stm32蓝牙小车代码
时间: 2023-09-05 09:09:54 浏览: 101
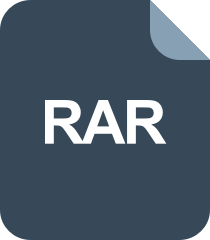
STM32智能小车蓝牙遥控(手机APP,速度可调)+测速基于库函数程序源代码.rar
以下是一个简单的STM32蓝牙小车代码示例。代码使用了HC-05蓝牙模块和L298N驱动模块,可以通过蓝牙控制小车的前进、后退、左转和右转。
```
#include "stm32f10x.h"
#define Bluetooth_RX_Pin GPIO_Pin_10
#define Bluetooth_TX_Pin GPIO_Pin_9
#define Bluetooth_GPIO GPIOA
#define Motor1_EN_Pin GPIO_Pin_0
#define Motor2_EN_Pin GPIO_Pin_1
#define Motor1_Pin1 GPIO_Pin_2
#define Motor1_Pin2 GPIO_Pin_3
#define Motor2_Pin1 GPIO_Pin_4
#define Motor2_Pin2 GPIO_Pin_5
#define Motor_GPIO GPIOB
USART_InitTypeDef USART_InitStructure;
GPIO_InitTypeDef GPIO_InitStructure;
void USART1_Init(void);
void GPIO_Configuration(void);
void Motor_Forward(void);
void Motor_Backward(void);
void Motor_Turn_Left(void);
void Motor_Turn_Right(void);
void Motor_Stop(void);
int main(void)
{
USART1_Init();
GPIO_Configuration();
Motor_Stop();
while(1)
{
if(USART_GetFlagStatus(USART1, USART_FLAG_RXNE) == SET)
{
char data = USART_ReceiveData(USART1);
switch(data)
{
case 'F':
Motor_Forward();
break;
case 'B':
Motor_Backward();
break;
case 'L':
Motor_Turn_Left();
break;
case 'R':
Motor_Turn_Right();
break;
case 'S':
Motor_Stop();
break;
default:
break;
}
}
}
}
void USART1_Init(void)
{
RCC_APB2PeriphClockCmd(RCC_APB2Periph_USART1 | RCC_APB2Periph_GPIOA, ENABLE);
USART_InitStructure.USART_BaudRate = 9600;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
USART_Init(USART1, &USART_InitStructure);
USART_Cmd(USART1, ENABLE);
}
void GPIO_Configuration(void)
{
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA | RCC_APB2Periph_GPIOB, ENABLE);
GPIO_InitStructure.GPIO_Pin = Bluetooth_RX_Pin;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_Init(Bluetooth_GPIO, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Pin = Bluetooth_TX_Pin;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_Init(Bluetooth_GPIO, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Pin = Motor1_EN_Pin | Motor2_EN_Pin | Motor1_Pin1 | Motor1_Pin2 | Motor2_Pin1 | Motor2_Pin2;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(Motor_GPIO, &GPIO_InitStructure);
}
void Motor_Forward(void)
{
GPIO_SetBits(Motor_GPIO, Motor1_Pin1 | Motor2_Pin1);
GPIO_ResetBits(Motor_GPIO, Motor1_Pin2 | Motor2_Pin2);
GPIO_SetBits(Motor_GPIO, Motor1_EN_Pin | Motor2_EN_Pin);
}
void Motor_Backward(void)
{
GPIO_SetBits(Motor_GPIO, Motor1_Pin2 | Motor2_Pin2);
GPIO_ResetBits(Motor_GPIO, Motor1_Pin1 | Motor2_Pin1);
GPIO_SetBits(Motor_GPIO, Motor1_EN_Pin | Motor2_EN_Pin);
}
void Motor_Turn_Left(void)
{
GPIO_SetBits(Motor_GPIO, Motor1_Pin2 | Motor2_Pin1);
GPIO_ResetBits(Motor_GPIO, Motor1_Pin1 | Motor2_Pin2);
GPIO_SetBits(Motor_GPIO, Motor1_EN_Pin | Motor2_EN_Pin);
}
void Motor_Turn_Right(void)
{
GPIO_SetBits(Motor_GPIO, Motor1_Pin1 | Motor2_Pin2);
GPIO_ResetBits(Motor_GPIO, Motor1_Pin2 | Motor2_Pin1);
GPIO_SetBits(Motor_GPIO, Motor1_EN_Pin | Motor2_EN_Pin);
}
void Motor_Stop(void)
{
GPIO_ResetBits(Motor_GPIO, Motor1_Pin1 | Motor1_Pin2 | Motor2_Pin1 | Motor2_Pin2);
GPIO_ResetBits(Motor_GPIO, Motor1_EN_Pin | Motor2_EN_Pin);
}
```
注意:这只是一个示例代码,需要根据具体的硬件电路和需求进行修改。
阅读全文
相关推荐
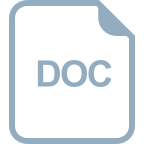
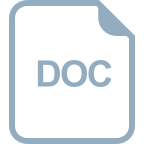
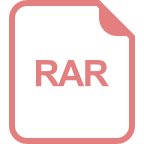
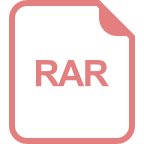



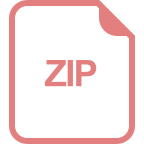
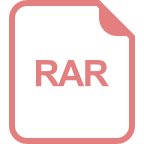
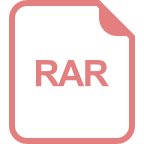
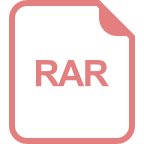
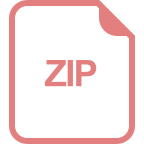





