请帮我写一个卷积神经网络的代码
时间: 2023-10-24 13:07:59 浏览: 42
当然可以帮您写一个卷积神经网络的代码,以下是一个简单的例子:
```python
import tensorflow as tf
# 设置超参数
learning_rate = 0.001
training_epochs = 10
batch_size = 100
display_step = 1
# 输入数据大小
n_input = 784 # MNIST 数据集的像素维度
n_classes = 10 # MNIST 数据集的类别数
# 定义输入占位符
x = tf.placeholder(tf.float32, [None, n_input])
y = tf.placeholder(tf.float32, [None, n_classes])
# 定义卷积神经网络结构
def conv_net(x, weights, biases):
# 将输入转换为卷积层可接受的形式
x = tf.reshape(x, shape=[-1, 28, 28, 1])
# 第一层卷积层,5x5 的窗口大小,32 个卷积核
conv1 = tf.nn.conv2d(x, weights['wc1'], strides=[1,1,1,1], padding='SAME')
conv1 = tf.nn.bias_add(conv1, biases['bc1'])
conv1 = tf.nn.relu(conv1)
# 第一层池化层,2x2 大小的窗口,步长为2
pool1 = tf.nn.max_pool(conv1, ksize=[1,2,2,1], strides=[1,2,2,1], padding='SAME')
# 第二层卷积层,5x5 的窗口大小,64 个卷积核
conv2 = tf.nn.conv2d(pool1, weights['wc2'], strides=[1,1,1,1], padding='SAME')
conv2 = tf.nn.bias_add(conv2, biases['bc2'])
conv2 = tf.nn.relu(conv2)
# 第二层池化层,2x2 大小的窗口,步长为2
pool2 = tf.nn.max_pool(conv2, ksize=[1,2,2,1], strides=[1,2,2,1], padding='SAME')
# 将卷积层的输出展开成一维向量
fc1 = tf.reshape(pool2, [-1, weights['wd1'].get_shape().as_list()[0]])
# 第一层全连接层,将展开后的卷积层输出变成512维的向量
fc1 = tf.add(tf.matmul(fc1, weights['wd1']), biases['bd1'])
fc1 = tf.nn.relu(fc1)
# 第二层全连接层,将第一层全连接层输出变成10维,即分类结果
out = tf.add(tf.matmul(fc1, weights['out']), biases['out'])
return out
# 构造卷积神经网络的各层参数
weights = {
'wc1': tf.Variable(tf.random_normal([5, 5, 1, 32])),
'wc2': tf.Variable(tf.random_normal([5, 5, 32, 64])),
'wd1': tf.Variable(tf.random_normal([7*7*64, 512])),
'out': tf.Variable(tf.random_normal([512, n_classes]))
}
biases = {
'bc1': tf.Variable(tf.random_normal([32])),
'bc2': tf.Variable(tf.random_normal([64])),
'bd1': tf.Variable(tf.random_normal([512])),
'out': tf.Variable(tf.random_normal([n_classes]))
}
# 构造卷积神经网络的输出,损失函数和优化器
logits = conv_net(x, weights, biases)
cost = tf.reduce_mean(tf.nn.softmax_cross_entropy_with_logits(logits=logits, labels=y))
optimizer = tf.train.AdamOptimizer(learning_rate=learning_rate).minimize(cost)
# 初始化变量
init = tf.global_variables_initializer()
# 启动 TF 图
with tf.Session() as sess:
sess.run(init)
# 训练循环
for epoch in range(training_epochs):
avg_cost = 0.
total_batch = int(mnist.train.num_examples/batch_size)
# 遍历所有批次
for i in range(total_batch):
batch_x, batch_y = mnist.train.next_batch(batch_size)
# 运行优化器和损失函数
_, c = sess.run([optimizer, cost], feed_dict={x: batch_x, y: batch_y})
avg_cost += c / total_batch
# 显示训练过程
if epoch % display_step == 0:
print("Epoch:", '%04d' % (epoch+1), "cost=", "{:.9f}".format(avg_cost))
print("Optimization Finished!")
# 测试模型
correct_prediction = tf.equal(tf.argmax(logits, 1), tf.argmax(y, 1))
accuracy = tf.reduce_mean(tf.cast(correct_prediction, tf.float32))
print("Accuracy:", accuracy.eval({x: mnist.test.images[:256], y: mnist.test.labels[:256]}))
```
该卷积神经网络使用 TensorFlow 库,其中包含了两个卷积层和两个全连接层,用于对 MNIST 手写数字分类数据集进行分类。在训练之前,需要设置超参数、定义输入占位符和网络结构等。在训练过程中,通过 Adam 优化器和交叉熵损失函数来优化模型,并计算准确度。最后,将该卷积神经网络应用于测试集上,可以得到分类准确度的评估结果。
相关推荐
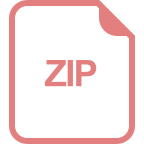














