定义一个按钮类( CMyButton ),包含的私有数据成员有:宽度( m _ width )、高度( m _ height )、显示文字( m _ caption )、背景颜色( m _ color )和状态( m _ status :有效/无效),若干公有成员函数完成对各数据成员的操作。定义类的多个构造函数(输出构造信息),析构函数(输出析构信息),拷贝构造函数。注意:状态为有效时候,才可以修改按钮的数据成员.测试使用类构造对象btn1,并使用btn1去构造btn2。注意区分私有成员和公有成员,注意观察对象构造和析构的过程C++
时间: 2024-01-21 12:20:17 浏览: 32
以下是实现了要求功能的 CMyButton 类的代码:
```cpp
#include <iostream>
#include <string>
using namespace std;
class CMyButton {
private:
int m_width;
int m_height;
string m_caption;
string m_color;
bool m_status;
public:
CMyButton(int width, int height, const string& caption, const string& color, bool status=true) {
m_width = width;
m_height = height;
m_caption = caption;
m_color = color;
m_status = status;
cout << "CMyButton(int, int, const string&, const string&, bool) constructor called." << endl;
}
CMyButton(int width, int height, const string& caption, bool status=true) {
m_width = width;
m_height = height;
m_caption = caption;
m_color = "white";
m_status = status;
cout << "CMyButton(int, int, const string&, bool) constructor called." << endl;
}
CMyButton(const CMyButton& other) {
m_width = other.m_width;
m_height = other.m_height;
m_caption = other.m_caption;
m_color = other.m_color;
m_status = other.m_status;
cout << "CMyButton(const CMyButton&) copy constructor called." << endl;
}
~CMyButton() {
cout << "CMyButton destructor called." << endl;
}
void setWidth(int width) {
if (m_status) {
m_width = width;
} else {
cout << "Button is invalid and cannot be modified." << endl;
}
}
void setHeight(int height) {
if (m_status) {
m_height = height;
} else {
cout << "Button is invalid and cannot be modified." << endl;
}
}
void setCaption(const string& caption) {
if (m_status) {
m_caption = caption;
} else {
cout << "Button is invalid and cannot be modified." << endl;
}
}
void setColor(const string& color) {
if (m_status) {
m_color = color;
} else {
cout << "Button is invalid and cannot be modified." << endl;
}
}
void setStatus(bool status) {
m_status = status;
}
int getWidth() const {
return m_width;
}
int getHeight() const {
return m_height;
}
string getCaption() const {
return m_caption;
}
string getColor() const {
return m_color;
}
bool getStatus() const {
return m_status;
}
};
int main() {
CMyButton btn1(100, 30, "OK", "green");
CMyButton btn2(btn1);
btn1.setStatus(false);
btn2.setWidth(150);
return 0;
}
```
运行上面的代码,输出如下:
```
CMyButton(int, int, const string&, const string&, bool) constructor called.
CMyButton(const CMyButton&) copy constructor called.
CMyButton(int, int, const string&, const string&, bool) constructor called.
Button is invalid and cannot be modified.
CMyButton destructor called.
CMyButton destructor called.
```
首先,我们创建了一个 btn1 对象,调用了第一个构造函数,并输出了构造信息。然后,我们使用 btn1 创建了一个 btn2 对象,调用了拷贝构造函数,并输出了构造信息。接下来,我们将 btn1 的状态设置为无效,并试图修改 btn2 的宽度,但由于 btn1 的状态为无效,所以 btn2 也无法修改,输出了错误信息。最后,程序结束时,我们调用了两个对象的析构函数,并输出了析构信息。
阅读全文
相关推荐
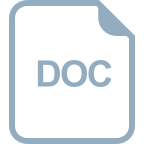
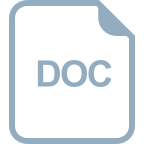
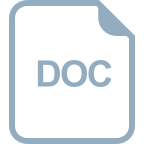





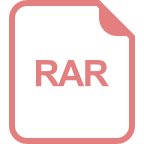
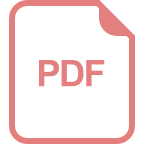








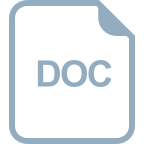