c语言实现仿射密码
时间: 2023-08-13 12:19:02 浏览: 130
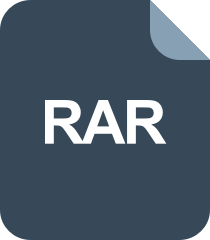
实现仿射密码

以下是使用C语言实现仿射密码的示例代码:
```c
#include <stdio.h>
#include <string.h>
#include <ctype.h>
int gcd(int a, int b) {
if (b == 0) {
return a;
} else {
return gcd(b, a % b);
}
}
int mod_inverse(int a, int m) {
int x0 = 1, x1 = 0, y0 = 0, y1 = 1;
int r0 = a, r1 = m, q, r, x, y;
while (r1 != 0) {
q = r0 / r1;
r = r0 % r1;
x = x0 - q * x1;
y = y0 - q * y1;
r0 = r1;
r1 = r;
x0 = x1;
x1 = x;
y0 = y1;
y1 = y;
}
if (r0 > 1) {
return -1;
}
if (x0 < 0) {
x0 += m;
}
return x0;
}
int is_valid_key(int k, int m) {
return gcd(k, m) == 1;
}
void affine_encrypt(char *plaintext, int k, int b, char *ciphertext) {
int i, x, y;
for (i = 0; i < strlen(plaintext); i++) {
if (isalpha(plaintext[i])) {
x = toupper(plaintext[i]) - 'A';
y = (k * x + b) % 26;
ciphertext[i] = 'A' + y;
} else {
ciphertext[i] = plaintext[i];
}
}
ciphertext[i] = '\0';
}
int main() {
char plaintext[100], ciphertext[100];
int k, b;
printf("请输入明文:");
gets(plaintext);
printf("请输入密钥k和b:");
scanf("%d%d", &k, &b);
if (!is_valid_key(k, 26)) {
printf("无效的密钥!\n");
return 0;
}
affine_encrypt(plaintext, k, b, ciphertext);
printf("密文为:%s\n", ciphertext);
return 0;
}
```
这个代码中,我们使用了两个辅助函数gcd和mod_inverse,分别用于求最大公约数和模反元素。is_valid_key函数用于检查密钥是否有效,即要求密钥k与模数26互质。affine_encrypt函数用于对明文进行加密,具体实现和前面介绍的算法类似。需要注意的是,这个程序只能处理大小写字母,如果要处理数字或其他字符,需要进行相应的修改。
阅读全文
相关推荐




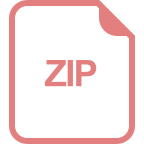
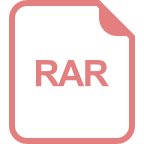
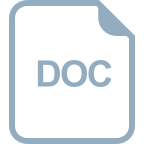





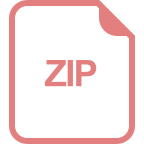
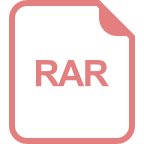
