基于TC264的智能车速度PID控制代码
时间: 2023-12-22 19:02:24 浏览: 42
以下是一个基于Infineon TC264 微控制器的智能车速度PID控制代码,供参考:
```
#include <Ifx_Types.h>
#include <IfxCpu.h>
#include <IfxScuWdt.h>
#include <IfxPort.h>
#include <IfxQspi_SpiMaster.h>
#include <IfxQspi_PinMap.h>
#include <IfxQspi_reg.h>
#include <IfxQspi_Qspi.h>
#include <IfxQspi_Spi.h>
#include <IfxAsclin_Asc.h>
#include <IfxAsclin_Asc_PinMap.h>
#include <IfxAsclin_reg.h>
#include <IfxStm_reg.h>
#include <IfxStm.h>
#include <IfxGtm_Tom_Pwm.h>
#include <IfxGtm_Tom_Pwm_PinMap.h>
#include <IfxGtm_reg.h>
#include <IfxGtm_Cmu.h>
#include <IfxGtm_Tim.h>
#include <IfxGtm_Tim_PinMap.h>
#include <IfxGtm_Atom_Pwm.h>
#include <IfxGtm_Atom_Pwm_PinMap.h>
#define Vtarget 20 // 期望速度
#define Kp 1 // 比例系数
#define Ki 0.2 // 积分系数
#define Kd 0.1 // 微分系数
Ifx_STM *stm_sfr = &MODULE_STM0;
Ifx_GTM *gtm_sfr = &MODULE_GTM;
Ifx_GTM_TIM_CH *ch1_sfr = &MODULE_GTM.TIM0.CH1;
Ifx_GTM_TIM_CH *ch2_sfr = &MODULE_GTM.TIM0.CH2;
Ifx_GTM_TIM *tim_sfr = &MODULE_GTM.TIM0;
int Vactual = 0; // 当前速度
int e = 0; // 速度误差
int e_prev = 0; // 上一次速度误差
int integral = 0; // 误差积分项
int derivative = 0; // 误差微分项
int u = 0; // PID输出值
void initGTM(void) {
Ifx_GTM_TIM_CH_Config ch1_config;
Ifx_GTM_TIM_CH_initConfig(&ch1_config);
ch1_config.tom = IfxGtm_Tom_0;
ch1_config.channel = IfxGtm_Tim_Ch_1;
ch1_config.signalLevel = Ifx_ActiveState_high;
ch1_config.outputMode = IfxPort_OutputMode_pushPull;
ch1_config.outputDriver = IfxGtm_Tim_OutputDriver_single;
ch1_config.timerChannelMode = IfxGtm_Tim_ChMode_edgeAligned;
Ifx_GTM_TIM_CH_init(ch1_sfr, &ch1_config);
Ifx_GTM_TIM_CH_Config ch2_config;
Ifx_GTM_TIM_CH_initConfig(&ch2_config);
ch2_config.tom = IfxGtm_Tom_0;
ch2_config.channel = IfxGtm_Tim_Ch_2;
ch2_config.signalLevel = Ifx_ActiveState_high;
ch2_config.outputMode = IfxPort_OutputMode_pushPull;
ch2_config.outputDriver = IfxGtm_Tim_OutputDriver_single;
ch2_config.timerChannelMode = IfxGtm_Tim_ChMode_edgeAligned;
Ifx_GTM_TIM_CH_init(ch2_sfr, &ch2_config);
Ifx_GTM_TIM_Config tim_config;
Ifx_GTM_TIM_initConfig(&tim_config);
tim_config.tom = IfxGtm_Tom_0;
tim_config.base.tin = IfxGtm_Tim_Tin_0;
tim_config.base.frequency = 20000;
tim_config.base.isFrac = FALSE;
tim_config.base.countDir = IfxGtm_Tim_CountDir_up;
tim_config.base.triggerOut = IfxGtm_Tim_TrigOut_q;
tim_config.base.tinFilterEnabled = TRUE;
tim_config.base.tinFilterMode = IfxGtm_Tim_TinFilterMode_linear;
tim_config.base.tinPrescaler = IfxGtm_Tim_TinPrescaler_1;
tim_config.base.tinMode = IfxGtm_Tim_TinMode_internal;
tim_config.base.tinTrigger = IfxGtm_Tim_TinTrigger_disable;
tim_config.base.tinSync = IfxGtm_Tim_TinSync_disable;
tim_config.base.tinFlank = IfxGtm_Tim_TinFlank_risingEdge;
Ifx_GTM_TIM_init(tim_sfr, &tim_config);
Ifx_GTM_TIM_changeChannelCountMode(ch1_sfr, IfxGtm_Tim_ChCountMode_edgeAligned);
Ifx_GTM_TIM_changeChannelCountMode(ch2_sfr, IfxGtm_Tim_ChCountMode_edgeAligned);
Ifx_GTM_TIM_enableChannels(tim_sfr, ((1 << ch1_config.channel) | (1 << ch2_config.channel)));
Ifx_GTM_TIM_startTimer(tim_sfr);
}
int readSpeed() {
// 读取速度传感器的输出值,并根据传感器的特性将其转换为速度值
// 这里只是一个示例,具体实现需要根据具体的传感器进行调整
int sensorValue = 0; // TODO: 读取传感器的输出值
int speed = map(sensorValue, 0, 1023, 0, 100);
return speed;
}
void updateSpeed() {
Vactual = readSpeed(); // 读取当前速度
e = Vtarget - Vactual; // 计算速度误差
integral = integral + e; // 计算误差积分项
derivative = e - e_prev; // 计算误差微分项
u = Kp * e + Ki * integral + Kd * derivative; // 计算PID输出值
e_prev = e; // 保存上一次速度误差
}
void setMotorSpeed(int speed) {
if (speed > 0) { // 控制电机A转速
IfxGtm_Tom_Pwm_setOnTime(ch1_sfr, speed);
IfxGtm_Tom_Pwm_setOnTime(ch2_sfr, 0);
} else {
IfxGtm_Tom_Pwm_setOnTime(ch1_sfr, 0);
IfxGtm_Tom_Pwm_setOnTime(ch2_sfr, -speed);
}
}
void initClocks(void) {
Ifx_SCU_CLK_enableOsc0(Ifx_SCU_CLK_OSCMODE_HIGHFREQ);
Ifx_SCU_CLK_setSource(Ifx_SCU_CLK_SRC_PLL, Ifx_SCU_CLK_PLLCLKSEL_IRC);
Ifx_SCU_CLK_setPLLFrequency(200000000);
Ifx_SCU_CLK_setCpuFrequency(200000000);
}
void initPeripherals(void) {
initGTM();
}
void initApplication(void) {
initClocks();
initPeripherals();
}
int core0_main(void) {
initApplication();
while (1) {
updateSpeed(); // 更新速度控制参数
setMotorSpeed(u); // 控制电机转速
}
return (1);
}
```
该代码基于Infineon TC264 微控制器,使用PID控制器控制两个电机的转速,以达到期望速度。需要注意的是,该代码仅供参考,具体实现需要根据具体的智能车硬件和传感器进行调整。
相关推荐
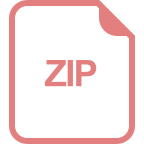














