写一个光追里射线和三角形求交的代码
时间: 2024-05-12 14:16:16 浏览: 101
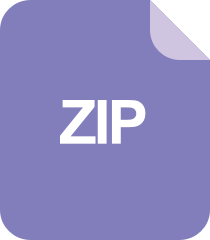
Triangle/Ray Intersection:快速矢量化三角形/射线交叉算法-matlab开发
以下是一个基于C++的简单代码示例:
```
#include <iostream>
#include <vector>
#include <cmath>
using namespace std;
struct Vector3 {
float x, y, z;
Vector3 operator+(const Vector3& v) const {
return { x + v.x, y + v.y, z + v.z };
}
Vector3 operator-(const Vector3& v) const {
return { x - v.x, y - v.y, z - v.z };
}
Vector3 operator*(float f) const {
return { x * f, y * f, z * f };
}
Vector3 cross(const Vector3& v) const {
return { y * v.z - z * v.y, z * v.x - x * v.z, x * v.y - y * v.x };
}
float dot(const Vector3& v) const {
return x * v.x + y * v.y + z * v.z;
}
float length() const {
return sqrtf(x * x + y * y + z * z);
}
Vector3 normalize() const {
float len = length();
return { x / len, y / len, z / len };
}
};
struct Ray {
Vector3 origin;
Vector3 direction;
};
struct Triangle {
Vector3 v0, v1, v2;
Vector3 normal() const {
return (v1 - v0).cross(v2 - v0).normalize();
}
};
// 判断射线和三角形是否相交
bool intersectRayTriangle(const Ray& ray, const Triangle& triangle, float& t) {
Vector3 e1 = triangle.v1 - triangle.v0;
Vector3 e2 = triangle.v2 - triangle.v0;
Vector3 h = ray.direction.cross(e2);
float a = e1.dot(h);
if (a > -1e-6f && a < 1e-6f) {
return false;
}
float f = 1.0f / a;
Vector3 s = ray.origin - triangle.v0;
float u = f * s.dot(h);
if (u < 0.0f || u > 1.0f) {
return false;
}
Vector3 q = s.cross(e1);
float v = f * ray.direction.dot(q);
if (v < 0.0f || u + v > 1.0f) {
return false;
}
t = f * e2.dot(q);
return t > 1e-6f;
}
int main() {
// 构建射线
Ray ray = { { 0.0f, 0.0f, 0.0f }, { 1.0f, 1.0f, 1.0f }.normalize() };
// 构建三角形
Triangle triangle = { { 1.0f, 0.0f, 0.0f }, { 0.0f, 1.0f, 0.0f }, { 0.0f, 0.0f, 1.0f } };
// 求交
float t;
if (intersectRayTriangle(ray, triangle, t)) {
cout << "Intersection at t = " << t << endl;
} else {
cout << "No intersection" << endl;
}
return 0;
}
```
在这个示例中,我们定义了三个结构体:`Vector3`表示三维向量,`Ray`表示射线,`Triangle`表示三角形。接着,我们实现了一个名为`intersectRayTriangle`的函数来判断射线和三角形是否相交,如果相交,函数将返回`true`并返回相交点的参数`t`,否则返回`false`。
函数的实现基于Möller-Trumbore算法,该算法是一种高效的射线三角形求交算法。
最后,在`main`函数中,我们构建了一个射线和一个三角形,并使用`intersectRayTriangle`函数来求交。如果有交点,我们输出参数`t`,否则输出"No intersection"。
阅读全文
相关推荐
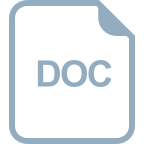
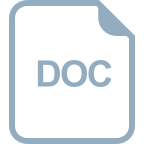
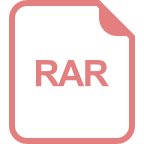
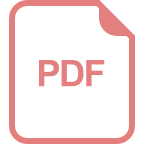
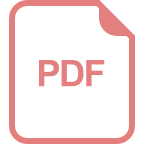
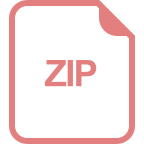
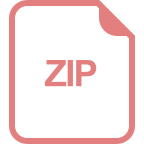
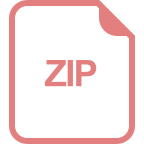
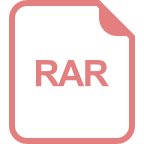
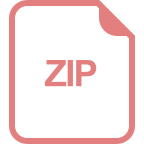
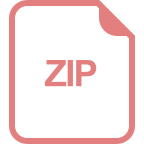
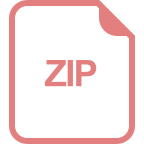
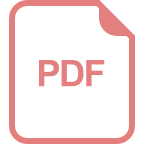
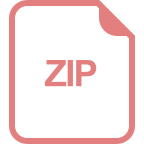
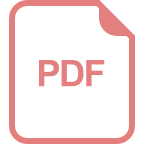
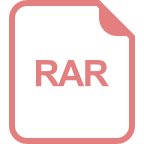
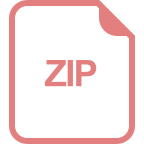
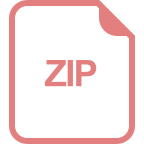