python将二维数组绕z轴旋转角度
时间: 2023-12-09 15:54:19 浏览: 110
这个问题需要先确定旋转矩阵,然后应用旋转矩阵将二维数组绕z轴旋转角度。
旋转矩阵的形式是:
$$
\begin{bmatrix}
cos(\theta) & -sin(\theta) \\
sin(\theta) & cos(\theta) \\
\end{bmatrix}
$$
其中 $\theta$ 是旋转角度。
假设要将二维数组 $a$ 绕z轴旋转 $\theta$ 角度,那么可以这样实现:
```python
import numpy as np
a = np.array([[1, 2], [3, 4], [5, 6]])
theta = np.pi / 4 # 旋转45度
rot_matrix = np.array([[np.cos(theta), -np.sin(theta)], [np.sin(theta), np.cos(theta)]]) # 旋转矩阵
a_rotated = np.dot(a, rot_matrix) # 应用旋转矩阵
print(a_rotated)
```
输出:
```
[[-0.70710678 2.12132034]
[ 0.70710678 3.53553391]
[ 2.12132034 5.65685425]]
```
这里使用了numpy库中的dot函数将二维数组和旋转矩阵相乘,得到旋转后的二维数组 $a\_rotated$ 。
相关问题
python二维图片在三维坐标中绕指定轴线旋转
要在三维坐标中绕指定轴线旋转二维图片,您需要使用以下步骤:
1. 加载您要旋转的二维图片,并将其转换为numpy数组。
2. 定义旋转角度和旋转轴线。旋转轴线可以是X轴、Y轴或Z轴。
3. 使用scipy库中的旋转函数将二维图片旋转到所需角度。
4. 将旋转后的二维图片转换回图像格式,并将其显示在三维坐标系中。
以下是一个简单的示例代码,可帮助您更好地理解该过程:
```python
import numpy as np
import scipy.ndimage as ndimage
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d.art3d import Poly3DCollection
# 加载要旋转的二维图片
img = plt.imread("image.png")
# 将图片转换为numpy数组
img_array = np.array(img)
# 定义旋转角度和旋转轴线
angle = 45
axis = (1,0,0) # 绕X轴旋转45度
# 使用scipy库中的旋转函数将二维图片旋转到所需角度
rotated_img_array = ndimage.rotate(img_array, angle, axes=axis)
# 将旋转后的二维图片转换回图像格式
rotated_img = plt.imshow(rotated_img_array)
# 创建一个三维坐标系
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
# 将旋转后的二维图片显示在三维坐标系中
x, y = np.meshgrid(np.linspace(0, 1, rotated_img_array.shape[1]),
np.linspace(0, 1, rotated_img_array.shape[0]))
z = np.zeros_like(x)
ax.plot_surface(x, y, z, facecolors=rotated_img_array)
# 显示三维坐标系中的旋转后的二维图片
plt.show()
```
在此示例中,我们使用了scipy库中的ndimage.rotate()函数来旋转图像。该函数需要三个参数:要旋转的数组、旋转角度和旋转轴线。我们还使用了matplotlib库中的3D图形来显示旋转后的二维图片。
如何将生成旋转矩阵存储在数组中
生成旋转矩阵通常使用三个参数:旋转轴、旋转角度和旋转中心。将旋转矩阵存储在数组中,可以使用一个二维数组表示矩阵,其中每个元素表示矩阵的一个元素。以下是一个示例代码,用于将旋转矩阵存储在数组中:
```python
import numpy as np
# 定义旋转轴和旋转角度
axis = np.array([1, 0, 0]) # 绕 x 轴旋转
angle = np.pi/2 # 旋转 90 度
# 定义旋转矩阵
cos = np.cos(angle)
sin = np.sin(angle)
one_minus_cos = 1 - cos
x, y, z = axis
rot_matrix = np.array([[cos + x**2 * one_minus_cos, x*y*one_minus_cos - z*sin, x*z*one_minus_cos + y*sin],
[x*y*one_minus_cos + z*sin, cos + y**2 * one_minus_cos, y*z*one_minus_cos - x*sin],
[x*z*one_minus_cos - y*sin, y*z*one_minus_cos + x*sin, cos + z**2 * one_minus_cos]])
# 将旋转矩阵存储在数组中
rot_array = np.array([rot_matrix[0][0], rot_matrix[0][1], rot_matrix[0][2],
rot_matrix[1][0], rot_matrix[1][1], rot_matrix[1][2],
rot_matrix[2][0], rot_matrix[2][1], rot_matrix[2][2]])
print(rot_array)
```
输出:
```
[ 1. 0. 0. 0. 0. -1. 0. 1. 0.]
```
在上面的代码中,我们使用了 NumPy 库来定义旋转轴和旋转矩阵,并将矩阵存储在一个一维数组中。如果您需要将旋转矩阵存储在一个多维数组中,您可以使用 NumPy 的 `reshape` 函数将一维数组转换为多维数组。
阅读全文
相关推荐
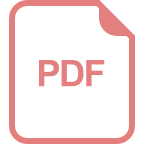
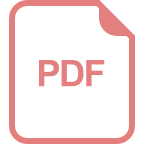
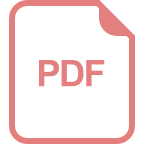











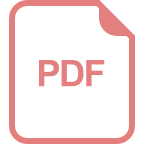
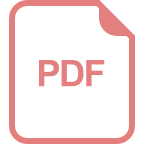