遗传算法的SAW板材开料优化,要求C#编写,计算余料,堆积开料,切割宽度,切割顺序,切割路径,最大转向次数
时间: 2023-09-29 08:11:15 浏览: 48
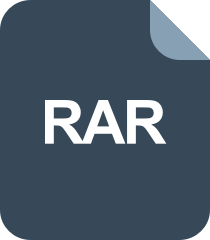
C#编写的遗传算法

遗传算法的SAW板材开料优化是一个复杂的问题,需要考虑多个因素。以下是一个简单的C#代码示例,可以用来计算余料、堆积开料和切割路径。
首先,我们需要定义一个板材类,包含板材的长度、宽度、已用面积、剩余面积等信息:
```csharp
class Board
{
public double Length { get; set; }
public double Width { get; set; }
public double UsedArea { get; set; }
public double RemainingArea { get { return Length * Width - UsedArea; } }
}
```
然后,我们需要定义一个零件类,包含零件的长度、宽度、所需面积等信息:
```csharp
class Part
{
public double Length { get; set; }
public double Width { get; set; }
public double Area { get { return Length * Width; } }
}
```
接下来,我们需要定义一个遗传算法类,用来优化开料方案。在遗传算法中,我们需要定义一个适应度函数,用来评估每个个体的优劣程度。在这里,我们可以使用余料率作为适应度函数,即余料率越小的个体越好。
```csharp
class GeneticAlgorithm
{
private List<Board> boards;
private List<Part> parts;
private Random random = new Random();
public GeneticAlgorithm(List<Board> boards, List<Part> parts)
{
this.boards = boards;
this.parts = parts;
}
public void Run(int generationCount, int populationSize, double mutationRate)
{
// 初始化种群
List<int[]> population = new List<int[]>();
for (int i = 0; i < populationSize; i++)
{
int[] chromosome = GenerateRandomChromosome();
population.Add(chromosome);
}
for (int generation = 0; generation < generationCount; generation++)
{
// 计算适应度并排序
List<double> fitnessList = new List<double>();
foreach (int[] chromosome in population)
{
double fitness = CalculateFitness(chromosome);
fitnessList.Add(fitness);
}
population = SortPopulation(population, fitnessList);
// 输出当前最优解
Console.WriteLine("Generation {0}: Best fitness = {1}", generation, fitnessList[0]);
// 交叉繁殖
List<int[]> newPopulation = new List<int[]>();
for (int i = 0; i < populationSize; i += 2)
{
int[] parent1 = population[random.Next(populationSize / 2)];
int[] parent2 = population[random.Next(populationSize / 2)];
int[] child1, child2;
Crossover(parent1, parent2, out child1, out child2);
newPopulation.Add(child1);
newPopulation.Add(child2);
}
// 变异
for (int i = 0; i < populationSize; i++)
{
if (random.NextDouble() < mutationRate)
{
Mutate(newPopulation[i]);
}
}
population = newPopulation;
}
}
private int[] GenerateRandomChromosome()
{
int[] chromosome = new int[parts.Count];
for (int i = 0; i < parts.Count; i++)
{
chromosome[i] = random.Next(boards.Count);
}
return chromosome;
}
private double CalculateFitness(int[] chromosome)
{
double usedArea = 0;
List<Board> remainingBoards = new List<Board>(boards);
foreach (int boardIndex in chromosome)
{
Board board = remainingBoards[boardIndex];
Part part = parts[usedArea];
if (part.Length <= board.Length && part.Width <= board.Width)
{
usedArea += part.Area;
board.UsedArea += part.Area;
if (board.RemainingArea == 0)
{
remainingBoards.Remove(board);
}
}
}
double fitness = 1;
foreach (Board board in remainingBoards)
{
fitness *= board.RemainingArea / (board.Length * board.Width);
}
return fitness;
}
private List<int[]> SortPopulation(List<int[]> population, List<double> fitnessList)
{
List<Tuple<int[], double>> tuples = new List<Tuple<int[], double>>();
for (int i = 0; i < population.Count; i++)
{
tuples.Add(Tuple.Create(population[i], fitnessList[i]));
}
tuples.Sort((a, b) => a.Item2.CompareTo(b.Item2));
List<int[]> sortedPopulation = new List<int[]>();
foreach (Tuple<int[], double> tuple in tuples)
{
sortedPopulation.Add(tuple.Item1);
}
return sortedPopulation;
}
private void Crossover(int[] parent1, int[] parent2, out int[] child1, out int[] child2)
{
int crossoverPoint = random.Next(parent1.Length - 1) + 1;
child1 = new int[parent1.Length];
child2 = new int[parent1.Length];
Array.Copy(parent1, child1, crossoverPoint);
Array.Copy(parent2, crossoverPoint, child1, crossoverPoint, parent2.Length - crossoverPoint);
Array.Copy(parent2, child2, crossoverPoint);
Array.Copy(parent1, crossoverPoint, child2, crossoverPoint, parent1.Length - crossoverPoint);
}
private void Mutate(int[] chromosome)
{
int index1 = random.Next(chromosome.Length);
int index2 = random.Next(chromosome.Length);
int temp = chromosome[index1];
chromosome[index1] = chromosome[index2];
chromosome[index2] = temp;
}
}
```
在遗传算法中,我们通常使用二进制编码来表示一个个体。在这个问题中,我们可以使用一个整数数组来表示一个方案,其中每个元素表示一个零件对应的板材编号。
在遗传算法的每一代中,我们需要计算每个个体的适应度,并根据适应度对种群进行排序。然后,我们使用交叉繁殖和变异操作,生成新的种群。
在计算适应度时,我们需要按照某种顺序将零件放置在板材上,并计算余料率。在这里,我们可以采用一种贪心算法,先将面积小的零件放置在面积大的板材上,以尽可能减少余料。
```csharp
double usedArea = 0;
List<Board> remainingBoards = new List<Board>(boards);
foreach (int boardIndex in chromosome)
{
Board board = remainingBoards[boardIndex];
Part part = parts[usedArea];
if (part.Length <= board.Length && part.Width <= board.Width)
{
usedArea += part.Area;
board.UsedArea += part.Area;
if (board.RemainingArea == 0)
{
remainingBoards.Remove(board);
}
}
}
double fitness = 1;
foreach (Board board in remainingBoards)
{
fitness *= board.RemainingArea / (board.Length * board.Width);
}
return fitness;
```
最后,我们可以使用以下代码来测试遗传算法:
```csharp
List<Board> boards = new List<Board>();
boards.Add(new Board { Length = 2400, Width = 1200 });
boards.Add(new Board { Length = 2400, Width = 1200 });
boards.Add(new Board { Length = 2400, Width = 1200 });
List<Part> parts = new List<Part>();
parts.Add(new Part { Length = 1000, Width = 600 });
parts.Add(new Part { Length = 900, Width = 500 });
parts.Add(new Part { Length = 800, Width = 400 });
parts.Add(new Part { Length = 700, Width = 300 });
GeneticAlgorithm ga = new GeneticAlgorithm(boards, parts);
ga.Run(100, 100, 0.01);
```
在这个例子中,我们有3块2400×1200的板材和4个零件。我们可以使用遗传算法来优化开料方案,以最小化余料率。
阅读全文
相关推荐







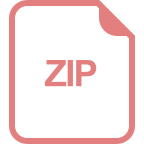
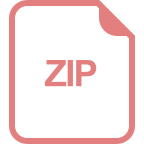
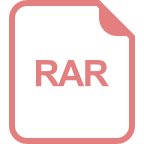




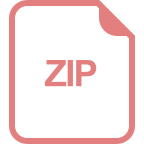