顺时针排螺旋阵 给你一个N行N列的方格矩阵,从外圈按顺时针依次填写自然数,这会构成一个螺旋阵,你能编程实现吗? 比如5行5列的情况如下: 1 2 3 4 5 16 17 18 19 6 15 24 25 20 7 14 23 22 21 8 13 12 11 10 9 输入 输入一个正整数数N(N<100)。 输出 输出符合题意的螺旋阵
时间: 2024-01-03 12:03:52 浏览: 29
可以使用循环来按照顺时针方向依次填入自然数。具体来说,每次填入一个数后,就判断下一个应该填入的位置是否已经被填过,如果是就改变方向,否则继续按当前方向前进。
以下是实现这个算法的 C++ 代码:
```c++
#include <iostream>
using namespace std;
int main() {
int n;
cin >> n;
int matrix[100][100] = {0}; // 初始化为0
// 定义四个方向,顺时针方向从右开始
int directionRow[] = {0, 1, 0, -1};
int directionCol[] = {1, 0, -1, 0};
int row = 0, col = 0, direction = 0;
for (int i = 1; i <= n * n; i++) {
matrix[row][col] = i;
// 计算下一个位置
int nextRow = row + directionRow[direction];
int nextCol = col + directionCol[direction];
// 判断下一个位置是否越界或已经被填过
if (nextRow < 0 || nextRow >= n || nextCol < 0 || nextCol >= n || matrix[nextRow][nextCol] != 0) {
// 改变方向
direction = (direction + 1) % 4;
nextRow = row + directionRow[direction];
nextCol = col + directionCol[direction];
}
row = nextRow;
col = nextCol;
}
// 输出螺旋阵
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++) {
cout << matrix[i][j] << " ";
}
cout << endl;
}
return 0;
}
```
输入一个正整数 $n$ 后,这段代码会输出一个 $n\times n$ 的符合题意的螺旋阵。例如,当 $n=5$ 时,输出结果如下:
```
1 2 3 4 5
16 17 18 19 6
15 24 25 20 7
14 23 22 21 8
13 12 11 10 9
```
这就是一个 $5\times 5$ 的符合题意的螺旋阵。
相关推荐
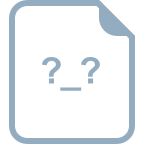
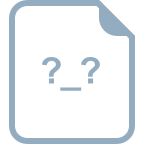














