用python写一个俄罗斯方块程序
时间: 2023-11-26 18:04:48 浏览: 44
好的,下面是一个简单的俄罗斯方块程序的代码,使用了 Pygame 游戏库:
```python
import pygame
import random
# 初始化 Pygame
pygame.init()
# 游戏区域的宽度和高度
WIDTH = 300
HEIGHT = 600
# 定义方块的大小
BLOCK_SIZE = 30
# 定义游戏区域的大小
GAME_AREA_WIDTH = BLOCK_SIZE * 10
GAME_AREA_HEIGHT = BLOCK_SIZE * 20
# 定义颜色
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
RED = (255, 0, 0)
GREEN = (0, 255, 0)
BLUE = (0, 0, 255)
YELLOW = (255, 255, 0)
# 创建游戏窗口
screen = pygame.display.set_mode((WIDTH, HEIGHT))
pygame.display.set_caption('俄罗斯方块')
# 定义字体
font = pygame.font.SysFont(None, 25)
# 定义方块的形状
SHAPE = [
[[1, 1, 1], [0, 1, 0]],
[[0, 2, 2], [2, 2, 0]],
[[3, 3, 0], [0, 3, 3]],
[[4, 0, 0], [4, 4, 4]],
[[0, 0, 5], [5, 5, 5]],
[[6, 6], [6, 6]],
[[7, 7, 7, 7]]
]
# 定义方块的颜色
COLORS = [RED, GREEN, BLUE, YELLOW]
# 定义方块的初始位置
INIT_POS = (3, 0)
# 定义游戏区域
game_area = [[0 for x in range(10)] for y in range(20)]
# 定义当前方块的位置和形状
current_shape = random.choice(SHAPE)
current_color = random.choice(COLORS)
current_pos = INIT_POS
# 定义下一个方块的位置和形状
next_shape = random.choice(SHAPE)
next_color = random.choice(COLORS)
next_pos = INIT_POS
# 定义计分和等级
score = 0
level = 1
# 定义游戏循环
while True:
# 处理游戏事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
# 如果当前方块向左移动会碰到游戏区域的边缘或者已经有方块了,就不能再向左移动了
if current_pos[0] == 0 or game_area[current_pos[1]][current_pos[0]-1] != 0:
pass
else:
current_pos = (current_pos[0]-1, current_pos[1])
elif event.key == pygame.K_RIGHT:
# 如果当前方块向右移动会碰到游戏区域的边缘或者已经有方块了,就不能再向右移动了
if current_pos[0] == 9 or game_area[current_pos[1]][current_pos[0]+1] != 0:
pass
else:
current_pos = (current_pos[0]+1, current_pos[1])
elif event.key == pygame.K_DOWN:
# 按下向下键加速方块下落
current_pos = (current_pos[0], current_pos[1]+1)
# 每隔一定时间让方块自动向下移动
if pygame.time.get_ticks() % (1000 // level) == 0:
# 如果当前方块到达游戏区域的底部或者下面已经有方块了,就不能再向下移动了,将当前方块落下
if current_pos[1] == 19 or game_area[current_pos[1]+1][current_pos[0]] != 0:
# 将当前方块落下,成为游戏区域的一部分
for i in range(2):
for j in range(3):
if current_shape[i][j] != 0:
game_area[current_pos[1]+i][current_pos[0]+j] = current_color
# 计算得分
rows_cleared = 0
for i in range(20):
if 0 not in game_area[i]:
rows_cleared += 1
for j in range(10):
game_area[i][j] = 0
for k in range(i, 0, -1):
game_area[k] = game_area[k-1]
game_area[0] = [0 for x in range(10)]
if rows_cleared == 1:
score += 100
elif rows_cleared == 2:
score += 300
elif rows_cleared == 3:
score += 500
elif rows_cleared == 4:
score += 800
# 显示得分和等级
text = font.render('Score: ' + str(score) + ' Level: ' + str(level), True, WHITE)
screen.blit(text, (10, 10))
# 将下一个方块设为当前方块
current_shape = next_shape
current_color = next_color
current_pos = INIT_POS
# 生成下一个方块
next_shape = random.choice(SHAPE)
next_color = random.choice(COLORS)
else:
current_pos = (current_pos[0], current_pos[1]+1)
# 绘制游戏区域
for i in range(20):
for j in range(10):
pygame.draw.rect(screen, BLACK, (j*BLOCK_SIZE, i*BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE), 1)
if game_area[i][j] != 0:
pygame.draw.rect(screen, COLORS[game_area[i][j]-1], (j*BLOCK_SIZE+1, i*BLOCK_SIZE+1, BLOCK_SIZE-2, BLOCK_SIZE-2))
# 绘制下一个方块
text = font.render('Next:', True, WHITE)
screen.blit(text, (210, 10))
for i in range(2):
for j in range(3):
if next_shape[i][j] != 0:
pygame.draw.rect(screen, COLORS[next_color-1], (220+j*BLOCK_SIZE, 50+i*BLOCK_SIZE, BLOCK_SIZE-2, BLOCK_SIZE-2))
# 绘制当前方块
for i in range(2):
for j in range(3):
if current_shape[i][j] != 0:
pygame.draw.rect(screen, COLORS[current_color-1], ((current_pos[0]+j)*BLOCK_SIZE+1, (current_pos[1]+i)*BLOCK_SIZE+1, BLOCK_SIZE-2, BLOCK_SIZE-2))
# 更新屏幕
pygame.display.update()
```
这个程序还有很多可以改进的地方,比如增加音效、增加游戏结束的判断等等。
阅读全文
相关推荐
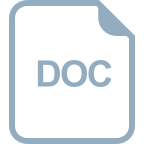
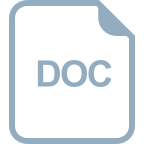
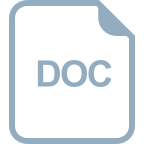















