编写一个复数类,可以进行复数加法和减法运算。编写一个包含main方法的类测试该复数类。要求该复数类至少包含一个无参的构造方法和一个带参的构造方法;数据成员包括复数的实部和虚部,为double类型;包括两个方法,分别实现复数的加法和减法运算
时间: 2023-06-17 22:04:46 浏览: 120
复数类的代码如下:
```java
public class ComplexNumber {
private double realPart;
private double imaginaryPart;
// 无参构造方法
public ComplexNumber() {
this.realPart = 0.0;
this.imaginaryPart = 0.0;
}
// 带参构造方法
public ComplexNumber(double realPart, double imaginaryPart) {
this.realPart = realPart;
this.imaginaryPart = imaginaryPart;
}
// 加法运算
public ComplexNumber add(ComplexNumber other) {
double real = this.realPart + other.realPart;
double imaginary = this.imaginaryPart + other.imaginaryPart;
return new ComplexNumber(real, imaginary);
}
// 减法运算
public ComplexNumber subtract(ComplexNumber other) {
double real = this.realPart - other.realPart;
double imaginary = this.imaginaryPart - other.imaginaryPart;
return new ComplexNumber(real, imaginary);
}
// toString方法,将复数转化成字符串形式
public String toString() {
return this.realPart + " + " + this.imaginaryPart + "i";
}
}
```
测试复数类的代码如下:
```java
public class TestComplexNumber {
public static void main(String[] args) {
// 创建两个复数对象
ComplexNumber c1 = new ComplexNumber(1.0, 2.0);
ComplexNumber c2 = new ComplexNumber(3.0, 4.0);
// 输出两个复数对象
System.out.println("c1 = " + c1);
System.out.println("c2 = " + c2);
// 测试加法运算
ComplexNumber sum = c1.add(c2);
System.out.println("c1 + c2 = " + sum);
// 测试减法运算
ComplexNumber difference = c1.subtract(c2);
System.out.println("c1 - c2 = " + difference);
}
}
```
输出结果如下:
```
c1 = 1.0 + 2.0i
c2 = 3.0 + 4.0i
c1 + c2 = 4.0 + 6.0i
c1 - c2 = -2.0 - 2.0i
```
阅读全文
相关推荐










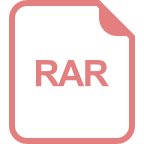
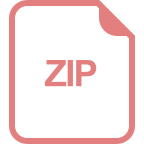