MPU6050的合成加速度数据和角度数据UNITY小球运动的代码
时间: 2023-05-31 16:02:36 浏览: 57
以下是使用MPU6050合成加速度和角度数据,控制Unity小球运动的代码:
```c#
using UnityEngine;
using System.Collections;
using System.IO.Ports;
using System.Threading;
public class MPU6050Controller : MonoBehaviour {
public float speed = 10.0f; //小球运动速度
public string portName = "COM3"; //串口名称
public int baudRate = 9600; //串口波特率
public float angleOffsetX = 0.0f; //X轴角度偏移量
public float angleOffsetY = 0.0f; //Y轴角度偏移量
public float angleOffsetZ = 0.0f; //Z轴角度偏移量
private SerialPort serialPort;
private Thread readThread;
private float angleX = 0.0f;
private float angleY = 0.0f;
private float angleZ = 0.0f;
void Start () {
serialPort = new SerialPort(portName, baudRate);
serialPort.ReadTimeout = 50;
serialPort.Open();
readThread = new Thread(ReadData);
readThread.Start();
}
void Update () {
float moveHorizontal = Input.GetAxis("Horizontal");
float moveVertical = Input.GetAxis("Vertical");
transform.Translate(new Vector3(moveHorizontal, 0.0f, moveVertical) * speed * Time.deltaTime);
transform.Rotate(new Vector3(-angleY, angleX, 0.0f));
}
void OnDestroy()
{
if (serialPort != null && serialPort.IsOpen)
{
serialPort.Close();
}
if (readThread != null && readThread.IsAlive)
{
readThread.Abort();
}
}
private void ReadData()
{
while (true)
{
try
{
string data = serialPort.ReadLine();
string[] values = data.Split(',');
if (values.Length == 6)
{
float accelX = float.Parse(values[0]);
float accelY = float.Parse(values[1]);
float accelZ = float.Parse(values[2]);
float gyroX = float.Parse(values[3]);
float gyroY = float.Parse(values[4]);
float gyroZ = float.Parse(values[5]);
angleX = Mathf.Atan2(accelY, Mathf.Sqrt(Mathf.Pow(accelX, 2) + Mathf.Pow(accelZ, 2))) * Mathf.Rad2Deg - angleOffsetX;
angleY = Mathf.Atan2(-accelX, Mathf.Sqrt(Mathf.Pow(accelY, 2) + Mathf.Pow(accelZ, 2))) * Mathf.Rad2Deg - angleOffsetY;
angleZ += gyroZ * Time.deltaTime - angleOffsetZ;
}
}
catch (System.Exception e)
{
Debug.Log(e.Message);
}
}
}
}
```
该代码首先会在Start()函数中初始化串口并开启读取数据的线程。在Update()函数中,根据玩家的输入控制小球的运动,并根据MPU6050传输的角度数据控制小球的旋转。
在ReadData()函数中,从串口读取MPU6050传输的加速度和角速度数据,并计算出合成的角度数据。其中,加速度数据的计算使用了三维空间中的向量计算方法,角速度数据则直接累加。
最后,在OnDestroy()函数中关闭串口和线程,释放资源。
需要注意的是,该代码需要使用Unity的SerialPort插件来实现串口的读取。在使用前需要先将SerialPort.cs文件导入到Unity工程中,并将该文件挂载到脚本所在的游戏对象上。同时,需要将MPU6050传输的数据格式设置为“加速度X,加速度Y,加速度Z,角速度X,角速度Y,角速度Z”的格式。
相关推荐
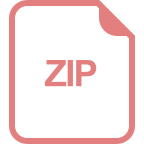
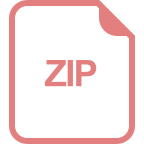














