帮我简析以下代码: for det in pred: # 每张图片的检测 if len(det): # 将框从 img 大小重新缩放为 original_image 大小 # 将预测信息映射到原图(坐标框的位置信息映射回原图,填入det中前面的4个位置信息上) det[:, :4] = scale_coords(img.shape[2:], det[:, :4], original_image.shape).round() # ====写入结果==== #打印检测到的类别名称和数量 for *xyxy, conf, cls in reversed(det): c = int(cls) # integer class label = (self.names[c] if args.hide_conf else f'{self.names[c]} {conf:.2f}') plot_one_box(xyxy, original_image, label=label, color=colors(c, True), line_thickness=2) # Save results (image with detections) # cv2.imwrite(save_path, original_image) print(f'Done. ({time.time() - t0:.3f}s)') return original_image
时间: 2024-01-13 22:05:13 浏览: 31
这段代码是一个目标检测算法的输出结果可视化部分。给定预测的目标框的位置信息和类别信息,代码会将这些信息映射回原图,然后在原图上画出检测到的目标框,并在框上方打印出目标类别和置信度等信息。最后将可视化结果保存到指定路径下。具体细节如下:
1. 对于每张输入图片,循环遍历其对应的预测结果pred中的每个检测框det。
2. 如果检测结果det不为空,则将框从原始输入图片img大小重新缩放为原图original_image大小,并将预测信息映射到原图,填入det中前面的4个位置信息。
3. 对于每个检测框det中的坐标信息,反向循环遍历并依次取出目标框的左上和右下角坐标xyxy、目标置信度conf和目标类别cls等信息。
4. 将目标类别cls转换为整数形式,并根据所选参数hide_conf决定是否打印出目标类别和置信度等信息。
5. 调用plot_one_box函数在原图original_image上画出目标框,并在框上方打印出目标类别和置信度等信息。
6. 最后将可视化结果保存到指定路径下,并打印出处理时间。
相关问题
if len(det): # Rescale boxes from img_size to im0 size这个可以怎么改
如果您想更改这一行代码,可以使用以下方法修改:
```python
if len(det):
# Rescale boxes from img_size to original image size
det[:, :4] = scale_coords(img.shape[2:], det[:, :4], im0.shape).round()
```
在这段代码中,`scale_coords` 函数的作用是将检测框的坐标从输入图像的大小(`img_size`)缩放到原始图像的大小(`im0`),以便在原始图像上显示检测结果。
如果您想更改缩放的方式,可以修改 `scale_coords` 函数的实现。以下是 `scale_coords` 函数的示例代码:
```python
def scale_coords(img1_shape, coords, img0_shape):
# img1_shape: (height, width)
# coords: [[x1, y1, x2, y2, conf, cls], ...]
# img0_shape: (height, width)
# 计算缩放比例
gain = max(img1_shape) / max(img0_shape)
pad = (img1_shape[1] - img0_shape[1] * gain) / 2, (img1_shape[0] - img0_shape[0] * gain) / 2
# 对检测框坐标进行缩放和平移
coords[:, [0, 2]] -= pad[0]
coords[:, [1, 3]] -= pad[1]
coords[:, :4] /= gain
# 对检测框坐标进行裁剪
coords[:, :4] = clip_coords(coords[:, :4], img0_shape)
return coords
```
在这个示例代码中,`scale_coords` 函数首先计算缩放比例 `gain` 和平移量 `pad`,然后对检测框坐标进行缩放和平移操作。您可以根据您的需求修改这个函数的实现,以实现不同的缩放方式。
if len(det): # Rescale boxes from img_size to im0 size这个把注释掉的那行怎么加进去
如果您想将注释掉的那行代码添加回去,可以将以下代码:
```python
if len(det):
# Rescale boxes from img_size to original image size
det[:, :4] = scale_coords(img.shape[2:], det[:, :4], im0.shape).round()
```
改为以下代码:
```python
if len(det):
# Rescale boxes from img_size to original image size
det[:, :4] = scale_coords(img.shape[2:], det[:, :4], im0.shape).round()
# Annotate detections
for *xyxy, conf, cls in reversed(det):
c = int(cls) # integer class
label = f'{names[c]} {conf:.2f}'
plot_one_box(xyxy, im0, label=label, color=colors(c, True), line_thickness=3)
```
这样就可以将注释掉的那行代码添加回去,同时保留原有代码中的绘制检测框的操作。修改后的代码会将目标检测结果绘制在原始图像上,并显示在屏幕上。
相关推荐
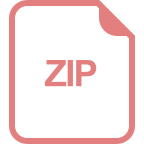
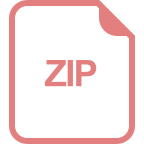
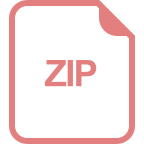












