mediapipe holistic
时间: 2023-06-05 07:47:10 浏览: 182
Mediapipe Holistic是一种基于机器学习的姿势估计工具,可以同时检测人体的21个关键点,包括身体、手和面部。它可以用于许多应用程序,例如人体姿势分析、手势识别和面部表情分析。Mediapipe Holistic使用Google的Mediapipe框架,该框架提供了一种快速、灵活和可扩展的方法来构建视觉和媒体处理应用程序。
相关问题
mediapipe 通过holistic输出2d坐标
Mediapipe Holistic 模型可以输出人体关键点的 2D 像素坐标。具体来说,可以按照以下步骤进行:
1. 读取输入的图像,将图像转换为 Mediapipe 模型要求的格式(例如 RGB 图像,大小为 256x256 像素)。
2. 初始化 Mediapipe Holistic 模型,并将图像输入模型中进行姿态估计。
3. 解析模型输出,获取人体各个关键点的 2D 像素坐标。具体来说,可以通过以下代码获取:
```
# 解析头部关键点坐标
nose_x = result.pose_landmarks.landmark[mp_holistic.PoseLandmark.NOSE].x * image_width
nose_y = result.pose_landmarks.landmark[mp_holistic.PoseLandmark.NOSE].y * image_height
# 解析左手腕关键点坐标
left_wrist_x = result.left_hand_landmarks.landmark[mp_holistic.HandLandmark.WRIST].x * image_width
left_wrist_y = result.left_hand_landmarks.landmark[mp_holistic.HandLandmark.WRIST].y * image_height
# 解析右膝盖关键点坐标
right_knee_x = result.right_leg_landmarks.landmark[mp_holistic.LegLandmark.KNEE].x * image_width
right_knee_y = result.right_leg_landmarks.landmark[mp_holistic.LegLandmark.KNEE].y * image_height
```
其中,`result` 是模型输出的结果,`image_width` 和 `image_height` 分别是输入图像的宽度和高度。关键点的坐标值是在像素坐标系中的值,需要乘以图像的宽度或高度才能得到实际的坐标值。
4. 对于每个关键点,可以将其坐标值保存到一个列表或者数组中,以便后续使用。例如:
```
keypoints = [[nose_x, nose_y], [left_wrist_x, left_wrist_y], [right_knee_x, right_knee_y]]
```
这个列表中包含了图像中检测到的三个关键点的像素坐标。
需要注意的是,Mediapipe Holistic 模型输出的关键点数量比较多,共计 33 个,涵盖了人体的各个部位,具体可以参考 Mediapipe 官方文档中的说明。
import cv2 import mediapipe as mp mp_drawing = mp.solutions.drawing_utils mp_drawing_styles = mp.solutions.drawing_styles mp_holistic = mp.solutions.holistic # For webcam input: filepath='F:\\video000\\ce.mp4' cap = cv2.VideoCapture(filepath) with mp_holistic.Holistic( min_detection_confidence=0.5, min_tracking_confidence=0.5) as holistic: while cap.isOpened(): success, image = cap.read() if not success: print("Ignoring empty camera frame.") # If loading a video, use 'break' instead of 'continue'. break # To improve performance, optionally mark the image as not writeable to # pass by reference. image.flags.writeable = False image = cv2.cvtColor(image, cv2.COLOR_BGR2RGB) results = holistic.process(image) # Draw landmark annotation on the image. image.flags.writeable = True image = cv2.cvtColor(image, cv2.COLOR_RGB2BGR) mp_drawing.draw_landmarks( image, results.face_landmarks, mp_holistic.FACEMESH_CONTOURS, landmark_drawing_spec=None, connection_drawing_spec=mp_drawing_styles .get_default_face_mesh_contours_style()) mp_drawing.draw_landmarks( image, results.pose_landmarks, mp_holistic.POSE_CONNECTIONS, landmark_drawing_spec=mp_drawing_styles .get_default_pose_landmarks_style()) # Flip the image horizontally for a selfie-view display. cv2.imshow('MediaPipe Holistic', cv2.flip(image, 1)) if cv2.waitKey(5) & 0xFF == 27: break cap.release() 在这段代码中引入注意力机制,使人体步态特征更好的提取
