用visual C++写代码:设有三个元素XYZ顺序进栈(进的过程中允许出栈),若出栈顺序为YZX,请用链栈实现这一过程。
时间: 2024-02-03 17:13:03 浏览: 130
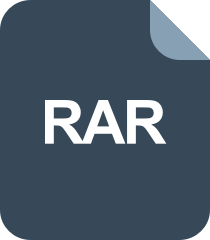
用C++实现栈的创建、输出、入栈、出栈

下面是用链栈实现该过程的代码示例:
```c++
#include <iostream>
using namespace std;
// 定义链栈结点结构体
struct ListNode {
char data; // 数据域
ListNode* next; // 指针域
};
// 初始化链栈
ListNode* InitStack() {
return NULL;
}
// 判断链栈是否为空
bool IsEmpty(ListNode* top) {
return (top == NULL);
}
// 元素入栈
ListNode* Push(ListNode* top, char x) {
ListNode* newNode = new ListNode;
newNode->data = x;
newNode->next = top;
top = newNode;
return top;
}
// 元素出栈
ListNode* Pop(ListNode* top, char& x) {
if (IsEmpty(top)) {
cout << "Stack is empty!" << endl;
return top;
}
ListNode* temp = top;
x = top->data;
top = top->next;
delete temp;
return top;
}
// 获取栈顶元素
char GetTop(ListNode* top) {
if (IsEmpty(top)) {
cout << "Stack is empty!" << endl;
return '\0';
}
return top->data;
}
int main() {
ListNode* top = InitStack(); // 初始化链栈
char x, y, z;
top = Push(top, 'X'); // X 入栈
top = Push(top, 'Y'); // Y 入栈
top = Pop(top, z); // X 出栈,z = 'X'
top = Push(top, 'Z'); // Z 入栈
top = Pop(top, y); // Y 出栈,y = 'Y'
top = Pop(top, x); // Z 出栈,x = 'Z'
cout << x << y << z << endl; // 输出 YZX
return 0;
}
```
以上代码中,每个元素进栈时都会被压入链栈的栈顶,因此出栈顺序为 YZX。
阅读全文
相关推荐
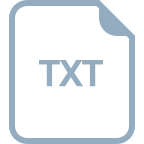















