最大团问题python
时间: 2023-10-14 13:25:58 浏览: 43
最大团问题是一个经典的计算机科学问题,它的目标是在一个给定的无向图中找到一个包含最多顶点的完全子图(即任意两个顶点之间都有一条边相连)。在Python中,可以使用networkx库来解决最大团问题。下面是一个示例代码:
```python
import networkx as nx
from networkx.algorithms.approximation import max_clique
# 创建一个无向图
G = nx.Graph()
# 添加顶点
G.add_nodes_from([1, 2, 3, 4, 5])
# 添加边
G.add_edges_from([(1, 2), (1, 3), (2, 3), (3, 4), (4, 5), (5, 1)])
# 计算最大团
max_clique = max_clique(G)
# 输出结果
print("最大团为:", max_clique)
```
在这个示例中,我们首先创建了一个无向图,然后使用`max_clique`函数计算最大团。最后,我们输出了最大团的结果。
相关问题
最大团问题 python
最大团问题是图论中的经典问题,指在一个无向图中找到一个最大的完全子图(即一个团),其中每两个顶点都有边相连。下面是一个用Python解决最大团问题的示例代码:
```python
import itertools
def max_clique(graph):
"""
寻找最大团的函数
:param graph: 无向图,用邻接矩阵表示
:return: 最大团中的节点列表
"""
n = len(graph)
nodes = set(range(n))
max_clique = set()
# 从所有节点集合中找到最大团
for size in range(n, 0, -1):
for subset in itertools.combinations(nodes, size):
if all(graph[i][j] for i, j in itertools.combinations(subset, 2)):
max_clique = subset
return list(max_clique)
```
该函数使用了Python中的itertools库,其中的combinations函数可以生成所有长度为size的子集。函数通过不断从大到小枚举子集的大小,判断子集是否为团,最终找到最大的团。
最大团问题算法python
根据提供的引用内容,最大团问题是一个NP问题,要求使用Python编写算法,并且需要记录中间结果。以下是一个使用回溯算法解决最大团问题的Python代码示例:
```python
def is_clique(graph, vertices):
for i in range(len(vertices)):
for j in range(i+1, len(vertices)):
if not graph[vertices[i]][vertices[j]]:
return False
return True
def max_clique(graph, vertices, max_cliques):
if len(vertices) == 0:
max_cliques.append(vertices)
return
for v in vertices:
neighbors = [n for n in vertices if graph[v][n]]
max_clique(graph, neighbors, max_cliques)
def find_max_cliques(graph):
max_cliques = []
vertices = list(range(len(graph)))
max_clique(graph, vertices, max_cliques)
return max_cliques
# 示例使用
graph = [[False, True, True, False],
[True, False, True, True],
[True, True, False, True],
[False, True, True, False]]
cliques = find_max_cliques(graph)
for clique in cliques:
print(clique)
```
这段代码使用了回溯算法来找到图中的最大团。首先,`is_clique`函数用于判断给定的顶点集合是否构成一个团。然后,`max_clique`函数使用递归的方式来找到所有的最大团,并将结果存储在`max_cliques`列表中。最后,`find_max_cliques`函数调用`max_clique`函数来找到图中的所有最大团,并返回结果。
相关推荐
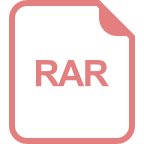
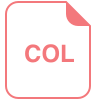











