ros通过c++实现话题订阅和udp数据转发
时间: 2023-05-28 15:05:46 浏览: 675
需要使用ROS中的C++ API来实现话题订阅和UDP数据转发。以下是一个简单的示例程序:
```cpp
#include <ros/ros.h>
#include <std_msgs/String.h>
#include <arpa/inet.h>
#include <sys/socket.h>
#define BUFLEN 512
#define PORT 8888
int main(int argc, char **argv) {
ros::init(argc, argv, "udp_forwarder");
ros::NodeHandle nh;
// Create a UDP socket
int udp_socket;
if ((udp_socket = socket(AF_INET, SOCK_DGRAM, IPPROTO_UDP)) == -1) {
ROS_ERROR("Failed to create UDP socket");
return 1;
}
// Configure the UDP socket
struct sockaddr_in udp_addr;
memset((char *)&udp_addr, 0, sizeof(udp_addr));
udp_addr.sin_family = AF_INET;
udp_addr.sin_port = htons(PORT);
udp_addr.sin_addr.s_addr = htonl(INADDR_ANY);
if (bind(udp_socket, (struct sockaddr *)&udp_addr, sizeof(udp_addr)) == -1) {
ROS_ERROR("Failed to bind UDP socket");
return 1;
}
// Subscribe to the ROS topic
ros::Subscriber sub = nh.subscribe("my_topic", 1000, [](const std_msgs::String::ConstPtr& msg) {
// Send the message over UDP
struct sockaddr_in dest_addr;
memset((char *)&dest_addr, 0, sizeof(dest_addr));
dest_addr.sin_family = AF_INET;
dest_addr.sin_port = htons(PORT);
dest_addr.sin_addr.s_addr = inet_addr("127.0.0.1");
if (sendto(udp_socket, msg->data.c_str(), msg->data.length(), 0, (struct sockaddr *)&dest_addr, sizeof(dest_addr)) == -1) {
ROS_ERROR("Failed to send UDP packet");
}
});
ros::spin();
return 0;
}
```
在这个示例中,我们使用了一个名为“my_topic”的ROS话题来接收数据。当有新的数据到达时,我们将其转发到UDP端口8888上的127.0.0.1。注意,我们在发送数据之前需要先创建一个UDP套接字并进行绑定。在程序退出时,我们使用ros::spin()来保持节点的活动状态,以确保我们能够接收到所有的ROS消息。
为了编译这个程序,您需要在CMakeLists.txt文件中添加以下内容:
```
add_executable(udp_forwarder src/udp_forwarder.cpp)
target_link_libraries(udp_forwarder ${catkin_LIBRARIES})
```
然后,您可以使用catkin_make命令来编译程序。当您运行这个程序时,它将开始订阅“my_topic”话题,并将所有接收到的消息转发到UDP端口8888上的127.0.0.1。您可以在需要接收这些数据的其他节点上运行一个UDP监听器来接收这些数据。
阅读全文
相关推荐
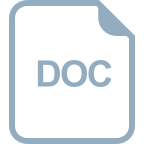
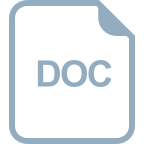
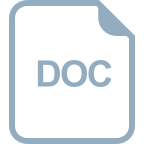
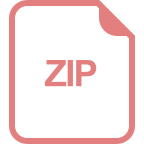
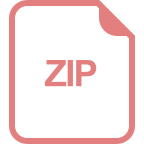
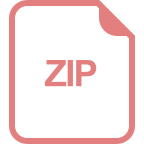
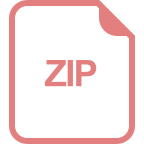
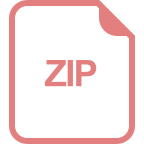
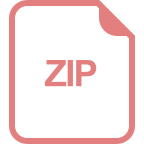
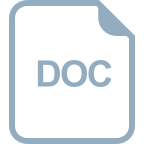
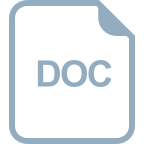
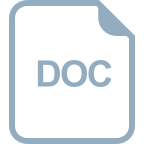


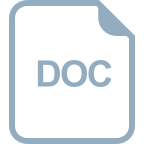
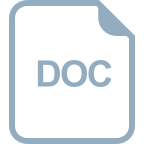