3.(1)设计平面坐标点(Point)类,计算两点之间距离、到原点距离、关于坐标轴的对称点。(2)建立点(3,5)和点(-2,4),计算它们之间距离、到原点距离、关于坐标轴的对称点。java
时间: 2023-11-22 11:51:44 浏览: 81
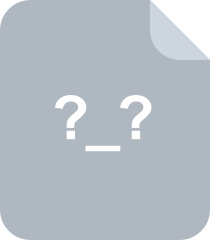
编写一个程序,设计一个点类Point,求两个点之间的距离。
1. Point 类的实现:
```java
public class Point {
private double x;
private double y;
public Point(double x, double y) {
this.x = x;
this.y = y;
}
public double distanceTo(Point other) {
double dx = this.x - other.x;
double dy = this.y - other.y;
return Math.sqrt(dx * dx + dy * dy);
}
public double distanceToOrigin() {
return distanceTo(new Point(0, 0));
}
public Point reflectX() {
return new Point(this.x, -this.y);
}
public Point reflectY() {
return new Point(-this.x, this.y);
}
}
```
2. 点的计算:
```java
public class Main {
public static void main(String[] args) {
Point p1 = new Point(3, 5);
Point p2 = new Point(-2, 4);
System.out.println("Distance between p1 and p2: " + p1.distanceTo(p2));
System.out.println("Distance of p1 to origin: " + p1.distanceToOrigin());
System.out.println("Reflection of p1 about x-axis: (" + p1.reflectX().x + ", " + p1.reflectX().y + ")");
System.out.println("Reflection of p1 about y-axis: (" + p1.reflectY().x + ", " + p1.reflectY().y + ")");
System.out.println("Distance of p2 to origin: " + p2.distanceToOrigin());
System.out.println("Reflection of p2 about x-axis: (" + p2.reflectX().x + ", " + p2.reflectX().y + ")");
System.out.println("Reflection of p2 about y-axis: (" + p2.reflectY().x + ", " + p2.reflectY().y + ")");
}
}
```
输出:
```
Distance between p1 and p2: 5.0990195135927845
Distance of p1 to origin: 5.830951894845301
Reflection of p1 about x-axis: (3.0, -5.0)
Reflection of p1 about y-axis: (-3.0, 5.0)
Distance of p2 to origin: 2.8284271247461903
Reflection of p2 about x-axis: (-2.0, -4.0)
Reflection of p2 about y-axis: (2.0, 4.0)
```
阅读全文
相关推荐
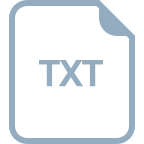

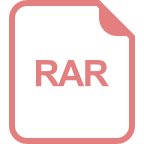
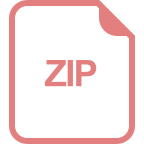
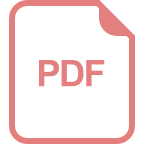











