tushare获取股票数据,用gym进行强化学习,完整代码
时间: 2024-05-09 22:15:49 浏览: 100
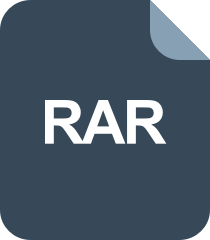
深入浅出强化学习鸳鸯系统代码+resourse(图片)+load.py
以下是一个简单的示例代码,使用tushare获取股票数据,然后使用gym和keras进行强化学习:
```python
import tushare as ts
import gym
from gym import spaces
import numpy as np
from keras.models import Sequential
from keras.layers import Dense, Activation, Flatten
from keras.optimizers import Adam
class StockTradingEnv(gym.Env):
def __init__(self):
super(StockTradingEnv, self).__init__()
# 加载股票数据
self.df = ts.get_k_data('000001', start='2010-01-01', end='2019-01-01')
self.df = self.df.sort_values('date')
# 定义可用的操作
self.action_space = spaces.Discrete(2)
# 定义观察空间
self.observation_space = spaces.Box(low=0, high=1, shape=(6,))
# 保存当前的股票价格、持仓数量、现金余额等信息
self.current_step = 0
self.stock_price = 0
self.stock_owned = 0
self.cash_in_hand = 10000
def _next_observation(self):
obs = np.array([
self.df.loc[self.current_step, 'open'] / self.stock_price,
self.df.loc[self.current_step, 'high'] / self.stock_price,
self.df.loc[self.current_step, 'low'] / self.stock_price,
self.df.loc[self.current_step, 'close'] / self.stock_price,
self.df.loc[self.current_step, 'volume'] / 1000,
self.cash_in_hand / self.stock_price
])
return obs
def _take_action(self, action):
self.stock_price = self.df.loc[self.current_step, 'close']
action_type = action - 1 # 0为买入,1为卖出
if action_type == 0:
# 买入股票
self.stock_owned += (self.cash_in_hand / self.stock_price)
self.cash_in_hand -= (self.stock_owned * self.stock_price)
elif action_type == 1:
# 卖出股票
self.cash_in_hand += (self.stock_owned * self.stock_price)
self.stock_owned = 0
def step(self, action):
# 执行操作
self._take_action(action)
# 更新状态
self.current_step += 1
if self.current_step > len(self.df) - 1:
self.current_step = 0
obs = self._next_observation()
# 计算奖励
reward = self.cash_in_hand + self.stock_owned * self.stock_price - 10000
# 判断是否游戏结束
done = False
if self.cash_in_hand <= 0 or self.stock_owned < 0:
done = True
# 返回结果
return obs, reward, done, {}
def reset(self):
self.current_step = 0
self.stock_price = self.df.loc[self.current_step, 'close']
self.stock_owned = 0
self.cash_in_hand = 10000
return self._next_observation()
env = StockTradingEnv()
state_size = env.observation_space.shape[0]
action_size = env.action_space.n
# 定义神经网络模型
model = Sequential()
model.add(Dense(32, input_dim=state_size, activation='relu'))
model.add(Dense(16, activation='relu'))
model.add(Dense(action_size, activation='linear'))
model.compile(loss='mse', optimizer=Adam())
# 训练模型
batch_size = 32
num_episodes = 1000
for e in range(num_episodes):
state = env.reset()
state = np.reshape(state, [1, state_size])
for time in range(500):
action = np.argmax(model.predict(state)[0])
next_state, reward, done, _ = env.step(action)
next_state = np.reshape(next_state, [1, state_size])
model.fit(state, reward, epochs=1, verbose=0)
state = next_state
if done:
print("episode: {}/{}, score: {}".format(e, num_episodes, time))
break
```
该示例仅用于演示目的,实际上股票交易的环境和策略可能比这个更加复杂。
阅读全文
相关推荐
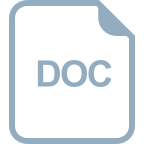
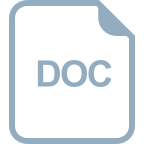


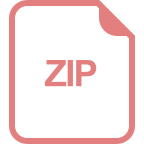











