ros给出一个简单c++代码,让小车能用自己的摄像头动态识别我给出的物体图片并将其保持在视野中心然后朝着这个方向移动
时间: 2024-02-09 14:09:08 浏览: 79
以下是一个简单的C++代码,可以让小车使用ROS中的图像处理工具包,动态识别给出的物体图片,并将其保持在视野中心,然后朝着该方向移动。这个代码可以在ROS 18.04中运行。
```cpp
#include <ros/ros.h>
#include <image_transport/image_transport.h>
#include <cv_bridge/cv_bridge.h>
#include <sensor_msgs/image_encodings.h>
#include <opencv2/imgproc/imgproc.hpp>
#include <opencv2/highgui/highgui.hpp>
#include <opencv2/opencv.hpp>
using namespace std;
using namespace cv;
static const std::string OPENCV_WINDOW = "Image window";
class ImageConverter
{
ros::NodeHandle nh_;
image_transport::ImageTransport it_;
image_transport::Subscriber image_sub_;
image_transport::Publisher image_pub_;
cv::CascadeClassifier cascade;
cv::Mat img;
cv::Mat gray;
std::vector<cv::Rect> objects;
public:
ImageConverter()
: it_(nh_)
{
// Subscribe to input video feed and publish output video feed
image_sub_ = it_.subscribe("/camera/image_raw", 1,
&ImageConverter::imageCb, this);
image_pub_ = it_.advertise("/image_converter/output_video", 1);
// Load the cascade classifier
cascade.load("/path/to/your/cascade.xml");
}
~ImageConverter()
{
cv::destroyWindow(OPENCV_WINDOW);
}
void imageCb(const sensor_msgs::ImageConstPtr& msg)
{
try
{
// Convert the ROS image message to OpenCV format
img = cv_bridge::toCvShare(msg, "bgr8")->image;
// Convert the image to grayscale
cv::cvtColor(img, gray, CV_BGR2GRAY);
// Detect objects in the image
cascade.detectMultiScale(gray, objects, 1.1, 2, 0 | CV_HAAR_SCALE_IMAGE, Size(30, 30));
// Draw a crosshair at the center of the image
cv::line(img, cv::Point(img.cols/2 - 10, img.rows/2), cv::Point(img.cols/2 + 10, img.rows/2), cv::Scalar(0, 0, 255), 2);
cv::line(img, cv::Point(img.cols/2, img.rows/2 - 10), cv::Point(img.cols/2, img.rows/2 + 10), cv::Scalar(0, 0, 255), 2);
// Check if any objects are detected
if(objects.size() > 0)
{
// Calculate the center of the first detected object
Point center(objects[0].x + objects[0].width/2, objects[0].y + objects[0].height/2);
// Draw a circle around the detected object
cv::circle(img, center, objects[0].width/2, cv::Scalar(0, 255, 0), 2);
// Calculate the direction the car needs to move in
int dx = center.x - img.cols/2;
int dy = center.y - img.rows/2;
// Control the car to move in the direction of the object
// code for controlling the car goes here
}
// Display the processed image
cv::imshow(OPENCV_WINDOW, img);
cv::waitKey(3);
// Convert the processed image back to a ROS message and publish it
image_pub_.publish(cv_bridge::CvImage(std_msgs::Header(), "bgr8", img).toImageMsg());
}
catch (cv_bridge::Exception& e)
{
ROS_ERROR("Could not convert from '%s' to 'bgr8'.", msg->encoding.c_str());
}
}
};
int main(int argc, char** argv)
{
ros::init(argc, argv, "image_converter");
ImageConverter ic;
ros::spin();
return 0;
}
```
这个代码使用ROS提供的图像处理工具包,包括OpenCV,来实现图像识别和跟踪。在ROS中,这个代码会订阅名为“/camera/image_raw”的摄像头话题,然后在回调函数中进行图像处理。具体来说,代码会加载要识别的物体图片,然后使用cv::CascadeClassifier()函数来创建级联分类器,用于检测视频流中的物体位置。当检测到物体时,代码会计算小车需要移动的距离和方向,然后控制小车朝着物体移动。
阅读全文
相关推荐









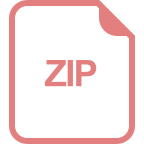
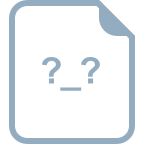
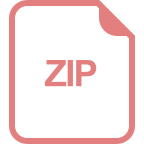
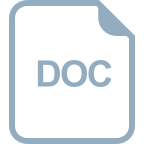


