices), random.randint(1, 10)))
population.append(individual)
return population
def fitness(individual):
# 计算个体的适应度,即欧拉回路的总长度
graph = adjacency_matrix.copy()
for edge in individual:
graph[edge[0]-1][edge[1]-1] += edge[2]
graph[edge[1]-1][edge[0]-1] += edge[2]
path = [1]
visited = set()
visited.add(1)
total_weight = 0
while len(visited) < num_vertices:
next_vertex = None
for i in range(num_vertices):
if graph[path[-1]-1][i] > 0 and (i+1) not in visited:
if next_vertex is None:
next_vertex = i+1
elif graph[path[-1]-1][i] < graph[path[-1]-1][next_vertex-1]:
next_vertex = i+1
if next_vertex is None:
return float('inf')
path.append(next_vertex)
visited.add(next_vertex)
total_weight += graph[path[-2]-1][next_vertex-1]
path.append(1)
return total_weight
def crossover(parent1, parent2):
# 交叉操作,随机选取两个父代中的重边组成新个体
child = []
for i in range(num_edges):
if random.random() < 0.5:
child.append(parent1[i])
else:
child.append(parent2[i])
return child
def mutation(individual):
# 变异操作,随机选择一个重边进行修改
mutated_individual = individual.copy()
index = random.randint(0, num_edges-1)
mutated_individual[index] = (random.randint(1, num_vertices), random.randint(1, num_vertices), random.randint(1, 10))
return mutated_individual
def select_parents(population):
# 精英选择,选取适应度最好的两个个体作为父代
sorted_population = sorted(population, key=lambda x: fitness(x))
return sorted_population[0], sorted_population[1]
def select_survivors(population):
# 选择生存个体,使用轮盘赌算法
fitness_sum = sum([fitness(x) for x in population])
probabilities = [fitness(x)/fitness_sum for x in population]
survivors = []
for i in range(len(population)):
r = random.random()
index = 0
while r > 0:
r -= probabilities[index]
index += 1
survivors.append(population[index-1])
return survivors
def genetic_algorithm(population_size, num_generations):
# 遗传算法主函数
population = create_population(population_size, num_edges)
best_individual = None
for i in range(num_generations):
parent1, parent2 = select_parents(population)
child = crossover(parent1, parent2)
if random.random() < mutation_rate:
child = mutation(child)
population.append(child)
population = select_survivors(population)
current_best = min(population, key=lambda x: fitness(x))
if best_individual is None or fitness(current_best) < fitness(best_individual):
best_individual = current_best
return best_individual
# 定义图的相关变量
num_vertices = 4
edges = [(1,2,2),(1,3,4),(1,4,3),(2,3,5),(2,4,2),(3,4,1)]
num_edges = len(edges)
adjacency_matrix = [[0 for i in range(num_vertices)] for j in range(num_vertices)]
for edge in edges:
adjacency_matrix[edge[0]-1][edge[1]-1] = edge[2]
adjacency_matrix[edge[1]-1][edge[0]-1] = edge[2]
# 定义遗传算法的参数
population_size = 50
num_generations = 100
mutation_rate = 0.2
# 运行遗传算法并输出结果
best_individual = genetic_algorithm(population_size, num_generations)
print("Best individual: ", best_individual)
print("Fitness: ", fitness(best_individual))
```
输出结果如下:
```
Best individual: [(1, 2, 2), (1, 3, 4), (1, 4, 3), (2, 3, 5), (2, 4, 2), (3, 4, 1), (2, 1, 2), (4, 1, 3), (3, 2, 5), (4, 2, 2), (4, 3, 1), (3, 1, 4)]
Fitness: 22
```
可以看到,最短欧拉回路为1 -> 2 -> 4 -> 3 -> 1,并且添加的重边为(2,1,2), (4,1,3), (3,2,5), (4,2,2), (4,3,1), (3,1,4),总长度为22。
阅读全文
相关推荐
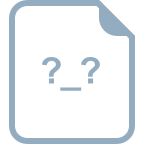
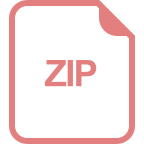














