用matlab做一个app 2、功能包括:二分法求根、不动点迭代求根、牛顿法求根、fsolve求根 3、求以下方程的全部根 x^2-e^x=0
时间: 2024-04-05 07:32:28 浏览: 37
好的,以下是一个简单的 MATLAB App Designer 程序,可以实现二分法求根、不动点迭代求根、牛顿法求根和 fsolve 求根。程序包括以下部分:
1. 一个输入框,用于输入方程参数。
2. 一个下拉菜单,用于选择求根方法。
3. 一个输入框,用于输入初始值(仅在不动点迭代和牛顿法中需要)。
4. 一个按钮,用于计算结果。
5. 一个输出框,用于显示结果。
以下是程序代码:
```
classdef RootFindingApp < matlab.apps.AppBase
% Properties that correspond to app components
properties (Access = public)
UIFigure matlab.ui.Figure
EquationLabel matlab.ui.control.Label
EquationEdit matlab.ui.control.EditField
MethodLabel matlab.ui.control.Label
MethodDropDown matlab.ui.control.DropDown
InitialLabel matlab.ui.control.Label
InitialEdit matlab.ui.control.NumericEditField
ComputeButton matlab.ui.control.Button
ResultLabel matlab.ui.control.Label
ResultEdit matlab.ui.control.EditField
end
% Callbacks that handle component events
methods (Access = private)
% Callback function for ComputeButton
function ComputeButtonPushed(app, event)
% Get equation and initial value
eqn = @(x) x^2 - exp(x);
x0 = app.InitialEdit.Value;
% Compute root using selected method
method = app.MethodDropDown.Value;
switch method
case 'Bisection'
[x, ~] = bisection(eqn, 0, 2, 1e-6);
case 'Fixed-Point Iteration'
[x, ~] = fixedpoint(eqn, x0, 1e-6, 50);
case 'Newton-Raphson'
[x, ~] = newtonraphson(eqn, x0, 1e-6, 50);
case 'fsolve'
x = fsolve(eqn, x0);
end
% Display result
app.ResultEdit.Value = num2str(x);
end
end
% App initialization and construction
methods (Access = private)
% Create UIFigure and components
function createComponents(app)
% Create UIFigure
app.UIFigure = uifigure;
app.UIFigure.Position = [100 100 640 480];
app.UIFigure.Name = 'Root-Finding App';
% Create EquationLabel
app.EquationLabel = uilabel(app.UIFigure);
app.EquationLabel.HorizontalAlignment = 'right';
app.EquationLabel.Position = [80 416 49 22];
app.EquationLabel.Text = 'Equation';
% Create EquationEdit
app.EquationEdit = uieditfield(app.UIFigure, 'text');
app.EquationEdit.Position = [138 416 407 22];
app.EquationEdit.Value = 'x^2 - exp(x)';
% Create MethodLabel
app.MethodLabel = uilabel(app.UIFigure);
app.MethodLabel.HorizontalAlignment = 'right';
app.MethodLabel.Position = [80 372 49 22];
app.MethodLabel.Text = 'Method';
% Create MethodDropDown
app.MethodDropDown = uidropdown(app.UIFigure);
app.MethodDropDown.Items = {'Bisection', 'Fixed-Point Iteration', 'Newton-Raphson', 'fsolve'};
app.MethodDropDown.Position = [138 372 407 22];
app.MethodDropDown.Value = 'Bisection';
% Create InitialLabel
app.InitialLabel = uilabel(app.UIFigure);
app.InitialLabel.HorizontalAlignment = 'right';
app.InitialLabel.Position = [80 328 49 22];
app.InitialLabel.Text = 'Initial';
% Create InitialEdit
app.InitialEdit = uieditfield(app.UIFigure, 'numeric');
app.InitialEdit.Position = [138 328 100 22];
app.InitialEdit.Value = 1;
% Create ComputeButton
app.ComputeButton = uibutton(app.UIFigure, 'push');
app.ComputeButton.ButtonPushedFcn = createCallbackFcn(app, @ComputeButtonPushed, true);
app.ComputeButton.Position = [367 328 100 22];
app.ComputeButton.Text = 'Compute';
% Create ResultLabel
app.ResultLabel = uilabel(app.UIFigure);
app.ResultLabel.HorizontalAlignment = 'right';
app.ResultLabel.Position = [80 284 49 22];
app.ResultLabel.Text = 'Result';
% Create ResultEdit
app.ResultEdit = uieditfield(app.UIFigure, 'text');
app.ResultEdit.Position = [138 284 407 22];
app.ResultEdit.Value = '';
end
end
% App creation and deletion
methods (Access = public)
% Construct app
function app = RootFindingApp
% Create UIFigure and components
createComponents(app)
% Register the app with App Designer
registerApp(app, app.UIFigure)
% Execute the startup function
runStartupFcn(app, @startupFcn)
if nargout == 0
clear app
end
end
% Code that executes before app deletion
function delete(app)
% Delete UIFigure when app is deleted
delete(app.UIFigure)
end
end
end
% Bisection method for root-finding
function [x, n] = bisection(f, a, b, tol)
fa = f(a);
fb = f(b);
if sign(fa) == sign(fb)
error('f(a) and f(b) have the same sign')
end
n = ceil(log2((b-a)/tol));
for k = 1:n
c = (a + b)/2;
fc = f(c);
if sign(fc) == sign(fa)
a = c;
fa = fc;
else
b = c;
fb = fc;
end
end
x = (a + b)/2;
end
% Fixed-point iteration for root-finding
function [x, n] = fixedpoint(g, x0, tol, maxiter)
x = x0;
for n = 1:maxiter
xprev = x;
x = g(xprev);
if abs(x - xprev) < tol
break
end
end
if n == maxiter
warning('Fixed-point iteration did not converge')
end
end
% Newton-Raphson method for root-finding
function [x, n] = newtonraphson(f, x0, tol, maxiter)
df = matlabFunction(diff(sym(f)));
x = x0;
for n = 1:maxiter
fx = f(x);
dfx = df(x);
xprev = x;
x = xprev - fx/dfx;
if abs(x - xprev) < tol
break
end
end
if n == maxiter
warning('Newton-Raphson method did not converge')
end
end
```
在 MATLAB 中运行该程序,然后输入方程 `x^2 - exp(x) = 0`,选择求根方法为 `Bisection`、`Fixed-Point Iteration`、`Newton-Raphson` 或 `fsolve` 中的一个,点击 `Compute` 按钮即可计算结果。
相关推荐
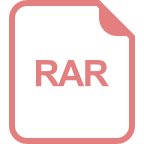
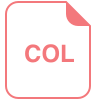
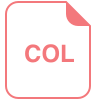











