解释def four_point_transform(image, pts): # 透视变换得到车牌小图 # rect = order_points(pts) rect = pts.astype('float32') (tl, tr, br, bl) = rect widthA = np.sqrt(((br[0] - bl[0]) ** 2) + ((br[1] - bl[1]) ** 2)) widthB = np.sqrt(((tr[0] - tl[0]) ** 2) + ((tr[1] - tl[1]) ** 2)) maxWidth = max(int(widthA), int(widthB)) heightA = np.sqrt(((tr[0] - br[0]) ** 2) + ((tr[1] - br[1]) ** 2)) heightB = np.sqrt(((tl[0] - bl[0]) ** 2) + ((tl[1] - bl[1]) ** 2)) maxHeight = max(int(heightA), int(heightB)) dst = np.array([ [0, 0], [maxWidth - 1, 0], [maxWidth - 1, maxHeight - 1], [0, maxHeight - 1]], dtype="float32") M = cv2.getPerspectiveTransform(rect, dst) warped = cv2.warpPerspective(image, M, (maxWidth, maxHeight)) return warped
时间: 2023-10-30 13:07:21 浏览: 198
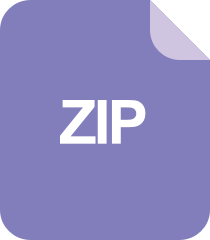
partial_transmit_sequence.zip_PAPR_papr pts_pts_pts PAPR_pts tec

这段代码实现的是对图像进行透视变换得到车牌小图的功能。具体解释如下:
- `image`:原始图像,即待进行透视变换的图像。
- `pts`:透视变换前后四个点的坐标,可以看作是变换矩阵的输入和输出。
- `rect = pts.astype('float32')`:将四个点的坐标转换为浮点数类型,方便后续计算。
- `(tl, tr, br, bl) = rect`:将转换后的四个点的坐标分别赋值给左上角、右上角、右下角、左下角,方便后续计算。
- `widthA`:计算右下角和左下角之间的距离。
- `widthB`:计算右上角和左上角之间的距离。
- `maxWidth`:取宽度距离的最大值,并向下取整。
- `heightA`:计算右上角和右下角之间的距离。
- `heightB`:计算左上角和左下角之间的距离。
- `maxHeight`:取高度距离的最大值,并向下取整。
- `dst`:变换后四个点的坐标,分别为左上角、右上角、右下角、左下角,并将其转换为浮点数类型。
- `M = cv2.getPerspectiveTransform(rect, dst)`:根据输入和输出的四个点的坐标计算透视变换矩阵。
- `warped = cv2.warpPerspective(image, M, (maxWidth, maxHeight))`:对原始图像进行透视变换,得到车牌小图。
- `return warped`:返回车牌小图。
综上所述,该段代码实现了透视变换得到车牌小图的功能,并返回变换后的图像。
阅读全文
相关推荐
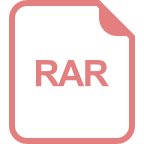
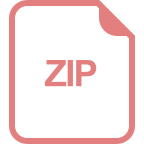












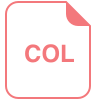
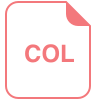

