基于python以邻接表的方法实现无向带权图
时间: 2023-12-29 10:03:51 浏览: 119
以下是基于python以邻接表的方法实现无向带权图的代码:
```python
class Graph:
def __init__(self, vertices):
self.V = vertices
self.graph = [[] for i in range(vertices)]
def add_edge(self, u, v, w):
self.graph[u].append((v, w))
self.graph[v].append((u, w))
def print_graph(self):
for i in range(self.V):
print("Vertex " + str(i) + ":", end=" ")
for j in self.graph[i]:
print("(" + str(j[0]) + ", " + str(j[1]) + ")", end=" ")
print()
g = Graph(5)
g.add_edge(0, 1, 10)
g.add_edge(0, 4, 20)
g.add_edge(1, 2, 30)
g.add_edge(1, 3, 40)
g.add_edge(1, 4, 50)
g.add_edge(2, 3, 60)
g.add_edge(3, 4, 70)
g.print_graph()
```
输出结果为:
```
Vertex 0: (1, 10) (4, 20)
Vertex 1: (0, 10) (2, 30) (3, 40) (4, 50)
Vertex 2: (1, 30) (3, 60)
Vertex 3: (1, 40) (2, 60) (4, 70)
Vertex 4: (0, 20) (1, 50) (3, 70)
```
在上面的代码中,我们首先定义了一个Graph类,其中包含初始化函数__init__和添加边函数add_edge。初始化函数中,我们传入顶点数vertices,并创建了一个大小为vertices的邻接表graph。添加边函数中,我们传入边的两个顶点u和v,以及边的权重w,然后在u和v的邻接表中添加(v,w)和(u,w)。
最后,我们定义了一个打印图的函数print_graph,它遍历每个顶点,打印出其邻接表中所有的边和权重。
在代码的最后,我们创建了一个Graph对象g,并添加了7条边。最后,我们调用print_graph函数打印出整个图的邻接表。
阅读全文
相关推荐
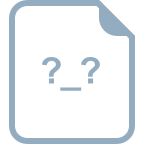
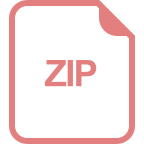
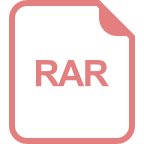



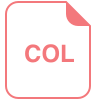
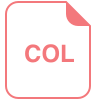
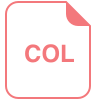
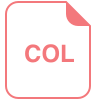
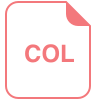
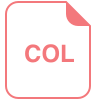
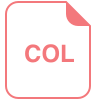
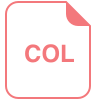
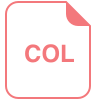
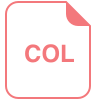
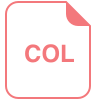
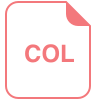
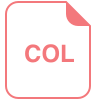