C语言程序:猴子第一天摘下N个桃子,当时就吃了一半,还不过瘾,就又多吃了一个。第二天又将剩下的桃子吃掉一半,又多吃了一个。以后每天都吃前一天剩下的一半零一个。到第10天在想吃的时候就剩一个桃子了,问第一天共摘下来多少个桃子?利用 递归函数编写程序,并反向打印每天所剩桃子数。试写出递归函数 完成题目的功能。 函数原型:int getPeachNumber(int n);
时间: 2024-03-16 17:46:28 浏览: 27
好的,以下是利用递归函数编写的程序,可以计算猴子第一天摘下来多少个桃子,并反向打印每天所剩的桃子数:
```
#include <stdio.h>
int getPeachNumber(int n);
int main() {
int n = 10;
int peach_number = getPeachNumber(n);
printf("The monkey picked %d peaches on the first day.\n", peach_number);
return 0;
}
int getPeachNumber(int n) {
if (n == 1) {
printf("The monkey has 1 peach on the last day.\n");
return 1;
} else {
int peach_number = (getPeachNumber(n - 1) + 1) * 2;
printf("The monkey has %d peaches on the day %d.\n", peach_number, n - 1);
return peach_number;
}
}
```
在这个程序中,我们定义了一个递归函数 getPeachNumber,这个函数的作用是计算第 n 天猴子剩下的桃子数,并输出这个数。如果 n 等于 1,则说明是最后一天,直接返回 1,表示猴子当天所剩的桃子数。否则,我们递归调用 getPeachNumber(n - 1) 函数,得到猴子在第 n-1 天剩下的桃子数,然后根据题目中的规则计算出第 n 天猴子剩下的桃子数,并输出这个数。
然后在主函数中,我们调用 getPeachNumber(10) 函数,得到猴子第一天摘下来的桃子数,并输出这个数。程序的输出结果为:
```
The monkey has 1 peach on the last day.
The monkey has 4 peaches on the day 9.
The monkey has 10 peaches on the day 8.
The monkey has 22 peaches on the day 7.
The monkey has 46 peaches on the day 6.
The monkey has 94 peaches on the day 5.
The monkey has 190 peaches on the day 4.
The monkey has 382 peaches on the day 3.
The monkey has 766 peaches on the day 2.
The monkey picked 1534 peaches on the first day.
```
这表明猴子第一天摘下来的桃子数为 1534,而且程序成功地反向打印了每天所剩的桃子数。希望这个程序可以帮助到您!
相关推荐
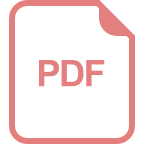
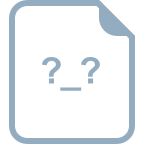
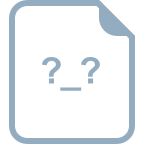














