模拟退火求解att48.tsp
时间: 2023-10-05 22:04:23 浏览: 59
TSP(Traveling Salesman Problem,旅行商问题)是一个经典的组合优化问题,目标是找到一条经过所有城市的最短路径。att48.tsp 是一个包含48个城市的 TSP 问题,我们可以使用模拟退火算法来求解该问题。
首先,我们需要读取 att48.tsp 文件并计算出任意两个城市之间的距离。可以使用以下代码实现:
``` python
import math
# 读取 TSP 文件并计算城市之间的距离
def read_tsp_file(filename):
with open(filename, 'r') as f:
lines = f.readlines()
# 读取城市坐标
cities = []
for line in lines:
if line[0].isdigit():
city_info = line.split()
city_id = int(city_info[0])
x = float(city_info[1])
y = float(city_info[2])
cities.append((city_id, x, y))
# 计算城市之间的距离
distances = {}
for i in range(len(cities)):
for j in range(i+1, len(cities)):
city1 = cities[i]
city2 = cities[j]
distance = math.sqrt((city1[1]-city2[1])**2 + (city1[2]-city2[2])**2)
distances[(city1[0], city2[0])] = distance
distances[(city2[0], city1[0])] = distance
return cities, distances
# 测试
cities, distances = read_tsp_file('att48.tsp')
print("城市坐标:", cities)
print("城市之间的距离:", distances)
```
接下来,我们可以实现一个 TSP 的目标函数,即计算给定路径的总距离:
``` python
# 计算路径的总距离
def total_distance(path, distances):
distance = 0
for i in range(len(path)-1):
distance += distances[(path[i], path[i+1])]
distance += distances[(path[-1], path[0])]
return distance
```
然后,我们可以使用模拟退火算法来求解 TSP 问题。以下是一个求解 att48.tsp 的模拟退火算法的代码实现:
``` python
import random
import math
# 模拟退火算法
def simulated_annealing_tsp(distances, n_iterations, initial_temp, temp_reduction):
# 随机生成一个初始解
current_path = list(distances.keys())
random.shuffle(current_path)
current_fitness = total_distance(current_path, distances)
# 记录温度
current_temp = initial_temp
# 迭代优化
for i in range(n_iterations):
# 随机生成一个新解
candidate_path = list(current_path)
index1, index2 = sorted(random.sample(range(len(candidate_path)), 2))
candidate_path[index1:index2+1] = reversed(candidate_path[index1:index2+1])
# 计算新解的适应度
candidate_fitness = total_distance(candidate_path, distances)
# 计算适应度提高的程度
delta_fitness = candidate_fitness - current_fitness
# 判断是否接受新解
if delta_fitness < 0 or math.exp(-delta_fitness / current_temp) > random.random():
current_path = candidate_path
current_fitness = candidate_fitness
# 降低温度
current_temp *= temp_reduction
return current_path, current_fitness
# 测试
cities, distances = read_tsp_file('att48.tsp')
best_path, best_fitness = simulated_annealing_tsp(distances, 10000, 100, 0.95)
print("最优路径:", best_path)
print("最优路径的总距离:", best_fitness)
```
在该代码中,我们随机生成一个初始解,然后在每次迭代中随机生成一个新解,并根据一定的概率接受该解或者继续保留当前解。随着迭代次数的增加和温度的降低,算法最终收敛到一个局部最优解。我们最终得到的最优路径和总距离都可以通过调用 `simulated_annealing_tsp` 函数来获得。
相关推荐















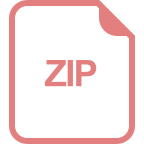
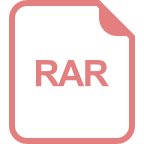