bounding_polygon = np.array([ [x[0], y[0], 21.000], [x[1], y[1], 21.000], [x[2], y[2], 21.000], [x[3], y[3], 21.000], ], dtype=np.float64) 这段代码如果x,y不是1到4,如何设置成0到i
时间: 2023-08-18 17:56:08 浏览: 127
如果 `x` 和 `y` 不是简单的连续整数序列,而是需要在 `[0, i]` 范围内生成对应的值,你可以使用一个 for 循环来设置 `bounding_polygon` 中的值。以下是一种可能的实现方式:
```python
import numpy as np
n = 4 # 数据点个数
bounding_polygon = np.zeros((n, 3), dtype=np.float64)
for i in range(n):
bounding_polygon[i] = [i, i, 21.0]
print(bounding_polygon)
```
在上述代码中,我们首先定义了数据点的个数 `n`。然后,我们创建了一个形状为 `(n, 3)` 的全零数组 `bounding_polygon`,用于存储数据点的坐标。接下来,通过使用一个 for 循环,我们将每个数据点的 x 和 y 坐标设置为当前循环变量 `i` 的值,而 z 坐标设置为固定值 21.0。
这样,循环结束后,`bounding_polygon` 中的值将变为 `[[0.0, 0.0, 21.0], [1.0, 1.0, 21.0], [2.0, 2.0, 21.0], [3.0, 3.0, 21.0]]`。请根据你的具体需求调整代码以适应不同的情况。
相关问题
bounding_polygon = np.array([ [x[0], y[0], 21.000], [x[1], y[1], 21.000], [x[2], y[2], 21.000], [x[3], y[3], 21.000], ], dtype=np.float64) 如何设置一个for循环,使这段代码中的[x[0], y[0], 21.000], [x[1], y[1], 21.000], [x[2], y[2], 21.000], [x[3], y[3], 21.000],变为0到i
如果你想使用一个 for 循环来设置 `bounding_polygon` 中的值,将其从 `[x[0], y[0], 21.000], [x[1], y[1], 21.000], [x[2], y[2], 21.000], [x[3], y[3], 21.000]` 变为 `[0, 0, i]`,可以按照以下方式进行:
```python
bounding_polygon = np.zeros((4, 3), dtype=np.float64)
for i in range(4):
bounding_polygon[i] = [0, 0, i]
```
上述代码中,我们首先创建一个形状为 (4, 3) 的全零数组 `bounding_polygon`,表示四个点的三维坐标。然后,通过使用一个 for 循环,将每个点的 x 和 y 坐标设置为 0,而 z 坐标使用循环变量 i 的值。
这样,循环结束后,`bounding_polygon` 中的值将变为 `[0, 0, 0], [0, 0, 1], [0, 0, 2], [0, 0, 3]`。
def get_bbox(height, width, points): polygons = points mask = np.zeros([height, width], dtype=np.uint8) mask = PIL.Image.fromarray(mask) xy = list(map(tuple, polygons)) PIL.ImageDraw.Draw(mask).polygon(xy=xy, outline=1, fill=1) mask = np.array(mask, dtype=bool) index = np.argwhere(mask == 1) rows = index[:, 0] clos = index[:, 1] left_top_r = np.min(rows) left_top_c = np.min(clos) right_bottom_r = np.max(rows) right_bottom_c = np.max(clos) return [ left_top_c, left_top_r, right_bottom_c - left_top_c, right_bottom_r - left_top_r ]
这段代码是一个函数,用于从图像中给定的多边形 points 中提取出包含该多边形的最小矩形 bounding box 的位置和大小信息。具体来说,输入参数包括图像的高度 `height`、宽度 `width`,以及多边形的顶点坐标 `points`。函数首先将多边形 points 转换为一个二值掩模图像,其中多边形内部的像素值为 1,其余像素值为 0。然后,通过 PIL 库中的 ImageDraw.Draw.polygon 函数,将多边形的轮廓绘制在掩模图像上,生成一个包含多边形的二值掩模。接着,函数将该二值掩模转换为一个布尔类型的 numpy 数组 `mask`。然后,函数根据 `mask` 中像素值为 1 的位置,计算出多边形的最小矩形 bounding box 的位置和大小信息,即左上角坐标 `(left_top_c, left_top_r)` 和宽高 `(right_bottom_c - left_top_c, right_bottom_r - left_top_r)`,并将其打包成一个列表返回。
阅读全文
相关推荐
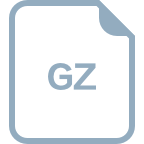
















