以二叉链表作为二叉树的存储结构。设计算法求二叉树中第一条最长的路径长度,并输出此路径上各结点的值。要求:(1)给出二叉链表中结点类型BTNode定义;(2)给出算法的基本设计思想;(3)根据设计思想,采用C或者C++语言描述算法。
时间: 2024-02-01 20:13:26 浏览: 34
(1)结点类型BTNode定义:
```
typedef struct BTNode {
int data; // 结点数据
struct BTNode *leftChild; // 左孩子指针
struct BTNode *rightChild; // 右孩子指针
} BTNode;
```
(2)算法的基本设计思想:
- 首先判断二叉树是否为空,如果为空则返回0。
- 如果不为空,则分别递归求出左子树和右子树的最大深度,记作leftDepth和rightDepth。
- 计算以根节点为起点的最长路径长度,即leftDepth + rightDepth,同时记录下此路径上的各结点值。
- 返回以根节点为起点的最长路径长度。
(3)C++实现:
```
int longestPath(BTNode *root, vector<int> &path) {
if (root == NULL) {
return 0;
}
int leftDepth = longestPath(root->leftChild, path);
int rightDepth = longestPath(root->rightChild, path);
int curDepth = leftDepth + rightDepth;
if (curDepth > path.size()) {
path.clear();
BTNode *p = root;
for (int i = 0; i < leftDepth; i++) {
path.push_back(p->data);
p = p->leftChild;
}
path.push_back(root->data);
p = root;
for (int i = 0; i < rightDepth; i++) {
path.push_back(p->data);
p = p->rightChild;
}
}
return max(leftDepth, rightDepth) + 1;
}
```
调用方式:
```
vector<int> path;
int length = longestPath(root, path);
cout << "最长路径长度为:" << length << endl;
cout << "该路径上的结点值为:";
for (int i = 0; i < path.size(); i++) {
cout << path[i] << " ";
}
cout << endl;
```
阅读全文
相关推荐
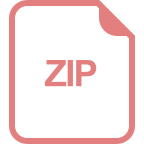
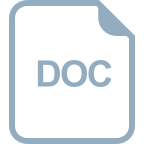












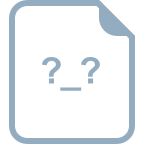