务分配问题要求把n项任务分配给n个人,每个人完成每项任务的成本不同,求分配总成本最小的最优分配方案。用分支限界法求解任务分配问题,随机生成成本矩阵,矩阵中元素的取值范围为1~10,任务数目n取值为3,4,5,6,…。
时间: 2023-12-03 13:04:18 浏览: 186
任务分配问题可以使用分支限界法来求解。具体步骤如下:
1. 定义状态空间:每个状态包含一个已经分配了任务的人员列表,以及该列表下已经分配的任务的成本总和。
2. 定义扩展操作:对于每一个状态,扩展操作为将一个未分配任务分配给一个未被分配任务的人。
3. 定义限界函数:限界函数为已分配任务的成本总和加上剩余未分配任务的最小成本估计。
4. 用优先队列维护待扩展状态。
5. 不断取出队列中成本估计最小的状态进行扩展,直到找到一个可行解或者队列为空为止。
6. 返回解的成本。
以下是用 Python 实现任务分配问题的分支限界算法:
```python
import heapq
import random
# 随机生成任务成本矩阵
def generate_cost_matrix(n):
cost_matrix = []
for i in range(n):
row = []
for j in range(n):
row.append(random.randint(1, 10))
cost_matrix.append(row)
return cost_matrix
# 计算状态的限界值
def calculate_bound(state, cost_matrix):
# 已分配的任务成本总和
bound = sum(cost_matrix[i][state[i]] for i in range(len(state)))
# 剩余未分配任务的最小成本估计
for i in range(len(state), len(cost_matrix)):
min_cost = min(cost_matrix[i][j] for j in range(len(state)) if j not in state)
bound += min_cost
return bound
# 扩展状态
def expand_state(state, cost_matrix):
states = []
for i in range(len(state), len(cost_matrix)):
for j in range(len(state)):
if j not in state:
new_state = state + [j]
bound = calculate_bound(new_state, cost_matrix)
heapq.heappush(states, (bound, new_state))
return states
# 任务分配问题求解
def task_assignment(n):
cost_matrix = generate_cost_matrix(n)
initial_state = []
for i in range(n):
initial_state.append(-1)
queue = [(calculate_bound(initial_state, cost_matrix), initial_state)]
while queue:
(bound, state) = heapq.heappop(queue)
if len(state) == n:
# 找到可行解
return sum(cost_matrix[i][state[i]] for i in range(n))
for (new_bound, new_state) in expand_state(state, cost_matrix):
if new_bound < bound:
heapq.heappush(queue, (new_bound, new_state))
return None
# 测试
for n in range(3, 10):
cost = task_assignment(n)
print("n = {}, cost = {}".format(n, cost))
```
注意:这里使用 Python 的优先队列 heapq 来维护待扩展状态。在优先队列中,每个元素都是一个二元组 (bound, state),其中 bound 表示该状态的限界值,state 表示该状态。优先队列按照 bound 的大小进行排序,每次取出 bound 最小的状态进行扩展。同时,为了方便计算限界值,我们将未分配任务的人员编号设为 -1。
阅读全文
相关推荐
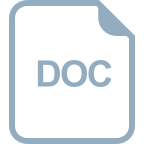
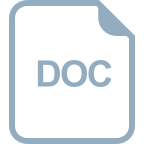
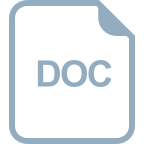


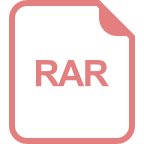
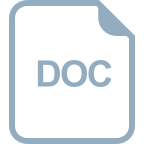
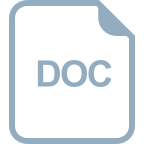
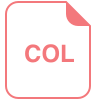