在这段代码中,要引入注意力机制以提取人体步态特征,可以考虑在Holistic模型的输出结果中使用注意力机制进行加权融合,以强化对关键信息的关注和捕捉。具体操作如下:
1. 在Holistic模型的process()函数中,设置use_attention=True,以启用注意力机制。
2. 在Holistic模型的输出结果中,选择关键的特征点或特征向量,并使用注意力机制进行加权融合,以得到最终的人体步态特征表示。
3. 对特征表示进行进一步的处理和分类,以实现步态识别等应用。
下面是一个示例代码,用于在Holistic模型中引入注意力机制:
```
import cv2
import mediapipe as mp
mp_drawing = mp.solutions.drawing_utils
mp_drawing_styles = mp.solutions.drawing_styles
mp_holistic = mp.solutions.holistic
# For webcam input:
filepath = 'F:\\video000\\ce.mp4'
cap = cv2.VideoCapture(filepath)
with mp_holistic.Holistic(
min_detection_confidence=0.5,
min_tracking_confidence=0.5,
use_attention=True) as holistic:
while cap.isOpened():
success, image = cap.read()
if not success:
print("Ignoring empty camera frame.")
# If loading a video, use 'break' instead of 'continue'.
break
# To improve performance, optionally mark the image as not writeable to
# pass by reference.
image.flags.writeable = False
image = cv2.cvtColor(image, cv2.COLOR_BGR2RGB)
results = holistic.process(image)
# Extract the key feature points or vectors from the output results.
pose_landmarks = results.pose_landmarks.landmark
face_landmarks = results.face_landmarks.landmark
left_hand_landmarks = results.left_hand_landmarks.landmark
right_hand_landmarks = results.right_hand_landmarks.landmark
# Apply attention mechanism to the key feature points or vectors.
pose_attention = apply_attention(pose_landmarks)
face_attention = apply_attention(face_landmarks)
left_hand_attention = apply_attention(left_hand_landmarks)
right_hand_attention = apply_attention(right_hand_landmarks)
# Combine the attention-weighted feature vectors to form the final gait feature.
gait_feature = np.concatenate([pose_attention, face_attention, left_hand_attention, right_hand_attention])
# Further process and classify the gait feature to achieve gait recognition.
...
# Draw landmark annotation on the image.
image.flags.writeable = True
image = cv2.cvtColor(image, cv2.COLOR_RGB2BGR)
mp_drawing.draw_landmarks(
image,
results.face_landmarks,
mp_holistic.FACEMESH_CONTOURS,
landmark_drawing_spec=None,
connection_drawing_spec=mp_drawing_styles
.get_default_face_mesh_contours_style())
mp_drawing.draw_landmarks(
image,
results.pose_landmarks,
mp_holistic.POSE_CONNECTIONS,
landmark_drawing_spec=mp_drawing_styles
.get_default_pose_landmarks_style())
# Flip the image horizontally for a selfie-view display.
cv2.imshow('MediaPipe Holistic', cv2.flip(image, 1))
if cv2.waitKey(5) & 0xFF == 27:
break
cap.release()
```
其中,apply_attention()函数用于对输入的特征点或特征向量应用注意力机制,可以根据具体需求选择不同的注意力模型和参数。注意力机制的加权融合可以使用numpy库中的矩阵乘法或加法等运算实现。
相关推荐
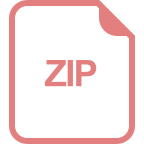
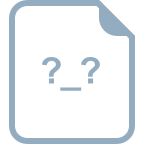
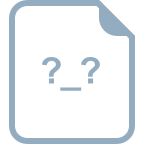












